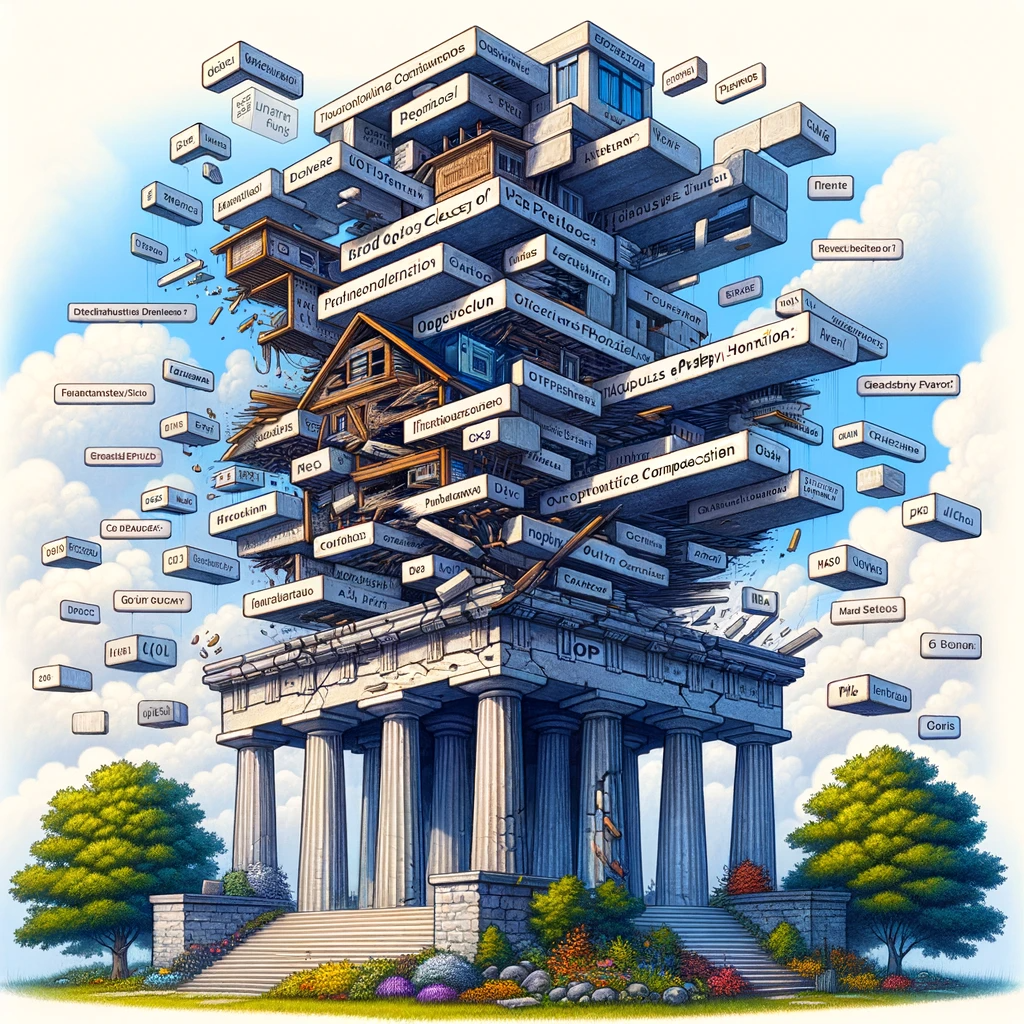
Introduction
In the realm of object-oriented programming (OOP), PHP’s introduction of traits in version 5.4 was greeted with enthusiasm by many developers. Traits, essentially a collection of methods that can be inserted into a class, were seen as a silver bullet for enhancing code reusability and reducing redundancy. However, this notion is a bit misguided. The overuse of traits often merely acts as a bandage over poor class design, concealing deeper issues in architectural planning and understanding of OOP principles. In this post, I will elucidate why relying heavily on traits is a symptom of bad class design, and how true OOP principles should guide PHP development.
Understanding Traits
Before delving into the critique, it’s essential to comprehend what traits are. In PHP, a trait is similar to a class, but intended to group functionality in a fine-grained and consistent way. They enable a sort of horizontal composition of behavior, as opposed to the vertical inheritance from classes. This sounds beneficial, but it’s exactly here that the problem begins.
Example
The following examples contrast the same class implementation using traits versus using dependency to accomplish the same functionality.
Traits:
trait LoggingTrait {
protected function log($message) { /* ... */ }
}
trait FileHandlingTrait {
protected function saveFile($content) { /* ... */ }
}
class ReportGenerator {
use LoggingTrait, FileHandlingTrait;
public function generateReport() {
$content = ...;
$this->log("Report generated, saving.");
$this->saveFile($content);
}
}
Dependency Injection:
class Logger {
public function log($message) { /* ... */ }
}
class FileHandler {
public function saveFile($content) { /* ... */ }
}
class ReportGenerator {
public function __construct(private Logger $logger, private FileHandler $fileHandler) {}
public function generateReport() {
$content = ...;
$this->logger->log("Report generated, saving.");
$this->fileHandler->saveFile($content);
}
}
The Crux of the Issue
1. Violation of Single Responsibility Principle (SRP)
The single responsibility principle, a core tenet of solid OOP design, states that a class should have only one reason to change. Traits, when misused, lead to classes having multiple responsibilities shoehorned into them via multiple traits. This not only violates SRP but also makes the code less readable and harder to maintain.
2. Encouraging Lazy Design
Traits can be misused to quickly add functionality to classes without properly considering the design. This “patchwork” approach leads to a lack of coherent structure in the codebase, making it difficult to understand the relationships and responsibilities of different classes.
3. Obscuring Class Dependencies
One of PHP’s strengths is its clarity and straightforward nature. However, when traits are overused, they can obscure the true nature of a class’s dependencies. This goes against the transparency and predictability that PHP is capable of delivering in well-designed applications.
4. Difficulty in Testing
Overusing traits complicates unit testing. Since traits can be included in any class, it becomes challenging to predict how a particular class will behave, especially when it uses multiple traits. This unpredictability is antithetical to robust, reliable software design.
The Superiority of Well-Thought-Out Class Design
PHP, as a language, provides ample features to create well-designed classes that adhere to OOP principles. Instead of relying on traits, developers should focus on:
1. Embracing Inheritance and Composition
Proper use of inheritance and composition is key to good class design. Inheritance should be used for ‘is-a’ relationships, while composition is for ‘has-a’ relationships. This clear distinction helps in building a more structured and understandable codebase.
2. Implementing Interfaces
Interfaces in PHP define contracts that classes can implement, ensuring a consistent API and promoting adherence to SRP. They are a far superior alternative to traits for defining and enforcing consistent behavior across classes.
3. Utilizing Dependency Injection
Dependency injection is a technique where a class is provided with its dependencies rather than creating them itself. This promotes a more modular and testable design, aligning with the best practices of software engineering.
4. Refactoring for Cohesion and Coupling
Code should be constantly refactored to improve cohesion (relatedness of features within a class) and reduce coupling (dependency on other classes). This ongoing process ensures the design remains clean, scalable, and maintainable.
Conclusion
In conclusion, while traits in PHP offer a mechanism for reusing code, their overuse is a clear indicator of poor class design. As PHP developers, our focus should be on leveraging the full spectrum of OOP features the language offers, adhering to principles like SRP, and striving for clarity, maintainability, and scalability in our designs. Traits have their place, but they are not a panacea for architectural challenges. Embracing the discipline of proper class design is not just beneficial; it’s essential for the longevity and success of PHP applications.