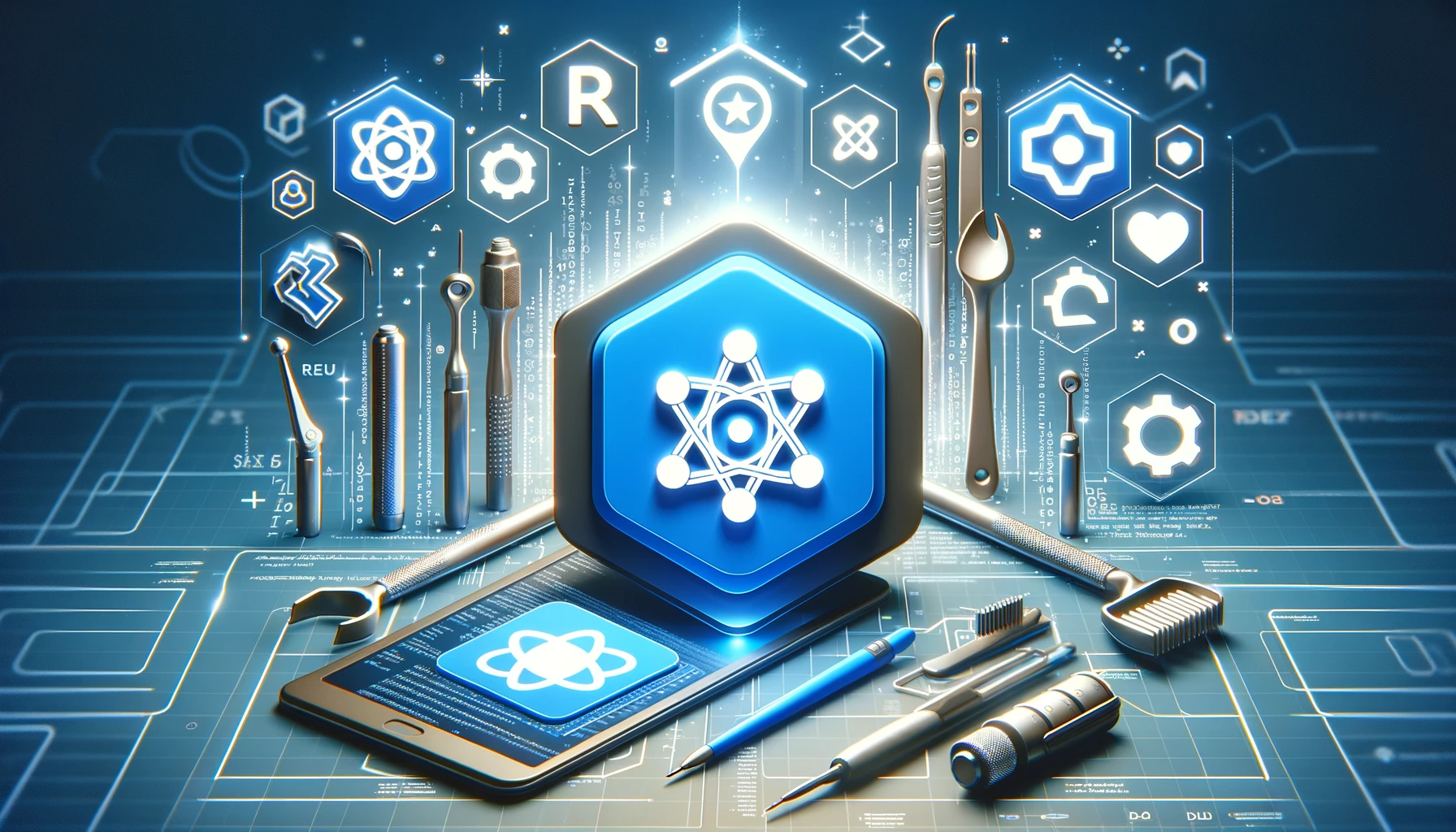
State management in React applications can be a challenging endeavor, especially when handling complex state logic. Redux, a popular state management library, has long been the go-to solution, but with its verbosity and boilerplate, developers often find themselves tangled in layers of repetition. Enter Redux Toolkit, a game-changer that simplifies Redux code, making state management more efficient and less error-prone.
Redux vs Redux Toolkit: A Comparative Glance
Redux, in its standard form, is powerful but verbose. It requires setting up actions, reducers, and optionally, middleware and thunks for asynchronous operations. This setup often leads to a lot of boilerplate code, which, while explicit, can be cumbersome and error-prone.
Redux Toolkit, on the other hand, streamlines this process. It encapsulates the best practices of Redux into a more manageable and concise API. The toolkit reduces the amount of code you need to write and maintain, making your application more robust and easier to understand.
Simplification: Redux Toolkit simplifies state management by abstracting the standard Redux patterns. It offers utility functions like
createSlice
andcreateAsyncThunk
, which significantly reduce the need for boilerplate code.Readability: With Redux Toolkit, you can define reducers, initial state, and actions all in one go using
createSlice
. This not only makes the code more concise but also enhances readability.Safety: Redux Toolkit includes Immer, a library that allows you to write simpler immutable updates. This means you can write code that “mutates” state in a way that is actually immutable, reducing the chances of accidental state mutations.
Exploring Redux Toolkit Features
CreateSlice: The Heart of Simplification
createSlice
is a function that accepts an initial state, an object of reducer functions, and a slice name, and automatically generates action creators and action types that correspond to the reducers and state.
import { createSlice } from '@reduxjs/toolkit';
const userSlice = createSlice({
name: 'user',
initialState: { loggedIn: false },
reducers: {
logIn: state => {
state.loggedIn = true;
},
logOut: state => {
state.loggedIn = false;
}
}
});
export const { logIn, logOut } = userSlice.actions;
export default userSlice.reducer;
In this example, createSlice
creates a user slice with logIn
and logOut
actions. The beauty of this approach is the elimination of separate action type constants and action creators, bundling everything into a single, readable construct.
CreateAsyncThunk: Managing Async Logic with Ease
Handling asynchronous logic in Redux can be complex, often requiring middleware like Redux Thunk. Redux Toolkit simplifies this with createAsyncThunk
.
import { createAsyncThunk } from '@reduxjs/toolkit';
export const fetchUserById = createAsyncThunk(
'users/fetchById',
async (userId, thunkAPI) => {
const response = await fetch(`https://api.example.com/users/${userId}`);
return response.data;
}
);
Here, createAsyncThunk
handles the async operation of fetching a user by ID. It automatically dispatches standard actions based on the lifecycle of the async request (pending, fulfilled, rejected), reducing the need for manual action dispatching.
Enhanced Configuration: configureStore
Redux Toolkit’s configureStore
function simplifies store setup and configuration. It automatically sets up the Redux DevTools extension and middleware like Redux Thunk.
import { configureStore } from '@reduxjs/toolkit';
import userReducer from './userSlice';
const store = configureStore({
reducer: {
user: userReducer
}
});
export default store;
In this example, configureStore
creates a Redux store with the userReducer
. It handles middleware and DevTools extension setup behind the scenes.
EntityAdapter: Normalizing State with Ease
Normalizing state is a common practice in Redux for efficient state updates and queries. Redux Toolkit provides createEntityAdapter
for this purpose.
import { createEntityAdapter, createSlice } from '@reduxjs/toolkit';
const userAdapter = createEntityAdapter();
const initialState = userAdapter.getInitialState();
const userSlice = createSlice({
name: 'users',
initialState,
reducers: {
addUser: userAdapter.addOne,
addUsers: userAdapter.addMany,
updateUser: userAdapter.updateOne,
removeUser: userAdapter.removeOne
}
});
export const { addUser, addUsers, updateUser, removeUser } = userSlice.actions;
export default userSlice.reducer;
The createEntityAdapter
simplifies the process of storing entities in a normalized form and provides utility functions for updating them.
Conclusion
Redux Toolkit is a robust and efficient solution for managing state in React applications. It streamlines Redux.