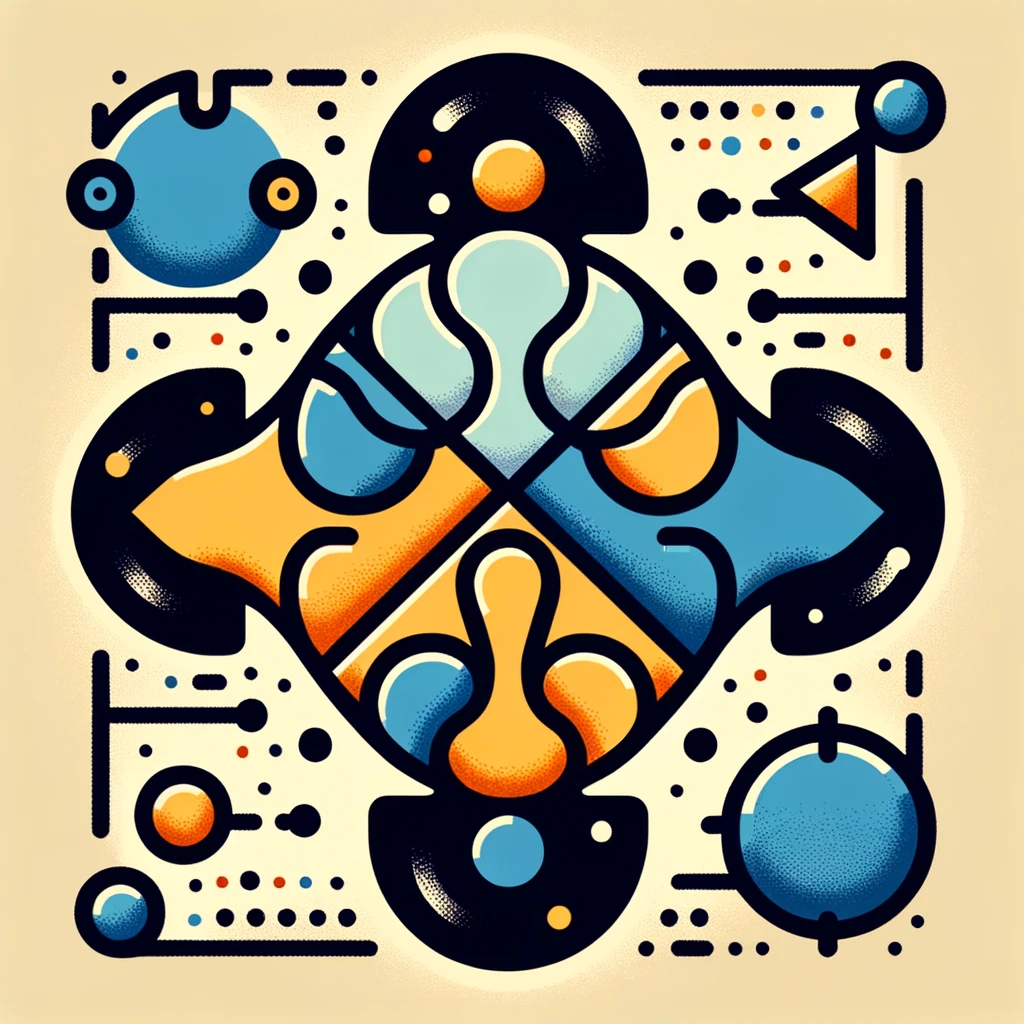
Generics are a cornerstone in the world of modern programming, allowing developers to write more flexible, reusable, and maintainable code. When you’re starting as a software engineer, the concept of generics might seem daunting, but it’s a powerful tool that can significantly enhance your coding efficiency and the quality of your applications. In this post, we’ll explore what generics are, why they matter, and how you can use them to write better code. We’ll also dive into practical examples and provide code snippets to demonstrate the concepts in action. For this post, I will be using examples written in Java, but generics are used throughout many (if not most) of the populate programming languages, and most of the same principles apply.
What Are Generics?
Generics enable algorithms, classes, interfaces, and methods to operate on objects of various types while providing compile-time type safety. The concept allows you to write a class or method that can work with any data type, not just one. This flexibility means you can reuse the same code for multiple data types, making your code more modular and reducing redundancy.
Why Use Generics?
Generics offer several benefits, including:
- Type Safety: Generics enforce type checks at compile time, preventing common pitfalls like ClassCastException at runtime. This means you catch errors sooner, during the development process.
- Code Reusability: Write a method or class once, and you can use it with different types, reducing code duplication.
- Performance: Generics have no runtime overhead; they use type erasure to ensure that no extra memory is used for parametrized types, making your code as fast as it is without generics.
Generics in Action: A Practical Example
To understand generics better, let’s consider a simple example without generics first. Imagine you want to create a method that returns the largest object from a list of objects. Without generics, your code might look something like this:
public static Object findLargest(List objects) {
if (objects == null || objects.isEmpty()) {
throw new IllegalArgumentException("List must not be null or empty");
}
Object largest = objects.get(0);
for (Object obj : objects) {
if (((Comparable) obj).compareTo(largest) > 0) {
largest = obj;
}
}
return largest;
}
This code works, but it’s not type-safe. You have to cast the objects to Comparable
, and if the list contains elements that are not comparable, it will throw a runtime exception.
Now, let’s refactor this code using generics:
public static <T extends Comparable<T>> T findLargest(List<T> objects) {
T largest = objects.get(0);
for (T obj : objects) {
if (obj.compareTo(largest) > 0) {
largest = obj;
}
}
return largest;
}
With generics, the method is now type-safe and more readable. The compiler ensures that the method is called with a list of objects that implement the Comparable
interface, eliminating the risk of runtime exceptions related to incorrect types.
Getting Started with Generics
To start using generics, here are some key concepts and syntaxes you should be familiar with:
- Generic Types: These are classes and interfaces that are parameterized over types, e.g.,
List<E>
, whereE
represents the type of elements in the list. - Type Parameter Naming Conventions: By convention, type parameter names are single, uppercase letters. The most commonly used type parameter names are:
E
- Element (used extensively by the Java Collections Framework)K
- KeyN
- NumberT
- Type
Generics and Collections
The Java Collections Framework is a prime example of generics in action. By using generics, collections can hold any type of object, and you can specify the type of objects they should contain, making the code safer and cleaner. For instance, List<String>
clearly indicates a list that contains strings, ensuring type safety without the need for explicit type casting or the risk of ClassCastException
.
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
// names.add(123); // Compile-time error
String first = names.get(0); // No casting needed
Generics in Custom Data Structures
When building your own data structures, generics are invaluable. They allow your data structures to be more flexible and applicable to a wider range of scenarios. For example, a generic Tree data structure can hold any type of data:
public class TreeNode<T> {
private T data;
private TreeNode<T> left;
private TreeNode<T> right;
public TreeNode(T data) {
this.data = data;
}
// getters and setters
}
This TreeNode
class can be used to construct trees of integers, strings, or any other objects, with the guarantee of type safety throughout.
Generics with Interfaces
Generics also shine when used with interfaces, allowing for flexible implementations. For example, a generic Repository
interface can define operations for various entity types:
public interface Repository<T> {
void add(T item);
T find(int id);
}
Implementations of this interface can specify the type of objects they work with, making the code modular and reusable across different parts of an application.
Wildcards and Generics
Wildcards (?
) add flexibility to generic code, especially when you’re dealing with complex method signatures. They allow a method to be parameterized with different types while maintaining type safety. For example, a method that processes a list of any subclass of Number
:
public void processNumbers(List<? extends Number> numbers) {
for (Number n : numbers) {
System.out.println(n);
}
}
This method can accept a List<Integer>
, List<Double>
, and so on, showcasing the power of wildcards in creating flexible and reusable code.
Best Practices Revisited
As you delve deeper into generics, here are a few more best practices to consider:
- Document Generics Well: Given their complexity and power, documenting how and why generics are used in your codebase is crucial for maintainability.
- Leverage Generic Algorithms: Generics aren’t just for data structures. They can also be applied to algorithms, making them more adaptable and reusable.
- Consider Generics during Design: When designing your system, think about where generics could be applied to make components more flexible and reduce code duplication.
Embracing Generics for Advanced Programming
Generics are not just a feature to be used occasionally; they should be a fundamental part of your programming toolkit. As you grow more comfortable with generics, challenge yourself with more complex scenarios where generics can provide elegant solutions. Whether it’s implementing design patterns, utilizing functional interfaces, or designing APIs, generics can often be the key to writing cleaner, safer, and more reusable code.
Remember, mastering generics is a journey. Continue experimenting, learning, and applying generics in new ways. The more you use them, the more you’ll appreciate the depth and flexibility they bring to your programming capabilities. Happy coding, and let the power of generics lead you to write exceptionally robust and flexible code!