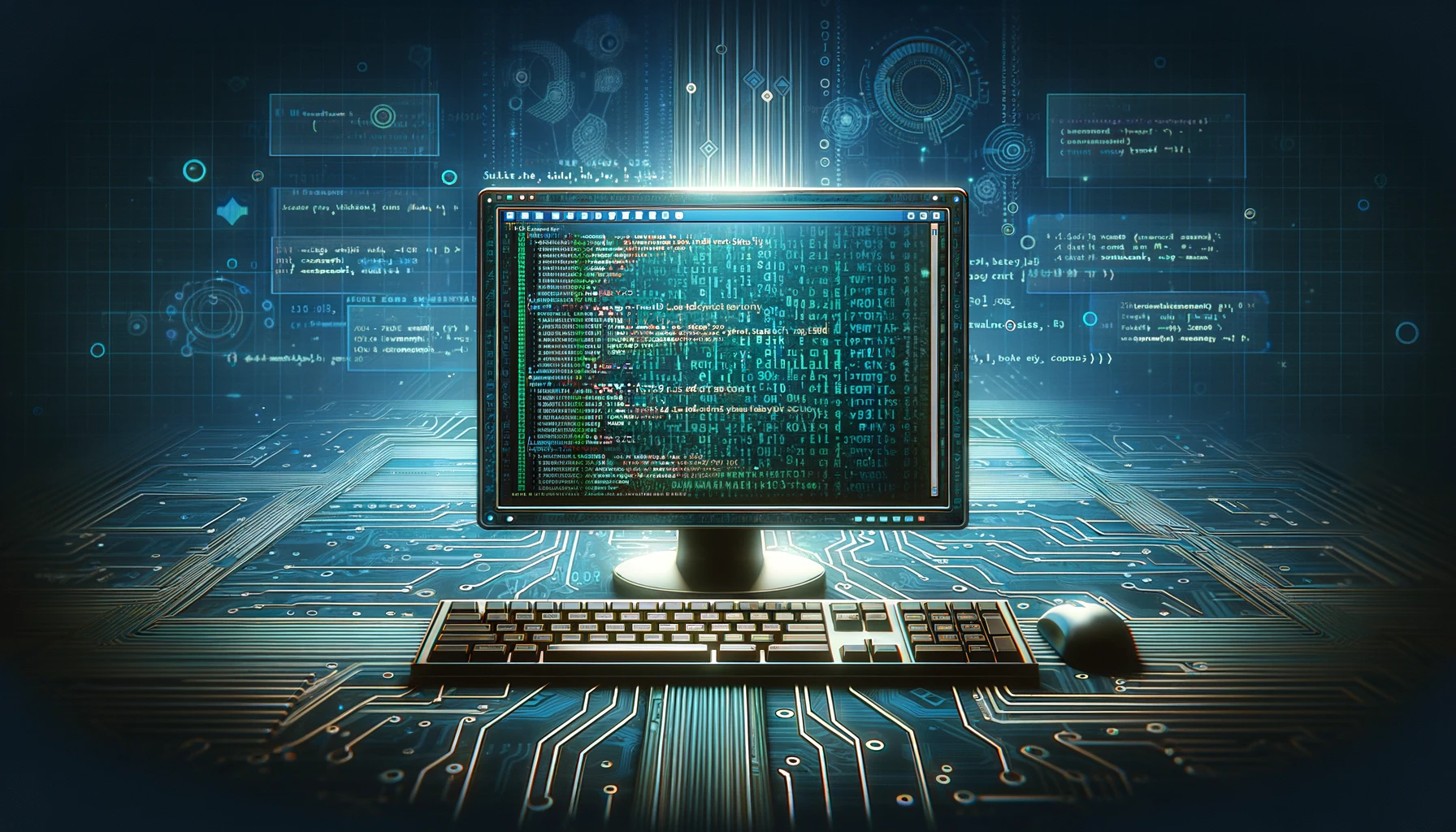
Shell scripting is a powerful tool in any developer’s arsenal, enabling the automation of complex tasks in a simple, effective manner. Two often-used, yet sometimes misunderstood, features in shell scripts are the set -x
and set -e
commands. While they serve different purposes—set -x
for debugging and set -e
for error handling—their correct usage can significantly improve the quality and reliability of your scripts. In this blog post, we’ll delve into the nuances of these commands and discuss some crucial security considerations.
Debugging with set -x
The set -x
command is a valuable debugging tool. It prints each command and its arguments to the standard error (stderr) before executing it. This feature provides a window into the script’s behavior, helping developers understand the flow and pinpoint where things might be going wrong.
Example Usage
Consider a simple script that creates a directory and moves files into it:
#!/bin/bash
mkdir my_directory
mv *.txt my_directory/
By adding set -x
at the beginning:
#!/bin/bash
set -x
mkdir my_directory
mv *.txt my_directory/
Running this script will output each command to stderr, showing exactly what the script is doing.
+ mkdir my_directory
+ mv some-file.txt my_directory/
When to Use set -x
set -x
is particularly useful in large scripts with many conditional statements or loops. When a script isn’t behaving as expected, set -x
can be a quick way to trace the execution path and the state of variables at different points in the script.
Error Handling with set -e
On the other hand, set -e
tells the shell to exit the script if any command returns a non-zero exit status (an indication of failure in Unix-like systems). This command is crucial for robust error handling.
Example Usage
In the absence of set -e
, a script will continue executing even after a command fails, which might lead to unexpected behaviors or corrupt data. For example:
#!/bin/bash
echo "Updating system..."
sudo apt-get update
echo "Installing important package..."
sudo apt-get install -y important-package
echo "Configuring system..."
sudo cp /etc/some/config /etc/some/other/config
echo "Script completed!"
If update
or install
fail, the script would continue executing subsequent commands, leading to the script succeeding when it didn’t actually do anything:
#!/bin/bash
set -e
echo "Updating system..."
sudo apt-get update
echo "Installing important package..."
sudo apt-get install -y important-package
echo "Configuring system..."
sudo cp /etc/some/config /etc/some/other/config
echo "Script completed!"
Now, if any of the commands fail, the script exits immediately, preventing any further potentially harmful actions.
Updating system...
script.sh: line 5: apt-get: command not found
When to Use set -e
set -e
is beneficial in scripts where errors need to be caught early and where each step depends on the successful completion of the previous one. It’s a simple way to make your scripts safer and more predictable.
Security Considerations with set -x
While set -x
is invaluable for debugging, it can inadvertently expose sensitive information. This is because it prints all commands and their arguments to stderr, including any that contain sensitive data like passwords or API keys.
Example of a Security Risk
#!/bin/bash
set -x
password="$1"
gh auth login --password "$password"
The above script will print the password in plain text to stderr, which is a significant security risk, especially if the logs are accessible to unauthorized users or are stored in insecure locations.
$ bash script.sh "my-secret-password"
+ password=my-secret-password
+ gh auth login --password my-secret-password
Mitigating the Risk
To mitigate this risk, you can temporarily turn off set -x
when executing commands that handle sensitive information:
#!/bin/bash
set -x
# Some non-sensitive commands
set +x
password="my_secret_password"
set -x
# More non-sensitive commands
By using set +x
, you disable the debugging output just before the sensitive command and re-enable it afterward with set -x
.
Conclusion
set -x
and set -e
are powerful tools in shell scripting, offering enhanced debugging and error handling capabilities. However, as with any tool, they must be used judiciously, especially in the context of security. Being mindful of how and where you use these commands, particularly set -x
in scenarios involving sensitive data, is crucial for maintaining robust and secure scripts. By integrating these practices into your scripting, you’ll achieve not only greater reliability but also uphold the crucial tenets of secure coding.