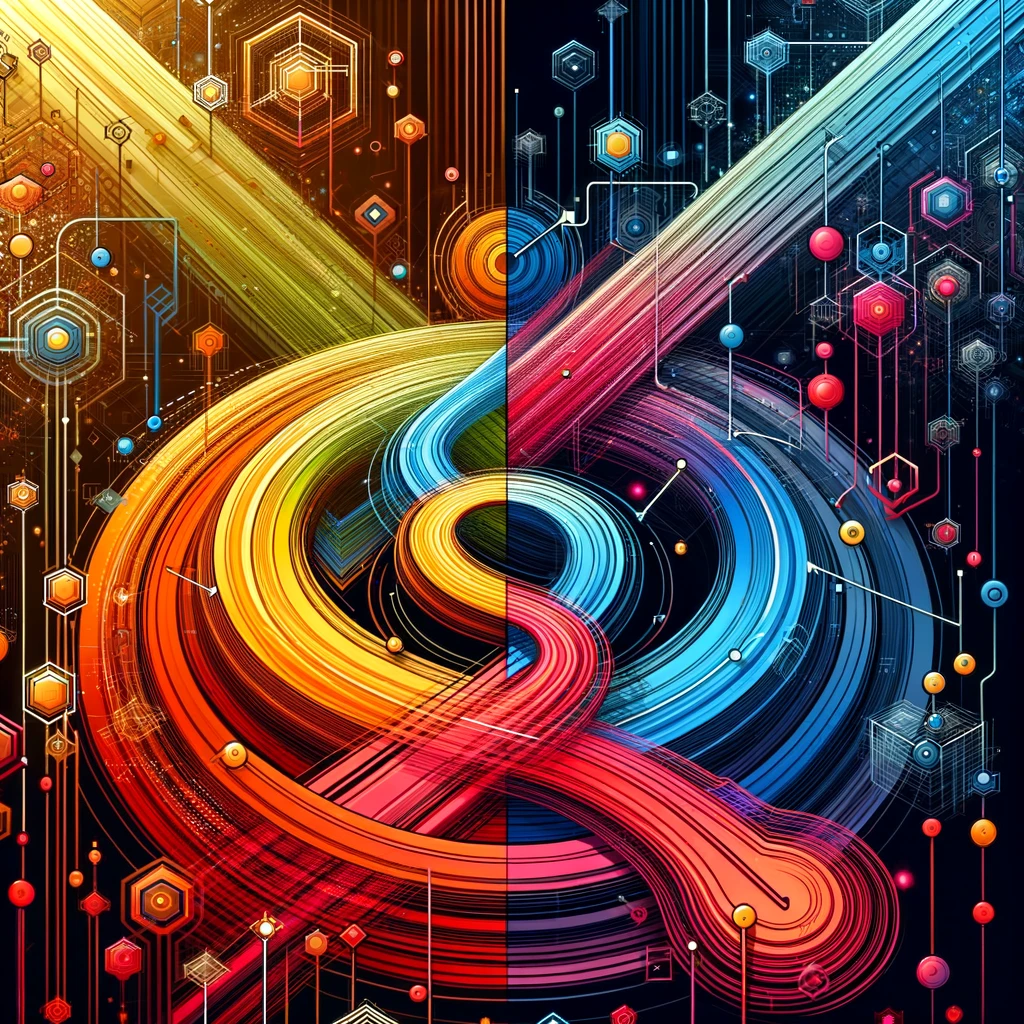
In software development, writing clean, maintainable, and efficient code is akin to an art form. Among the various metrics and methodologies developed to evaluate the quality of code, cyclomatic complexity stands out as a crucial gauge of a program’s complexity and its ease of testing and maintenance. This concept, introduced by Thomas J. McCabe in 1976, offers a quantitative measure of a program’s complexity, essentially reflecting the number of linearly independent paths through a program’s source code.
Understanding and managing cyclomatic complexity is vital for developers aiming to enhance the quality of their software. High complexity scores indicate code that is potentially difficult to understand, test, and maintain, while lower scores suggest simpler, more straightforward codebases. In this post, we’ll dive deep into what cyclomatic complexity is, why it matters, and how to effectively manage it in your projects.
What is Cyclomatic Complexity?
Cyclomatic complexity measures the number of linearly independent paths through a program’s source code. In simpler terms, it quantifies the complexity of a program based on the decision points it contains. A decision point could be an if statement, a loop, or anything that results in branching, such as case or switch statements.
The formula to calculate cyclomatic complexity (V(G)) for a given program is: [V(G) = E - N + 2P] Where:
- (E) represents the number of edges (transitions) in the flow graph.
- (N) stands for the number of nodes (functions, statements, or blocks) in the flow graph.
- (P) is the number of connected components (usually (P = 1) for a single program).
For most practical purposes, especially in simpler programs or functions, the cyclomatic complexity can be thought of as the count of decision points plus one. This metric does not only apply to entire applications but is incredibly useful for assessing the complexity of individual functions or methods within a larger codebase.
Why Does Cyclomatic Complexity Matter?
Cyclomatic complexity directly impacts several aspects of software development and maintenance:
- Testability: A higher cyclomatic complexity means more paths through the code, which in turn means more test cases are required to achieve thorough testing. Simpler, lower-complexity code is inherently easier to test.
- Maintainability: Complex code can be hard to understand and modify. Each additional path through the code adds to the cognitive load on developers trying to comprehend or change it.
- Reliability: Code with fewer paths is generally more predictable and, by extension, more reliable. With fewer potential execution paths, there’s less room for bugs to hide.
- Security: Code that is more testable, maintainable, and reliable is inherently more secure because it facilitates early detection and correction of vulnerabilities, supports easy updates and fixes, and it ensures consistent performance.
Managing Cyclomatic Complexity
The key to managing cyclomatic complexity lies in recognizing when your code is becoming too complex and taking steps to simplify it. Here are some strategies to achieve this:
Refactor Early and Often
Refactoring is your best tool for reducing complexity. Break down complex methods into smaller, more manageable ones. This not only reduces complexity but also improves code reusability and readability.
Example:
Consider a function with a high cyclomatic complexity due to multiple nested if-else statements. Refactoring this into multiple smaller functions can significantly reduce complexity.
Before Refactoring:
def complex_function(input):
if input > 0:
if input < 10:
return "Input is between 1 and 9"
else:
return "Input is 10 or more"
else:
if input == 0:
return "Input is zero"
else:
return "Input is negative"
After Refactoring:
def handle_positive_input(input):
if input < 10:
return "Input is between 1 and 9"
return "Input is 10 or more"
def handle_non_positive_input(input):
if input == 0:
return "Input is zero"
return "Input is negative"
def simplified_function(input):
if input > 0:
return handle_positive_input(input)
return handle_non_positive_input(input)
Leverage Polymorphism
In object-oriented programming, polymorphism can be used to handle decisions based on object type without resorting to explicit branching. This approach can significantly reduce cyclomatic complexity.
Example:
Instead of using a switch or if-else statements to perform type-specific operations, define a base class with a virtual method and override it in derived classes.
Before Using Polymorphism:
class AnimalHandler {
void handleAnimal(Animal animal) {
if (animal instanceof Dog) {
// Handle dog
} else if (animal instanceof Cat) {
// Handle cat
}
// Additional animal types
}
}
After Using Polymorphism:
abstract class Animal {
abstract void handle();
}
class Dog extends Animal {
@Override
void handle() {
// Handle dog
}
}
class Cat extends Animal {
@Override
void handle() {
// Handle cat
}
}
class AnimalHandler {
void handleAnimal(Animal animal) {
animal.handle();
}
}
Use Guard Clauses
Guard clauses are conditional statements at the beginning of a function that check for certain conditions and return early if they’re met. This strategy flattens the structure of your code, reducing nesting and complexity.
Example:
def process_data(data):
if not data:
return "No data"
# Process data
Simplify Conditions
Complex conditional logic can often be simplified through the use of boolean algebra or by extracting complicated conditions into well-named variables or functions.
Example:
Before Simplification:
if (user.age > 18 and user.age < 65 or user.has_special_permission) and not user.is_banned:
// Allow access
After Simplification:
is_adult = user.age > 18 and user.age < 65
can_access = is_adult or user.has_special_permission
if can_access and not user.is_banned:
// Allow access
Automation
Leveraging automation to detect overly complex code can significantly enhance the maintainability and readability of software projects. Tools like ESLint, a powerful static code analysis tool for identifying problematic patterns in JavaScript code, serve as an excellent example of how automation can aid in managing code complexity. By integrating ESLint into your development workflow, you can configure it to enforce specific rules that target complexity directly, such as the complexity
rule, which allows you to specify a maximum cyclomatic complexity threshold.
In your .eslintrc
file:
{
"rules": {
"complexity": ["error", 10]
}
}
In this example, "error"
means that any code exceeding the complexity threshold will cause ESLint to throw an error, and the number 10
is the maximum cyclomatic complexity allowed. If you prefer to only receive a warning rather than an error, you can replace "error"
with "warn"
.
Once set up, ESLint automatically scans your codebase for violations of this threshold, flagging functions or blocks of code that exceed the specified complexity limit. This immediate feedback enables developers to refactor complex code segments into simpler, more manageable pieces before they become entrenched in the codebase. Furthermore, incorporating ESLint into continuous integration (CI) pipelines ensures that new code submissions adhere to complexity standards.
In your GitHub workflow:
- name: Run ESLint
run: npx eslint .
This promotes a culture of clean coding practices and prevents the accumulation of technical debt over time. By automating the detection of overly complex code, teams can maintain high standards of code quality with minimal manual effort, making tools like ESLint indispensable in modern software development environments.
Conclusion
Cyclomatic complexity is more than just a metric; it’s a guide towards writing cleaner, more maintainable code. By understanding and managing this complexity, developers can improve the testability, readability, and overall quality of their codebases. The strategies discussed above are just a starting point. As you grow as a developer, you’ll find your own ways to keep complexity in check, leading to more robust and reliable software. Remember, the goal is not to eliminate complexity entirely but to ensure it does not become an obstacle to development and maintenance efforts.