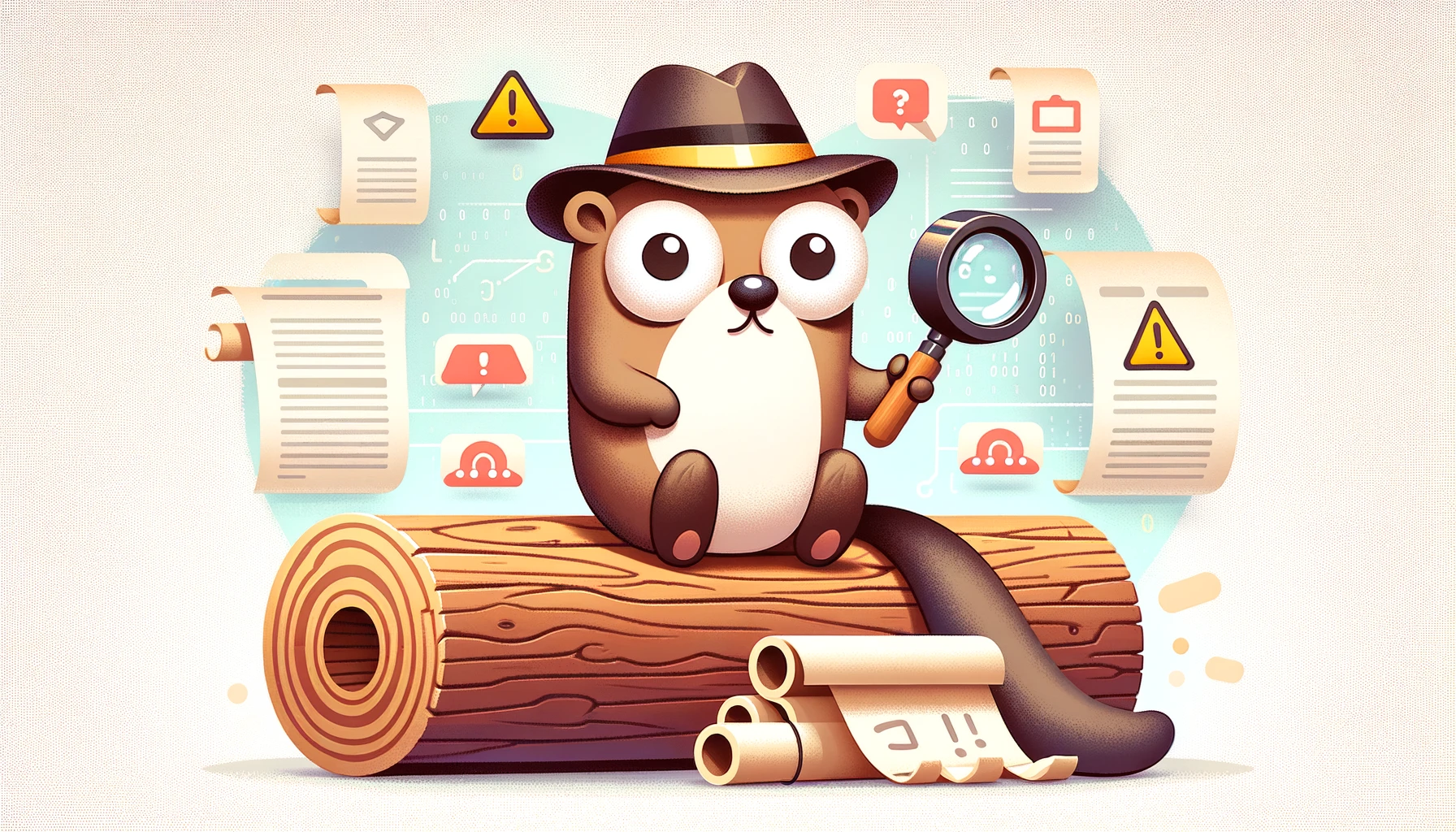
Logging is an essential aspect of software development, serving as a window into the operational health and behavior of applications. In Golang, effective logging is not just about recording data but ensuring that these logs contribute to the security and monitoring of the application. This post will explore the nuances of logging in Golang, focusing on its built-in capabilities and external libraries like Apex, to enhance both security and monitoring.
Understanding the Basics of Logging in Golang
Golang, with its simplicity and efficiency, offers basic logging capabilities through its standard library “log”. This package provides simple logging functions like Println
, Fatal
, and Panic
, which are straightforward to use but might be insufficient for more complex applications. They write to standard error (stderr) and are suitable for small-scale applications or those in early development stages.
Example: Basic Logging with the log
Package
import "log"
func main() {
log.Println("This is a basic log message")
}
This snippet demonstrates a simple log message, a starting point for most Go developers.
Enhancing Logging with Structured Logging
Structured logging is a format where logs are written as structured data (like JSON), making them easier to search and analyze. It is particularly beneficial in the context of security and monitoring, where understanding the context of logs is critical.
Using External Libraries for Structured Logging
While Go’s standard library doesn’t directly support structured logging, external libraries like Apex/log
and logrus
fill this gap. They offer more advanced features like JSON output, log levels, and hooks for sending logs to various destinations.
Apex/log: A Lightweight and Extensible Library
Apex/log
stands out for its simplicity and extensibility. It supports structured logging and provides handlers for various outputs like JSON.
Example: Using Apex/log for Structured Logging
import (
"github.com/apex/log"
"github.com/apex/log/handlers/json"
)
func main() {
log.SetHandler(json.New(os.Stderr))
log.WithFields(log.Fields{
"module": "billing",
"id": 123,
}).Info("Processing payment")
}
In this example, we configure Apex/log
to output logs in JSON format, enriching them with contextual information like module and ID.
Implementing Log Levels for Better Monitoring
Log levels (like INFO, WARN, ERROR) are crucial for categorizing the importance of log messages. They help in filtering logs for relevant information during debugging and monitoring.
Avoid log.Fatal
It is generally recommended to avoid using log.Fatal
unless absolutely necessary. This function terminates the application after logging the message, which might not be desirable especially in
packages other than main
.
Security Considerations in Logging
Security in logging is often overlooked. Logs can contain sensitive information, which, if exposed, can lead to security vulnerabilities.
Best Practices for Secure Logging
- Sanitization: Ensure that logs do not contain sensitive information like passwords or personal identifiable information (PII).
- Access Control: Restrict access to log files. They should be accessible only to authorized personnel.
- Encryption: In certain cases, encrypting log files is necessary, especially when they contain sensitive data.
- Monitoring Log Integrity: Regularly monitor logs for signs of tampering or unauthorized access.
- Avoid broad payload logging: This goes back to #1, but it’s worth emphasizing. Avoid logging artbirary payloads from things like http requests or responses. These payloads can contain sensitive information that can be exposed in logs unintentionally.
Example: Sanitizing Logs
func sanitize(input string) string {
// Implementation of sanitization logic
return sanitizedInput
}
func main() {
userInput := "Sensitive Info"
log.Println("User input:", sanitize(userInput))
}
In this example, the sanitize
function would contain logic to remove or mask sensitive information.
Conclusion
Effective logging in Go is more than just recording data; it’s about creating a secure and analyzable log environment. By utilizing Go’s built-in capabilities and external libraries like Apex/log, developers can achieve a robust logging system. Remember, the key to effective logging lies in structured data, appropriate log levels, and ensuring the security of log data. Implementing these practices will significantly aid in both monitoring and securing your Go applications.
This exploration of logging in Golang provides insights into both built-in functions and external libraries, emphasizing structured logging, log levels, and security considerations. Whether you’re starting a new project or looking to enhance an existing
one, these practices and tools will help create a more secure and monitorable application environment.