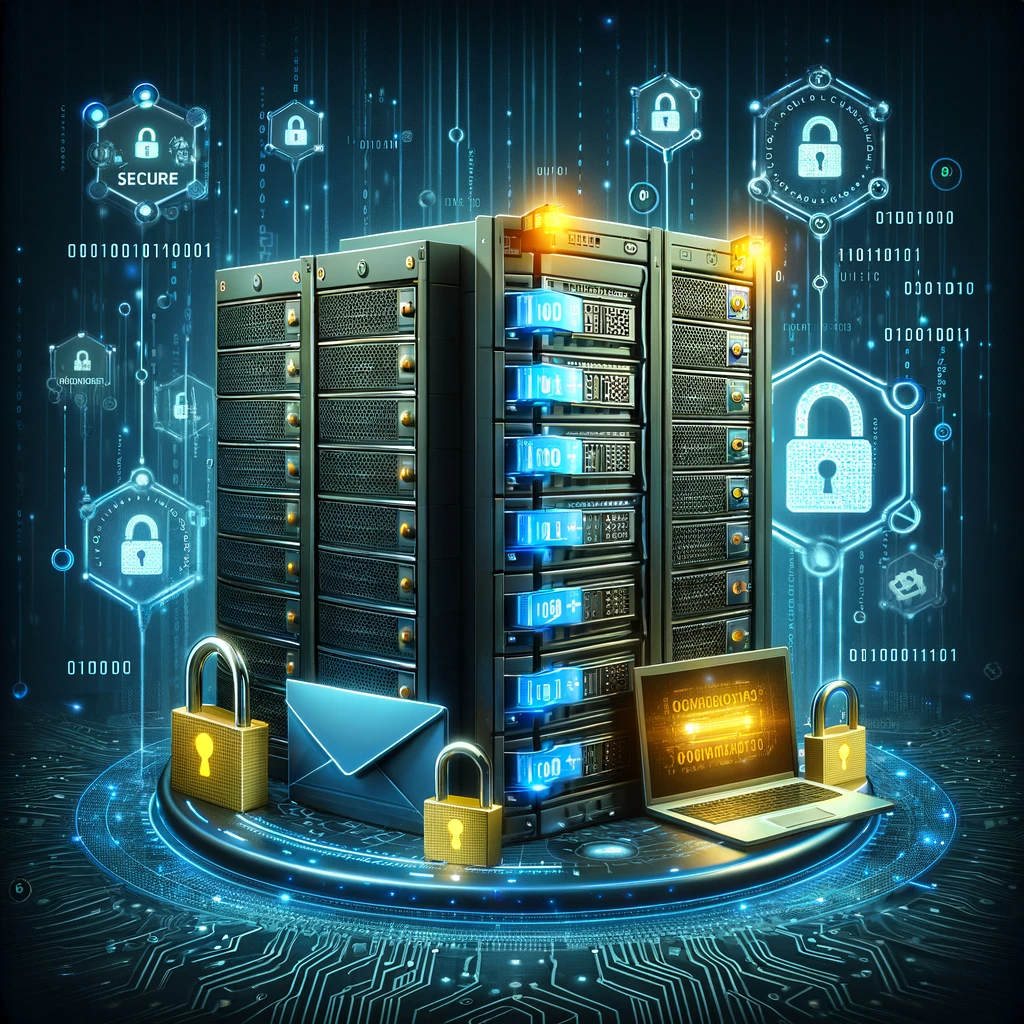
Webhooks have become a vital component in modern web applications, enabling real-time data sharing and event-driven architectures. However, as with any technology that facilitates data exchange, security is a paramount concern. In this post, we’ll delve into the best practices for securely signing webhooks, ensuring that the data your application sends and receives remains uncompromised.
Understanding Webhook Security
Webhooks, essentially user-defined HTTP callbacks, are triggered by specific events in your application. When an event occurs, your application sends an HTTP request to the URL configured for the webhook. The security risk here is clear: if a webhook is intercepted or impersonated, sensitive data could be exposed or corrupted.
The Role of Signing in Webhook Security
Signing webhooks is akin to sealing a letter with a unique wax seal. Just as the seal authenticates the letter’s origin and ensures its contents haven’t been tampered with during transit, signing a webhook verifies its source and integrity. This process involves generating a unique signature with each webhook request, using a secret key known only to the sender (your application) and the receiver (the server handling the webhook). This, however, does not prevent others from reading the leader as its contents are not encrypted.
Generating a Signature
The first step in securing a webhook is to generate a signature using a hash function. A popular choice is HMAC (Hash-based Message Authentication Code), which uses a secret key and the message data (the webhook payload) to produce a unique hash. In Python, this can be implemented as follows:
import hashlib
import hmac
def generate_signature(secret_key, message):
return hmac.new(secret_key.encode(), message.encode(), hashlib.sha256).hexdigest()
This function takes a secret_key
and the message
(your webhook payload) as inputs and returns a hexadecimal string representing the signature.
Attaching the Signature to the Webhook
Once the signature is generated, it must be included with the webhook request. Typically, this is done by adding it to the request headers. For example:
import requests
webhook_url = "https://example.com/webhook"
payload = {"event": "update", "data": {...}}
signature = generate_signature("your-secret-key", str(payload))
headers = {
"Content-Type": "application/json",
"X-My-App-Signature": signature
}
response = requests.post(webhook_url, json=payload, headers=headers)
In this snippet, the signature is added to the X-My-App-Signature
header. The receiver can then use the same secret key to generate a signature on their end and compare it to this header to verify the webhook’s authenticity.
Validating Webhooks
Upon receiving a webhook, the recipient must validate the signature before processing the request. This step is crucial for ensuring that the webhook has not been tampered with and that it comes from a trusted source.
Implementing Signature Validation
Here’s how you might implement signature validation in a Python-based server:
from flask import Flask, request
app = Flask(__name__)
@app.route('/webhook', methods=['POST'])
def webhook():
received_signature = request.headers.get('X-My-App-Signature')
expected_signature = generate_signature("your-secret-key", str(request.json))
if hmac.compare_digest(received_signature, expected_signature):
# Process the webhook
return "Webhook processed", 200
else:
return "Invalid signature", 403
In this Flask application, the server retrieves the signature from the X-My-App-Signature
header and compares it with a newly generated signature using hmac.compare_digest
. This method is used instead of a direct comparison to prevent timing attacks.
Best Practices for Webhook Security
- Keep Your Secret Key Safe: The security of your webhook signing process hinges on the secrecy of your key. Store it securely and never expose it in your client-side code.
- Use a Strong Hash Function: Prefer SHA-256 or SHA-3 for generating HMAC signatures. Avoid deprecated hash functions like MD5 and SHA-1.
- Validate Every Time: Always validate the signature of incoming webhooks before processing them. Don’t rely on IP whitelisting or other less secure methods alone.
- Monitor and Log Webhook Activity: Keep an eye on the webhooks your application sends and receives. Logging can help identify and respond to security incidents.
- Consider Using a Webhook Management Tool: Tools like Hookdeck or Webhook.site can simplify managing and securing webhooks, especially for more complex systems.
Additional Security Measures
While signing and validating webhooks provide a strong layer of security, it’s prudent to implement additional measures:
- HTTPS: Always use HTTPS for your webhook endpoints to ensure the data is encrypted during transit.
- Rate Limiting: Implement rate limiting on your webhook endpoints to mitigate the risk