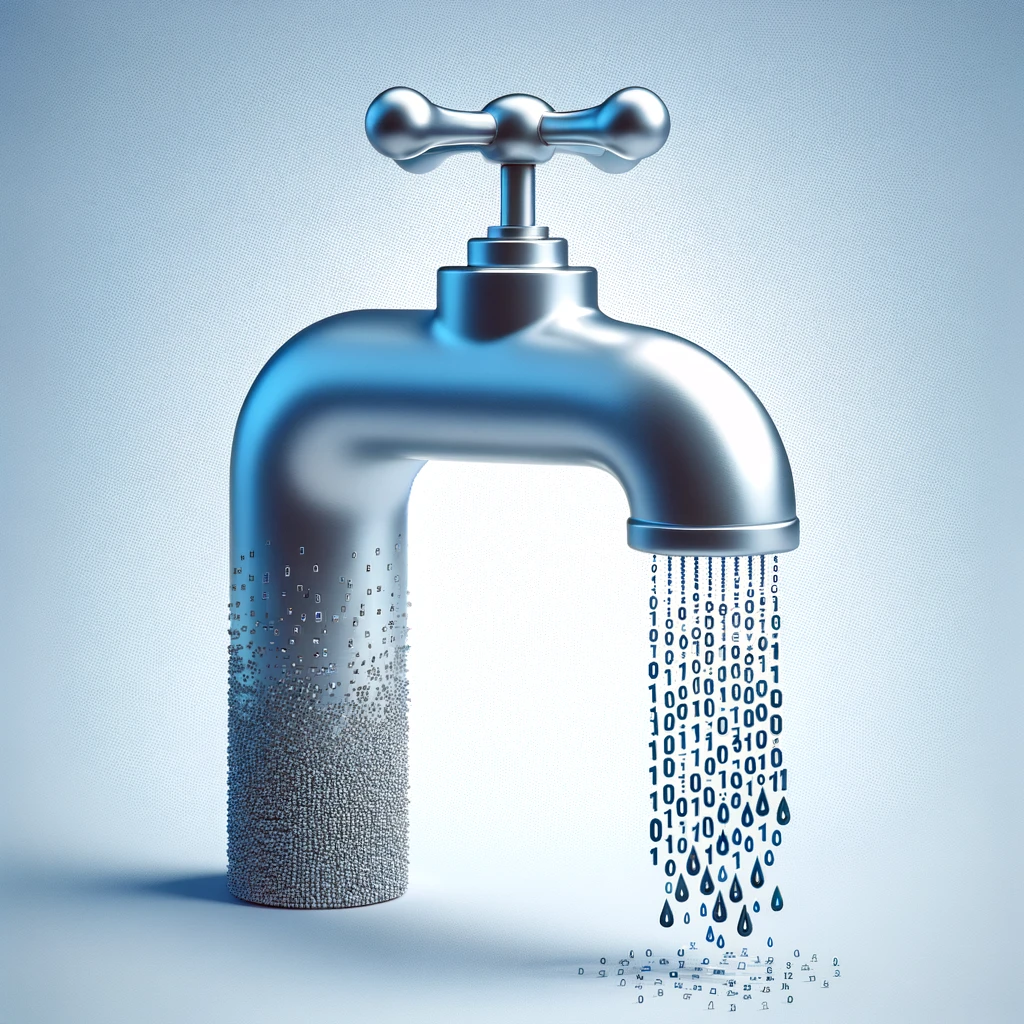
Memory leaks in C++ applications can be likened to silent assassins of system resources, gradually depleting them until the application or even the entire system grinds to a halt. Unlike more overt bugs that crash your application with a bang, memory leaks are insidious, often remaining undetected until they cause significant problems. This blog post delves into the strategies and tools that developers can wield to detect, diagnose, and obliterate these hidden threats, ensuring the robustness and efficiency of C++ applications.
Understanding Memory Leaks
A memory leak occurs when a program fails to release the memory it has allocated but no longer needs, leading to a gradual loss of available memory for other processes. In C++, where manual memory management is a common practice, the risk of leaks is notably higher compared to languages with automatic garbage collection.
Detecting memory leaks is more art than science, requiring a deep understanding of how your application manages memory and the tools at your disposal for monitoring and debugging.
Tools for the Trade
Several tools have been developed to assist in the detection of memory leaks in C++ applications. These range from simple, built-in language features to sophisticated third-party solutions. Let’s explore some of the most effective tools and techniques.
Valgrind
Valgrind is an instrumentation framework for building dynamic analysis tools. Among its various tools, Memcheck is particularly adept at detecting memory leaks. Running your application through Memcheck can help you identify where your program is leaking memory by providing detailed information about each leak, including the amount of memory leaked and the stack trace leading to the allocation.
To use Valgrind’s Memcheck, you would typically invoke it as follows:
valgrind --leak-check=full --show-leak-kinds=all --track-origins=yes --verbose --log-file=memcheck.log ./your_application
This command runs your application under Memcheck’s supervision, producing a detailed log file (memcheck.log
) containing information about memory leaks and other memory-related issues.
AddressSanitizer
AddressSanitizer (ASan) is a fast memory error detector integrated into compilers like GCC and Clang. It can detect various memory-related issues, including leaks, with minimal impact on execution time. ASan modifies your program at compile-time to include instrumentation code that checks each memory operation. When a leak is detected, ASan provides detailed information about the leak’s location and the stack trace.
To use ASan, compile your program with -fsanitize=address
flag:
g++ -fsanitize=address -g your_application.cpp -o your_application
Then, simply run your application as usual. ASan’s runtime will monitor memory usage and report leaks upon program exit.
LeakSanitizer
LeakSanitizer (LSan) is a part of AddressSanitizer specifically focused on detecting memory leaks. It’s available in GCC and Clang and can be used standalone (without the rest of ASan) if you’re only interested in leaks. Compilation and execution are similar to using ASan, but you specify -fsanitize=leak
instead:
g++ -fsanitize=leak -g your_application.cpp -o your_application
Visual Studio CRT Debug Heap Functions
For developers using Microsoft Visual Studio, the CRT (C Runtime Library) includes debug heap functions that can help detect memory leaks. By defining _CRTDBG_MAP_ALLOC
and including <crtdbg.h>
, these functions replace the normal memory allocation functions with debug versions that record information about each allocation.
At the program’s exit, you can automatically generate a report of all memory allocations that were not freed, indicating potential leaks. This feature is particularly useful for quick checks during development.
#define _CRTDBG_MAP_ALLOC
#include <stdlib.h>
#include <crtdbg.h>
int main() {
// Your code here
_CrtDumpMemoryLeaks(); // Check for memory leaks
return 0;
}
Best Practices for Avoiding Memory Leaks
While tools and techniques for detecting memory leaks are invaluable, the best strategy is to prevent them from occurring in the first place. Here are some best practices to follow:
- Use smart pointers (e.g.,
std::unique_ptr
,std::shared_ptr
) whenever possible, as they automatically manage memory for you, freeing it when the pointer goes out of scope. - Adopt RAII (Resource Acquisition Is Initialization) principles to ensure that resources are properly released when they’re no longer needed.
- Be cautious with ownership semantics, especially in complex data structures or when using raw pointers. Clearly define which part of your code is responsible for freeing memory.
- Regularly review and test your code for memory management issues, integrating tools like Valgrind or ASan into your development and testing workflows.
int main() {
// Manually allocate memory
Resource* ptr = new Resource();
// Use ptr...
// Manually deallocate memory.
delete ptr;
// Using std::unique_ptr to manage dynamically allocated memory. This
// pointer will be freed when it goes out of scope.
std::unique_ptr<Resource> ptr(new Resource());
// Allocating memory on the stack ensures that it gets cleaned up
// automatically. You also avoid having to interact with the heap with its
// own inefficiencies.
std::vector<int> data = {1, 2, 3, 4, 5};
return 0;
}
Conclusion
Memory leaks can be daunting, but with the right knowledge and tools, they are entirely manageable. By incorporating memory leak detection into your regular development and testing cycles, you can catch and fix leaks before they become serious issues. Remember, the goal is not only to find leaks but to understand why they occurred in the first place, allowing you to improve your coding practices and prevent future leaks.
Embrace the tools and techniques discussed in this post, but also commit to writing clean, maintainable code that minimizes the risk of leaks from the outset. With diligence and the right approach, you can master the art of detecting and preventing memory leaks in your C++ applications, leading to more robust, efficient software that stands the test of time.