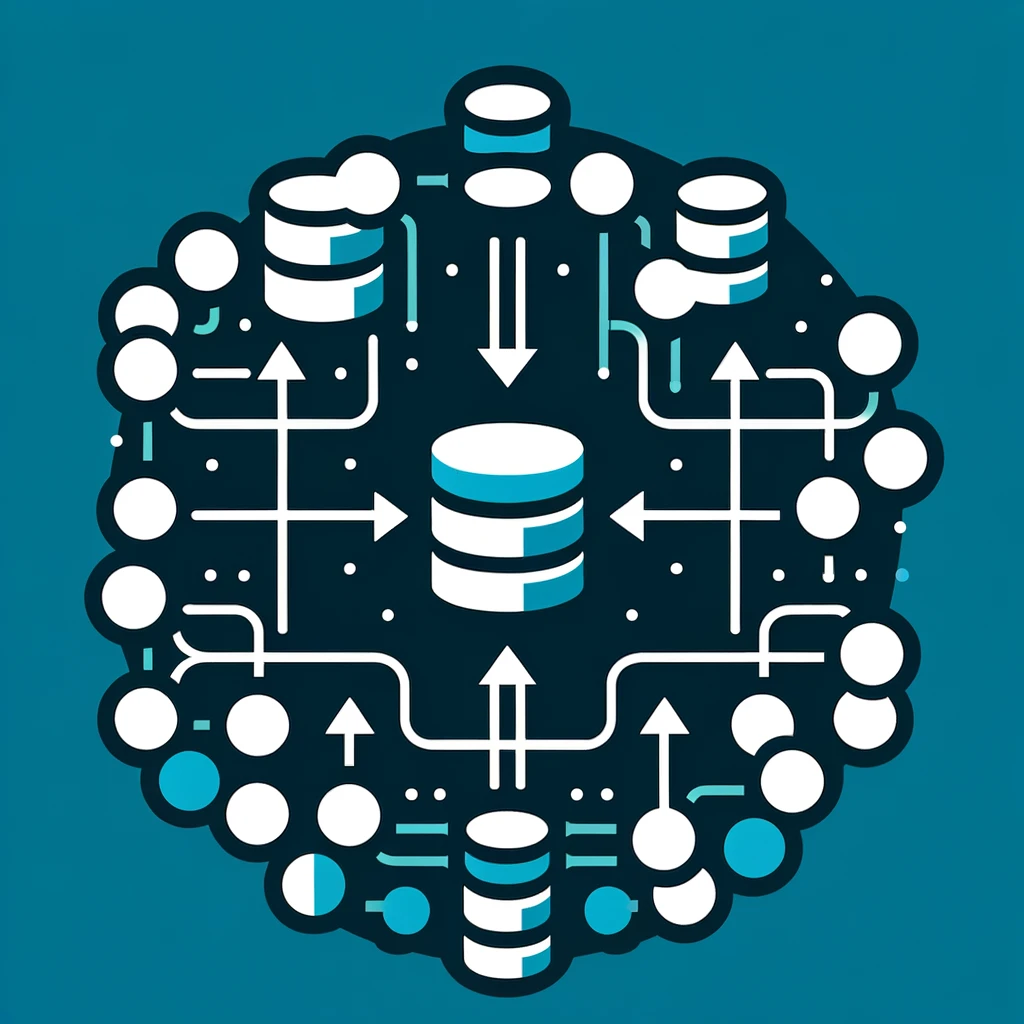
In the dynamic world of software development, enhancing performance while maintaining code clarity can seem like walking a tightrope. One technique that expertly balances this act is memoization. Memoization is a powerful optimization strategy used to speed up computer programs by storing the results of expensive function calls and returning the cached result when the same inputs occur again. It’s like having a knowledgeable assistant who, once you’ve asked a difficult question, remembers the answer for any future queries, saving you the time of figuring it out again.
Understanding Memoization Through Examples
Let’s delve into how memoization works with a classic example: computing Fibonacci numbers. The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. Here’s a simple recursive function to find the nth Fibonacci number:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
While elegant, this implementation suffers from severe inefficiency for large n
because it recalculates the same values repeatedly. This is where memoization shines.
By storing the results of each Fibonacci number as we compute them, we can avoid redundant calculations:
memo = {}
def fibonacci_memo(n):
if n in memo:
return memo[n]
if n <= 1:
return n
else:
memo[n] = fibonacci_memo(n-1) + fibonacci_memo(n-2)
return memo[n]
With this approach, each Fibonacci number is calculated once, dramatically improving performance.
Implementing Memoization in Python
Python provides a decorator that makes memoizing functions a breeze: functools.lru_cache
. This decorator caches the results of function calls, saving time when the function is called with the same arguments. Let’s apply it to our Fibonacci function:
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci_lru(n):
if n <= 1:
return n
else:
return fibonacci_lru(n-1) + fibonacci_lru(n-2)
Using lru_cache
is straightforward and doesn’t require manual management of the cache. The maxsize
parameter dictates the size of the cache, with None
meaning unlimited cache size. For most use cases, however, it’s wise to limit the cache size to prevent memory issues, unless you’re certain that the domain of inputs is small.
When to Use Memoization
Memoization is not a silver bullet for all performance issues. It’s most effective when:
- The function has a limited range of input values.
- Function calls are expensive (in terms of time or computational resources).
- The function is called repeatedly with the same arguments.
Common use cases include complex mathematical computations, recursive algorithms, and operations requiring heavy data processing.
Memoization in Modern Development
Beyond individual functions, memoization can be applied at the architecture level in software design. Caching strategies in web applications, for instance, use the same underlying principle to store responses to requests. Frameworks and libraries across programming languages offer built-in support for memoization, reflecting its integral role in efficient software development.
Best Practices and Pitfalls
While memoization is a powerful technique, it’s essential to use it judiciously:
- Memory Use: Be mindful of the cache size. Storing large amounts of data can lead to memory issues, especially with unlimited caches.
- Side Effects: Memoization is best used with pure functions (functions that have no side effects and return the same result for the same inputs).
- Debugging Complexity: Debugging memoized functions can be tricky, as it’s not immediately obvious whether a returned value came from the cache or was computed.
Comparing Memoization with Other Optimization Techniques
Here’s how memoization compares to other optimization techniques:
- Precomputation: Calculates and stores results ahead of time for known inputs as compared to memoization, which dynamically caches results as they’re computed.
- Lazy Evaluation: Defers computation until needed, reducing unnecessary work. Unlike memoization, which stores results, lazy evaluation postpones execution.
- Dynamic Programming (DP): DP solves problems by combining solutions of subproblems. Memoization is a DP technique, applicable in recursive scenarios to avoid recomputing.
- Code Optimization: Involves refining algorithms and data structures to improve efficiency, targeting overall algorithm performance rather than specific function calls.
Conclusion
Memoization is a cornerstone optimization technique in a developer’s toolkit, balancing performance with code maintainability. By understanding when and how to apply memoization, developers can significantly enhance the efficiency of their applications. Whether you’re tackling a known computational bottleneck or designing a system from the ground up, consider how memoization can contribute to a smoother, faster user experience.
In the landscape of software development, where efficiency and performance are paramount, embracing memoization is not just smart coding—it’s strategic problem-solving. Armed with the knowledge of how and when to implement this technique, you’re well on your way to writing code that’s not only fast and efficient but also elegant and easy to manage.