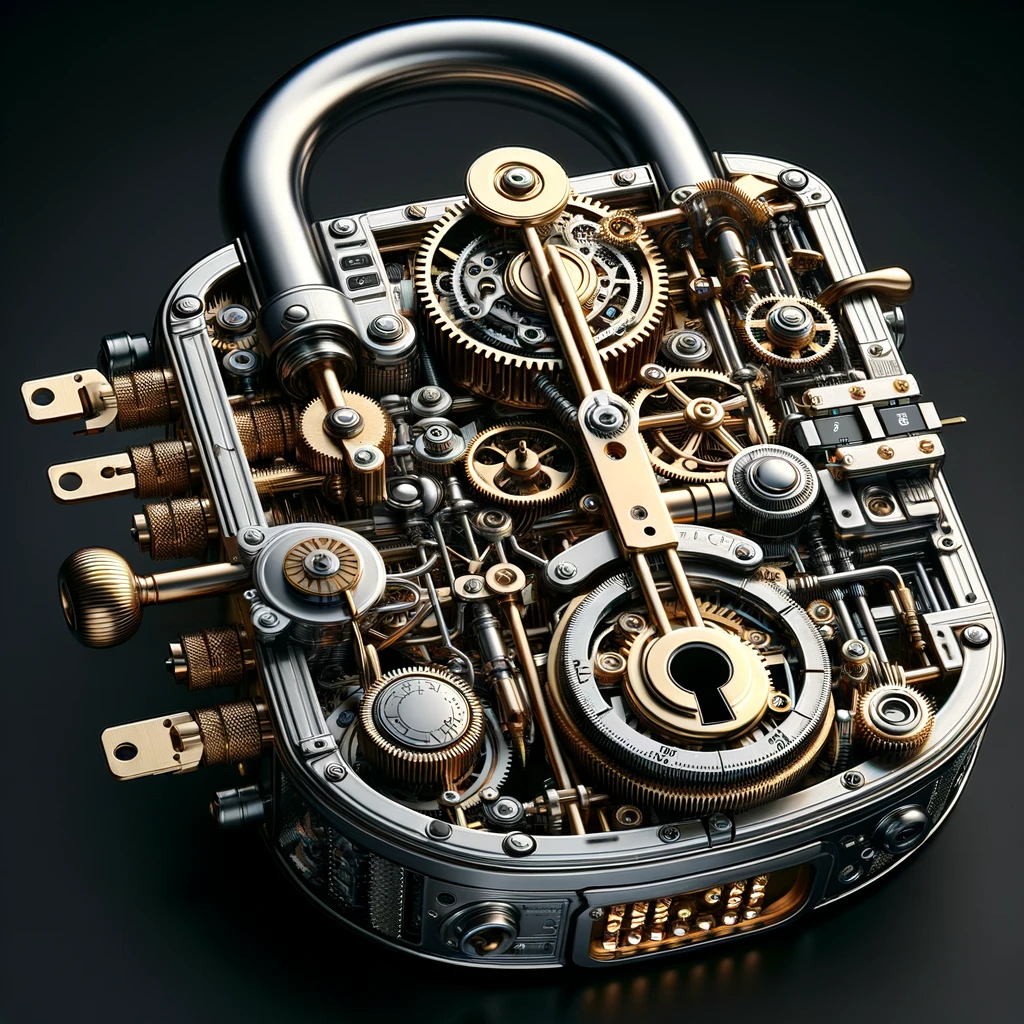
Hashing values is a cornerstone technique for protecting sensitive data. However, with rising computational power, traditional hashing methods have become vulnerable to brute-force attacks. This is where Key Derivation Functions (KDFs) like PBKDF2 (Password-Based Key Derivation Function 2) come into play. In this post, we’ll explore the benefits of using KDFs, particularly PBKDF2, for hashing values, highlighting how they enhance security in an increasingly digital world.
The Basics of Hashing and Its Vulnerabilities
Before diving into KDFs, let’s understand the basics of hashing. Hashing is a process of transforming input data (like a password) into a fixed-size string of characters, which is typically a hash code. The primary features of a good hash function include being fast to compute, deterministically producing the same hash for the same input, and being infeasible to reverse.
However, traditional hash functions like MD5 or SHA-1 have become less secure over time due to advancements in computing power and techniques like rainbow tables. These methods allow attackers to pre-compute hash values of common passwords and easily reverse-engineer them.
Enter Key Derivation Functions (KDFs)
KDFs are cryptographic algorithms that derive one or more secret keys from a secret value, such as a password. They are designed to be computationally intensive, making brute-force attacks much more difficult. One of the most widely used KDFs is PBKDF2.
PBKDF2: Strengthening Hashes with Salts and Iterations
PBKDF2 stands for Password-Based Key Derivation Function 2, a widely adopted standard defined in RFC 2898. It enhances the security of hashed passwords in two significant ways: by using salts and by applying many iterations of the hashing process.
Salting: PBKDF2 adds a random string, known as a salt, to the password before hashing it. This approach ensures that the same password will produce different hashes on each use, defeating rainbow table attacks.
Iteration: PBKDF2 applies the hash function multiple times (thousands or even millions of rounds) to the password and salt combination. This process, called stretching, makes the computation of the hash slow, significantly reducing the feasibility of brute-force attacks.
Practical Example: Implementing PBKDF2 in Python
Let’s look at a practical example of implementing PBKDF2 in Python, a commonly used language for back-end development.
import hashlib
import binascii
from os import urandom
def pbkdf2_hash(password, salt=None, iterations=100000):
if not salt:
salt = urandom(16)
dk = hashlib.pbkdf2_hmac('sha256', password.encode(), salt, iterations)
return binascii.hexlify(dk).decode(), binascii.hexlify(salt).decode()
password = "SecurePassword123"
hash, salt = pbkdf2_hash(password)
print(f"Hash: {hash}\nSalt: {salt}")
In this example, we use the hashlib
library to implement PBKDF2 with the SHA-256 hash function, a random salt, and 100,000 iterations. This code creates a unique, strong hash for any given password.
The Advantages of Using PBKDF2
Enhanced Security Against Brute-Force Attacks: By making the hash computation slow and resource-intensive, PBKDF2 significantly reduces the risk of brute-force attacks.
Mitigation of Rainbow Table Attacks: The use of unique salts for each password ensures that pre-computed hash tables (rainbow tables) are ineffective.
Flexibility and Customization: PBKDF2 allows for the adjustment of iterations based on the available computational power, balancing security and performance.
Widespread Adoption and Trust: As a standard that’s been rigorously tested and widely used, PBKDF2 is a trusted choice in many security-conscious applications.
Limitations and Considerations
While PBKDF2 is a robust option, it’s essential to consider its limitations:
- Resource Intensity: High iteration counts can strain system resources, particularly on servers handling many authentication requests.
- Evolving Threats: With advancements in technology like quantum computing, even PBKDF2 might need to evolve or be replaced by more advanced methods.
Concluding Thoughts
In summary, PBKDF2 represents a significant advancement in the security of hashing values, offering robust protection against some of the most common attack vectors. As we continue to navigate the digital landscape, understanding and utilizing such tools is paramount in safeguarding our data. By incorporating PBKDF2 into your security strategy, you can significantly enhance the safety and integrity of sensitive information in your applications.
For more information on PBKDF2 and its implementation, the RFC 2898 documentation provides comprehensive insights. Additionally, platforms like OpenSSL offer libraries and tools to integrate PBKDF2 into various applications.
Remember, security is a continually evolving field. Staying informed and adapting to new methods and threats is key to maintaining robust security protocols. Keep exploring, stay secure!