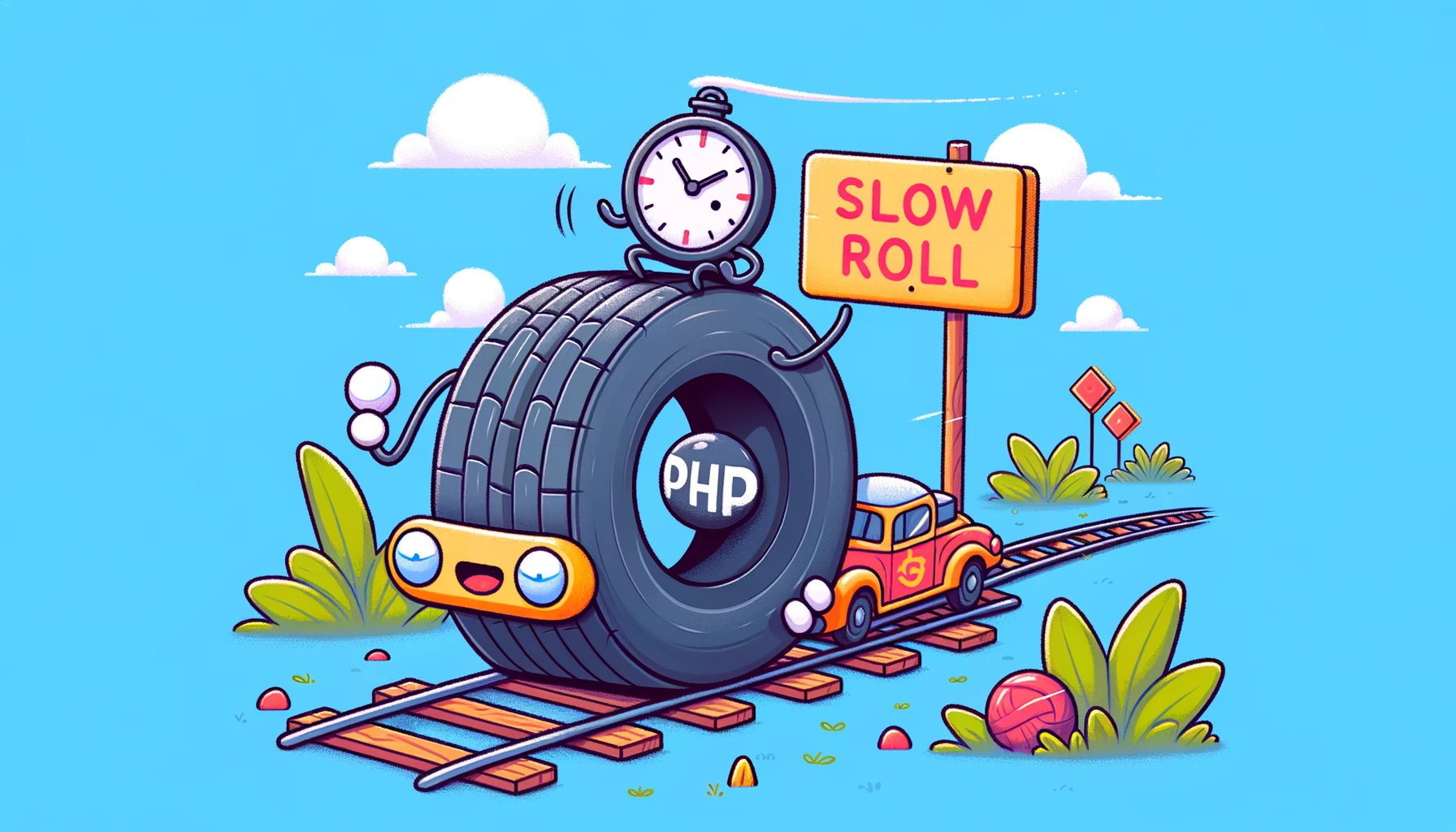
Refactoring, the process of restructuring existing computer code without changing its external behavior, is a crucial part of software development. It’s especially vital in the context of Laravel projects, where the framework’s elegance and robustness can be both a boon and a challenge during significant code changes. In this post, we’ll explore some practical tips for slow rolling refactors in Laravel projects. Our goal is to ensure a smooth transition while preventing breakages and incrementally implementing the refactor.
Understanding the Importance of Slow Rolling Refactors
In the bustling world of software development, refactoring isn’t just about cleaning up the code. It’s about enhancing the maintainability, performance, and scalability of your application. Laravel, with its rich set of features and expressive syntax, offers a fantastic platform for developing web applications. However, when it comes to refactoring, a rushed approach can lead to disastrous outcomes such as system outages, functionality breakages, and severe bugs.
Slow rolling refactors come into play here. This approach involves gradually implementing changes in a controlled and reversible manner. It allows teams to adapt to the evolving codebase progressively, minimizing disruptions and maintaining system stability.
Incremental Changes: Your Safety Net
The key to a successful slow rolling refactor is to break down the process into smaller, manageable changes. Each change should be self-contained, reversible, and, ideally, deployable on its own. This method reduces the risk of introducing significant errors and makes it easier to pinpoint the source of a problem if something goes wrong.
Example: Refactoring Database Queries
Consider a scenario where you need to refactor database queries in a Laravel application to improve performance. Instead of rewriting all queries at once, start with the most frequently used or the slowest ones. You can use Laravel’s query log or debugging tools like Laravel Telescope to identify these queries.
// Original query
$users = User::where('status', 'active')->get();
// Refactored query using eager loading to reduce the number of queries
$users = User::with('posts')->where('status', 'active')->get();
By refactoring one query at a time, you can monitor the performance impact of each change without overwhelming the system or the development team.
Automated Testing: Your Refactoring Companion
Automated tests are invaluable during a refactor. They provide a safety net, ensuring that changes don’t break existing functionality. Laravel’s built-in testing capabilities, like PHPUnit for unit and feature tests, and Dusk for browser tests, should be utilized to the fullest.
Before starting the refactor, ensure that your application has a comprehensive test suite. If it doesn’t, take the time to write tests for critical functionalities. This step might seem time-consuming, but it pays off by providing confidence during the refactoring process.
Feature Flags: Controlled Deployment
Feature flags are a powerful tool in slow rolling refactors. They allow you to enable or disable features in your application without deploying new code. This technique is particularly useful when you want to test new code paths in production without affecting all users.
In Laravel, you can implement feature flags using configuration files or packages like Laravel Feature Flags. Here’s a simple example:
// Using a feature flag to toggle between old and new code
if (config('features.new_refactored_feature')) {
// New refactored code
} else {
// Old code
}
This approach lets you gradually roll out changes to a subset of users, monitor the impact, and revert quickly if needed.
Embracing Continuous Integration (CI)
Continuous Integration plays a pivotal role in slow rolling refactors. With CI, every code change is automatically tested and integrated into the main codebase, ensuring that the refactor doesn’t introduce regressions.
Laravel integrates well with various CI tools like GitHub Actions, Travis CI, or GitLab CI. These tools can run your test suite on every commit, ensuring that each incremental change in your refactor maintains the integrity of the application.
Refactoring Techniques for Laravel
Service Extraction: If you have fat controllers or models, consider extracting business logic into services. This approach makes the code more readable, testable, and easier to refactor.
Middleware for Cross-Cutting Concerns: Utilize middleware for handling cross-cutting concerns like logging, authentication, or caching. This separation of concerns keeps your controllers clean and focused.
Leverage Laravel Collections: Collections are one of Laravel’s most powerful features. Refactor array manipulations to use collections, which are more readable and expressive.
Config and Language Files: Use config and language files for hard-coded values and strings. This practice makes your code more flexible and easier to update.
Dependency Injection: Use Laravel’s dependency injection features for better testability and decoupling of components.
Conclusion
Slow rolling refactors in Laravel require a well-thought-out strategy, focusing on incremental changes, thorough testing, and the use of modern tools and techniques. By breaking down the refactor into smaller steps, leveraging automated testing, utilizing feature flags, and embracing Continuous Integration, developers can ensure a smooth transition without disrupting the existing system. Additionally, applying specific Laravel-centric techniques like service extraction, middleware usage, leveraging collections, and dependency injection further streamline the process. The key is to approach refactoring as a journey of continuous improvement, enhancing the codebase while maintaining its integrity and performance. With these practices in place, your Laravel project can evolve gracefully, adapting to new requirements and technologies while minimizing risks and maintaining a high-quality code standard.