About the author
Telmo Goncalves is a software engineer with over 13 years of software development experience and an expert in React. He’s currently Engineering Team Lead at Marley Spoon.
Check out more of his work on telmo.is and follow him on Twitter @telmo
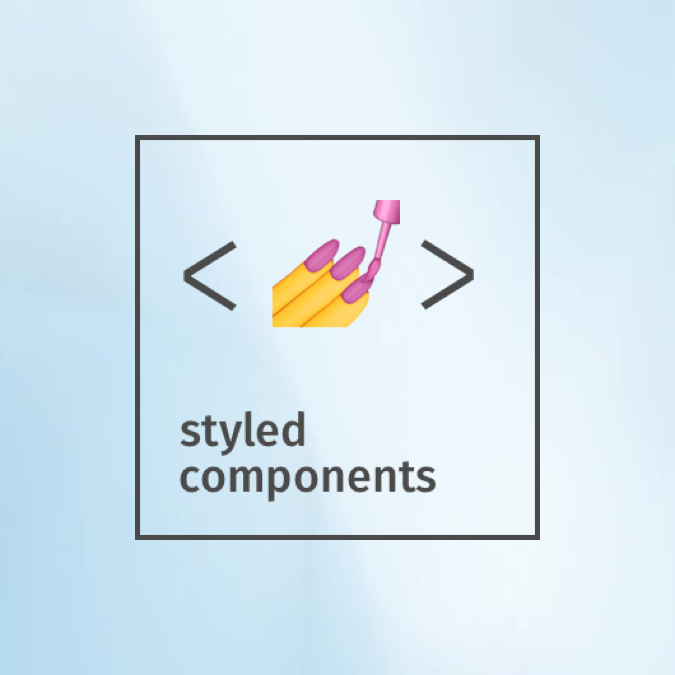
In the past I’ve used styled-components in pretty much all my projects. Having CSS code in the component can be helpful. After a while, as components grow, I’ve noticed that they can become a bit of a mess.
When dealing with small components it’s less an issue, the danger is when you start working with many elements.
Let’s take a look at an example:
export default function App() {
return (
<>
<h1>Hello!</h1>
<p>
Want to learn a neat way of using styled-components?
</p>
</>
)
}
We’ll convert both the h1
and p
elements. First we need to install styled-components
:
npm i styled-components
Now we’re ready to convert the elements:
import styled from 'styled-components';
const Title = styled.h1`
font-family: "Helvetica";
`
const Paragraph = styled.p`
font-family: "Helvetica";
font-size: 18px;
color: #666;
`
export default function App() {
return (
<>
<Title>Hello!</Title>
<Paragraph>
Want to learn a neat way of using styled-components?
</Paragraph>
</>
)
}
This is good! But can you image being 10 elements instead of just 2? This could get messy quickly.
The way I used to approach consolidating these is by creating a styles.js
file to hold the styles:
// styles.js
export const Title = styled.h1`
font-family: "Helvetica";
`;
export const Paragraph = styled.p`
font-family: "Helvetica";
font-size: 18px;
color: #666;
`;
// index.js
import { Title, Paragraph } from './styles'
Looks great! But again, imagine we have 10+ elements. The import
would look
something like this:
import {
Title,
Paragraph,
Header,
Button,
Secondary,
Image,
HeroCover,
SideBar,
// ... and so on!
} from './styles'
While it’s better than having all of the CSS in the component, we can avoid adding a long list of imports just taking up space. How can we do this? Import all!
import * as S from './styles'
In many cases import *
is avoided since it will import things you don’t need from large libraries
like Lodash. But in this case it’s fine because we’re limiting everything we need within styles.js
.
I like to use the S
namespace because, you know, Styles. You can name it whatever you want.
Doing so makes it easier to identify which components are styled:
<>
<S.Title>Hello!</S.Title>
<S.Paragraph>
Want to learn a neat way of using styled-components?
</S.Paragraph>
</>
Boom! Easy and helpful ✌️
Telmo regularly posts helpful React development tips and guides on Twitter. Be sure to follow him at @telmo