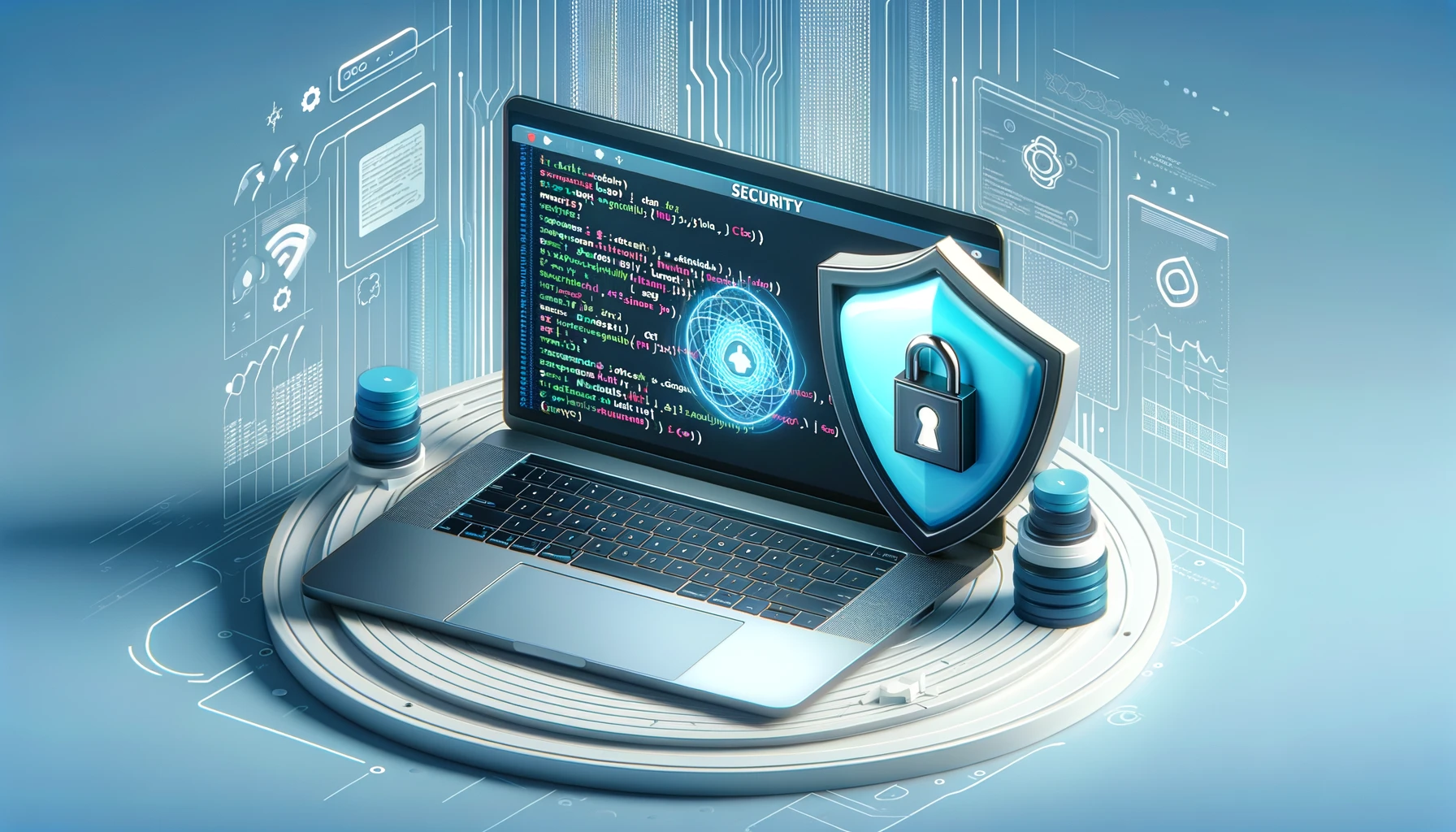
In the realm of web development, the use of Markdown for content creation has become increasingly popular due to its simplicity and readability. Showing user-generated content to other users always carries some sort of risk. While Markdown may seem like a solution to do this safely due to its plain text formatting, naive implementations can lead to security vulnerabilities like Cross-Site Scripting (XSS). This blog post delves into the nuances of securely rendering Markdown in React, highlighting tools like react-markdown.
Understanding the Security Risks of Markdown
Markdown, in its basic form, is a markup language with plain text formatting syntax. However, when Markdown is converted to HTML for rendering in web applications, there’s a risk of malicious code execution if the Markdown includes embedded HTML or JavaScript. This risk is particularly acute in applications that allow user-generated Markdown content.
Safe Markdown Rendering with react-markdown
One effective solution for rendering Markdown in React applications is using the react-markdown library. It’s designed to be secure by default, escaping any HTML contained within the Markdown. This means that tags like <script>
are not executed but are displayed as plain text, thereby mitigating the risk of XSS attacks.
Here’s a basic example of using react-markdown:
import React from 'react';
import ReactMarkdown from 'react-markdown';
const markdown = `# Hello world!\n\nThis is a Markdown text.`;
const MarkdownComponent = () => (
<ReactMarkdown>
{markdown}
</ReactMarkdown>
);
export default MarkdownComponent;
In this example, any Markdown content passed to ReactMarkdown
is safely rendered. This security feature makes react-markdown an excellent default choice for many React applications.
When HTML in Markdown is a Requirement
There are scenarios where you might want to allow HTML within Markdown—for instance, for advanced content formatting. This is where careful consideration is needed. Enabling HTML in Markdown can expose your application to XSS vulnerabilities if not handled correctly.
Integrating Tools like DOMPurify
In situations where you need to render HTML within Markdown, you’ll need to sanitize the HTML content to ensure it’s free from malicious scripts. While react-markdown doesn’t integrate directly with sanitization libraries, you can use a tool like DOMPurify independently to cleanse any HTML content before it’s rendered. You should ensure to use DOMPurify at a layer just before the HTML gets passed to dangerouslySetInnerHTML
, otherwise if the HTML is further modified after sanitization, it could no longer be safe.
DOMPurify works by purifying the HTML content, removing any unwanted and potentially harmful scripts, and leaving only safe-to-render HTML. However, it’s important to note that using DOMPurify or similar libraries adds complexity to your application and should only be done when absolutely necessary.
Content Security Policy: An Extra Layer of Defense
Content Security Policies (CSPs) are unrelated specifically to the topic of rendering Markdown or HTML in React, although we wanted to mention them briefly here as another layer of defence that you will want to ensure you have in place if you are doing something like rendering HTML in Markdown. A CSP is a browser-side mechanism that helps to detect and mitigate certain types of attacks, including XSS and data injection attacks.
A CSP allows you to do many things including specifying which domains are allowed to execute scripts, which can prevent malicious scripts from running even if they were to bypass other security measures. Implementing a CSP in conjunction with safe Markdown rendering practices adds an extra layer of security to your React application. This way if there is an XSS vulnerability in your Markdown rendering (or other parts of your application), the CSP can help to mitigate the risk of an attacker being able to execute injected malicious scripts.
This article doesn’t go in-depth on CSP, but this is largely just a call out to ensure that you investigate this further if you are on the path of rendering HTML in Markdown within your React application.
Conclusion
Rendering Markdown in React requires a balance between flexibility and security. Libraries like react-markdown provide a secure way to render Markdown by escaping HTML content. However, if your application requires HTML within Markdown, additional measures like using DOMPurify for sanitization are necessary. Remember, no security measure is foolproof, and combining multiple layers of defense, including a Content Security Policy, is always a best practice in securing your web applications.
Stay vigilant and prioritize security in your React applications, especially when dealing with user-generated content. By choosing the right tools and adhering to best practices, you can effectively mitigate the risks associated with rendering Markdown.