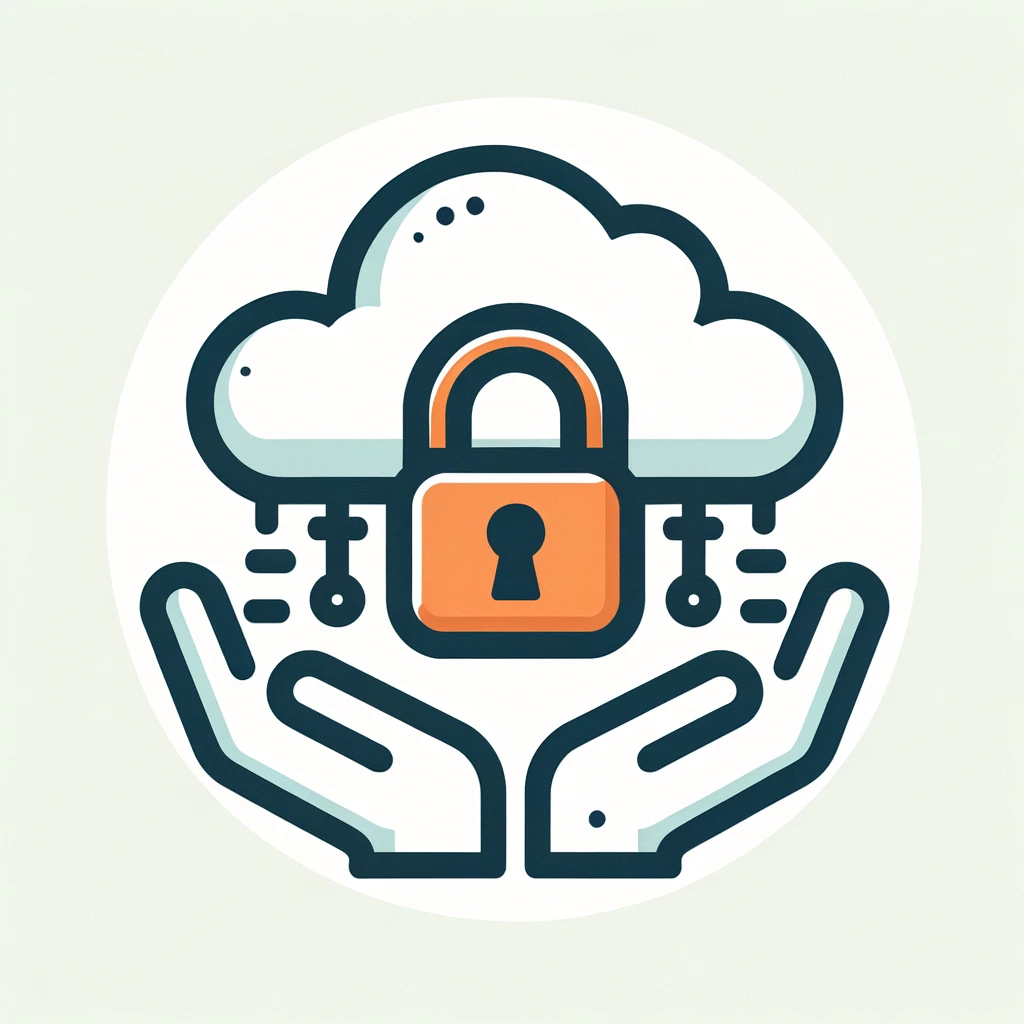
In the realm of web development, allowing users to upload files can significantly enhance the functionality of your web application. However, it can also open it up to a plethora of security risks. When improperly handled, file uploads can serve as a gateway for attackers to inject malicious files, leading to potential data breaches or server compromises. However, with the right precautions in place, you can mitigate these risks and secure your Flask application against such vulnerabilities. This blog post delves into secure file upload practices in Flask, focusing on filtering and validation techniques that safeguard your application.
Understanding the Risks
Before we dive into the solutions, it’s crucial to grasp the potential risks associated with file uploads. The primary concerns include:
- Malware Uploads: If an attacker manages to upload a malicious file, they could potentially harm the server, other files, or even users who download and execute the file.
- File Inclusion Vulnerabilities: Improper handling of file uploads can lead to remote code execution (RCE) vulnerabilities, where attackers execute arbitrary code on the server.
- Storage Consumption: Unrestricted file uploads can lead to denial of service (DoS) attacks by exhausting the server’s storage capacity.
Understanding these risks is the first step toward securing your Flask application against file upload vulnerabilities.
Flask Upload Basics
Flask, a micro web framework written in Python, makes it straightforward to handle file uploads. However, the simplicity of implementation does not inherently guarantee security. Let’s start with a basic example of handling file uploads in Flask:
from flask import Flask, request, redirect, url_for
from werkzeug.utils import secure_filename
app = Flask(__name__)
@app.route('/upload', methods=['POST'])
def upload_file():
if 'file' not in request.files:
return redirect(request.url)
file = request.files['file']
if file.filename == '':
return redirect(request.url)
if file and allowed_file(file.filename):
filename = secure_filename(file.filename)
file.save(os.path.join('/path/to/the/uploads', filename))
return 'File successfully uploaded'
else:
return 'Invalid file type'
if __name__ == '__main__':
app.run()
This code snippet provides a basic framework for handling file uploads, including the use of secure_filename
from Werkzeug to sanitize the file name. However, this alone does not fully secure your file upload feature. Let’s explore additional techniques to enhance security.
File Extension Filtering
One of the simplest yet effective ways to reduce the risk of malicious file uploads is to restrict the types of files that can be uploaded based on their extensions. This can be accomplished by maintaining a whitelist of allowed file types:
ALLOWED_EXTENSIONS = {'txt', 'pdf', 'png', 'jpg', 'jpeg', 'gif'}
def allowed_file(filename):
return '.' in filename and filename.rsplit('.', 1)[1].lower() in ALLOWED_EXTENSIONS
This function checks if the uploaded file’s extension is in the list of allowed extensions. While this approach is effective against many common attacks, it’s not foolproof. Attackers might still bypass these checks by disguising malicious files with allowed extensions.
Content-Type Validation
A more robust approach involves validating the MIME type (also known as the content type) of the uploaded file. This can be done using Python’s magic
library, which identifies file types by checking their headers rather than relying on extensions:
import magic
def allowed_mime_type(file):
mime = magic.from_buffer(file.stream.read(2048), mime=True)
file.stream.seek(0) # Reset file pointer after reading
return mime in ['image/png', 'image/jpeg', 'application/pdf']
This method reads the first few bytes of the file to determine its MIME type, ensuring that it matches one of the allowed types. This is significantly more reliable than merely filtering by file extension. However, even this method is not foolproof because attackers can craft files that have a malicious payload but still conform to the MIME type patterns expected by such validation.
For instance, an attacker could embed malicious code within what appears to be a legitimate image or PDF file. While the file might pass MIME type validation due to its correctly formatted header, it could still execute harmful actions if the application processes it in a vulnerable manner (e.g., displaying an image that exploits a vulnerability in the image processing library).
We will cover one strategy for dealing with this type of threat below, in the section on anti-virus scanning.
File Size Restrictions
Limiting the size of uploaded files is another critical step in securing file uploads. This can prevent attackers from overwhelming your server’s storage with excessively large files or launching DoS attacks. Flask allows you to set a maximum file size limit using the MAX_CONTENT_LENGTH
configuration:
app.config['MAX_CONTENT_LENGTH'] = 16 * 1024 * 1024 # 16 megabytes
Attempting to upload a file larger than the specified limit will result in a 413 Request Entity Too Large
error from Flask. Since Flask applications often have a web server sitting in front of them, it is a good idea to also configure this limit for the web server itself.
For example, nginx and Apache have configuration options that specify the maximum allowed size of a client’s request body, thus providing an additional layer of defense against large file uploads and other large payloads. Configuring these limits ensures that oversized files are rejected before they even reach the application, enhancing security and efficiency.
server {
listen 80;
server_name yourdomain.com;
client_max_body_size 8M;
location / {
proxy_pass http://127.0.0.1:3000;
}
}
If using Nginx, the client_max_body_size
setting above will limit HTTP body payloads to 8M
of size.
<VirtualHost *:80>
ServerName yourdomain.com
# Limit the size of the HTTP request body to 8 MB
LimitRequestBody 8388608
# Proxy HTTP requests to another server
ProxyPass / http://127.0.0.1:3000/
ProxyPassReverse / http://127.0.0.1:3000/
</VirtualHost>
The LimitRequestBody
setting above will do the same for Apache.
Anti-virus Scanning
For applications dealing with a high risk of malware uploads, integrating an anti-virus scanner can add an extra layer of security. This involves scanning uploaded files for viruses or malware before they’re stored or processed by your application. Various anti-virus solutions offer APIs that can be integrated into your Flask application, providing real-time scanning capabilities.
One of these anti-virus solutions is ClamAV, which stands out as a particularly effective and accessible choice for integrating malware scanning into your Flask application. ClamAV is an open-source (GPL) anti-virus engine used in a variety of situations including email scanning, web scanning, and endpoint security. It provides numerous features, including support for multiple file formats, signature-based malware detection, and a versatile scanning API.
First, you need to install ClamAV on your server. This can typically be done through your operating system’s package manager. For example, on Ubuntu, you would use:
sudo apt-get install clamav clamav-daemon
After installation, ensure that the ClamAV daemon is running and up to date with the latest virus databases:
sudo systemctl start clamav-daemon
sudo freshclam
To integrate ClamAV scanning into your Flask application, you can use Python’s pyclamd
library, which allows your application to communicate with the ClamAV daemon. Install pyclamd
using pip:
pip install pyclamd
Now, you can incorporate it into your route handler as follows:
import pyclamd
from flask import Flask, request, jsonify
app = Flask(__name__)
def scan_file(file_path):
cd = pyclamd.ClamdUnixSocket()
result = cd.scan_file(file_path)
return result
@app.route('/upload', methods=['POST'])
def upload_file():
file = request.files['file']
file_path = '/path/to/uploads/' + file.filename
file.save(file_path)
scan_result = scan_file(file_path)
if scan_result:
return jsonify({"message": "Malware detected, upload blocked!"}), 403
else:
return jsonify({"message": "File uploaded successfully."})
if __name__ == '__main__':
app.run()
Conclusion
Securing file uploads in Flask requires a comprehensive approach that combines multiple techniques. By filtering file extensions, validating MIME types, limiting file sizes, and potentially integrating anti-virus scanning like ClamAV, you can significantly reduce the risks associated with allowing users to upload files to your application. Remember, security is not about implementing a single solution but layering different strategies to create a robust defense against potential attacks.
Incorporating these practices into your Flask application not only protects your server and data but also builds trust with your users, ensuring they can safely upload files without compromising the security of your application or their own data. For an in-depth guide and more advanced techniques on securing file uploads, the OWASP File Upload Cheat Sheet is an invaluable resource. It provides a detailed overview of the risks involved in file uploads and practical recommendations for mitigating these risks, complementing the strategies discussed in this post.