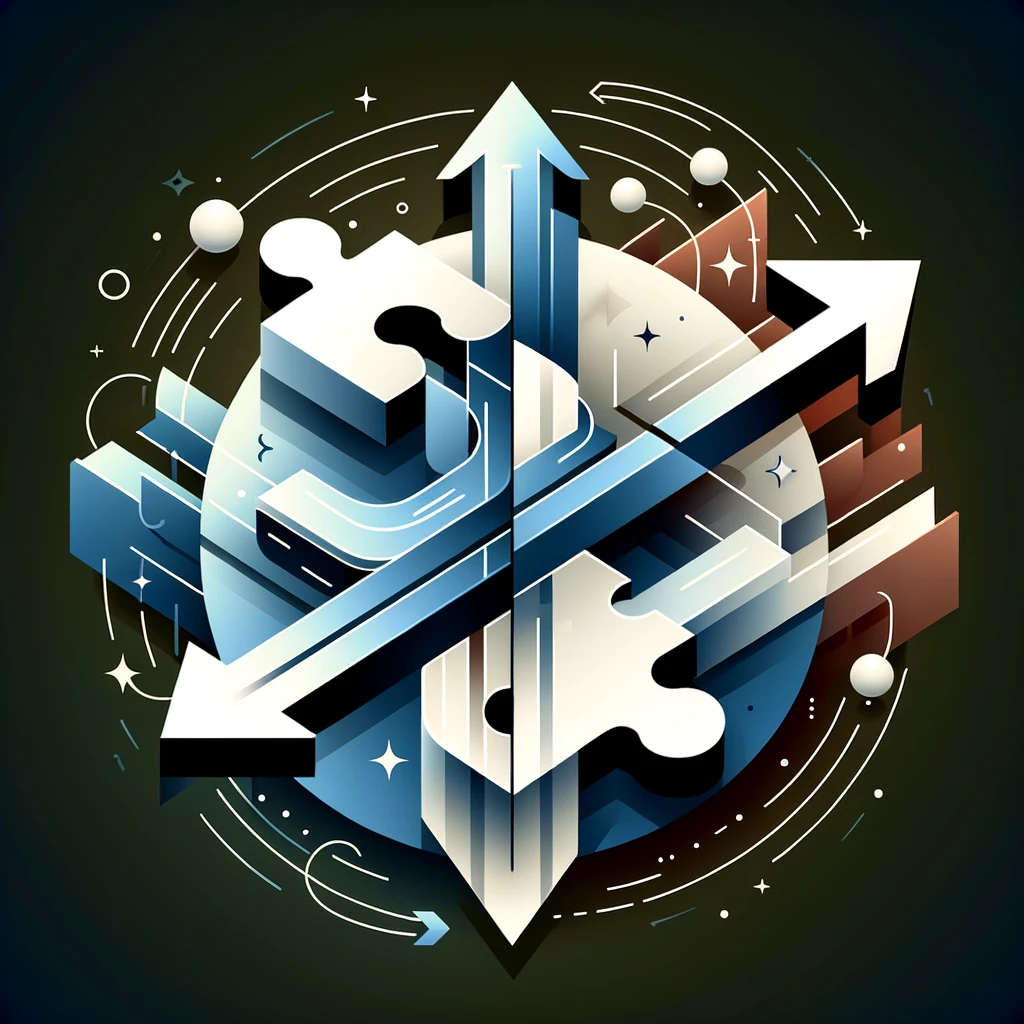
In the realm of software development, writing clean, efficient, and easily readable code is as crucial as the functionality it provides. TypeScript, a superset of JavaScript, brings strong typing and some syntactic sugar to the table, making our code more understandable and maintainable. Among the myriad of techniques to enhance code readability and efficiency, the use of logical operators (&&
, ||
) and the nullish coalescing operator (??
) stands out. These operators not only simplify conditional expressions but also make the intention behind the code clearer to fellow developers. In this blog post, we will delve into how replacing traditional code branches with these operators can lead to more streamlined and expressive code in TypeScript.
The Basics: Logical Operators
Logical operators are commonly used for boolean logic, but in TypeScript, they do more than just compare boolean values. They can be used to replace certain if-else structures, making the code more concise and readable.
Truthy vs Falsy vs Nullish
The concepts of “truthy” and “falsy” play a significant role in conditional logic and control flow. A “truthy” value is any value that translates to true
when evaluated in a boolean context, allowing for more flexible and concise conditional expressions. Conversely, a “falsy” value is one that evaluates to false
in the same contexts. The set of falsy values in TypeScript includes 0
, -0
, null
, undefined
, false
, NaN
, ''
(the empty string), and document.all
, a holdover for historical web compatibility reasons. Every other value is considered “truthy,” including all objects and arrays, even if they are empty. This distinction allows developers to write more succinct conditional checks and assignments, leveraging the fact that expressions like if (value)
or value || defaultValue
will behave predictably based on the truthiness or falsiness of value
.
The concept of “nullish” in TypeScript refers specifically to the values null
and undefined
, representing the absence of any value or an uninitialized state, respectively. This is where the nullish coalescing operator (??
) comes into play, offering a more precise alternative to the logical OR (||
) operator for default assignments. The ??
operator will only consider expressions to the left as “defaulted” if they are exactly null
or undefined
, ignoring other falsy values like 0
or ''
that might be valid in certain contexts. This distinction is particularly important for handling optional properties or variables that might legitimately hold falsy values while still needing a default when genuinely unset. By using ??
, TypeScript developers can ensure that only truly “nullish” values are replaced, making code logic clearer and preventing unintended behaviors associated with the broader category of falsy values.
Using &&
for Conditional Execution
The &&
operator can be used to execute code only if a certain condition is truthy. It evaluates expressions from left to right, and if all values are truthy, it returns the last value. If any value is falsy, it returns the first falsy value without evaluating the rest.
function logMessageIfTrue(isTrue: boolean, message: string) {
isTrue && console.log(message);
}
In this example, console.log(message)
is only executed if isTrue
is true
. This replaces the need for a more verbose if
statement.
Using ||
for Default Values
Similarly, the ||
operator is often used to provide a default value for variables that might be falsy. It returns the first truthy value it encounters or the last value if all are falsy.
function greet(name: string | undefined) {
const greetingName = name || 'Guest';
console.log(`Hello, ${greetingName}!`);
}
This function will greet the name provided or default to “Guest” if name
is undefined
or an empty string (and thus falsy).
Elevating the Game with Nullish Coalescing Operator (??
)
While the ||
operator is great for providing default values, it has a pitfall: it considers any falsy value (e.g., 0
, ''
, false
, null
, undefined
) as a trigger to return the second value. This is where the nullish coalescing operator (??
) shines, as it only considers null
or undefined
as triggers to return the second value.
function configureTimeout(timeout: number | undefined) {
const effectiveTimeout = timeout ?? 1000; // Defaults to 1000 only if timeout is null or undefined
setTimeout(doSomething, effectiveTimeout);
}
This is particularly useful when dealing with numeric values, where 0
might be a valid, intended value but would be treated as falsy by the ||
operator.
Practical Example: Streamlining Code with Logical and Nullish Coalescing Operators
Consider a scenario where we have a function that fetches user data. This data may or may not include a preferences object, and we want to apply defaults for any missing preferences.
interface User {
id: number;
name: string;
preferences?: {
theme: string;
notifications: boolean;
};
}
function fetchUserData(user: User) {
const theme = user.preferences?.theme ?? 'light';
const notificationsEnabled = user.preferences?.notifications ?? true;
console.log(`User ${user.name} prefers a ${theme} theme.`);
console.log(`Notifications enabled: ${notificationsEnabled}`);
}
In this example, the use of ?.
(optional chaining) combined with ??
allows us to succinctly apply default values for potentially undefined properties, making the code cleaner and more resilient to missing data.
Conclusion
The strategic use of logical operators (&&
, ||
) and the nullish coalescing operator (??
) in TypeScript can significantly enhance code readability and reduce line counts. By replacing traditional conditional branches with these operators, developers can write more expressive, concise, and maintainable code. Remember, the goal of using these techniques is not just to reduce the number of lines of code, but to make the code more intuitive and easier to understand for others (and your future self). Embrace these operators in your TypeScript projects and watch your codebase transform into a model of clarity.