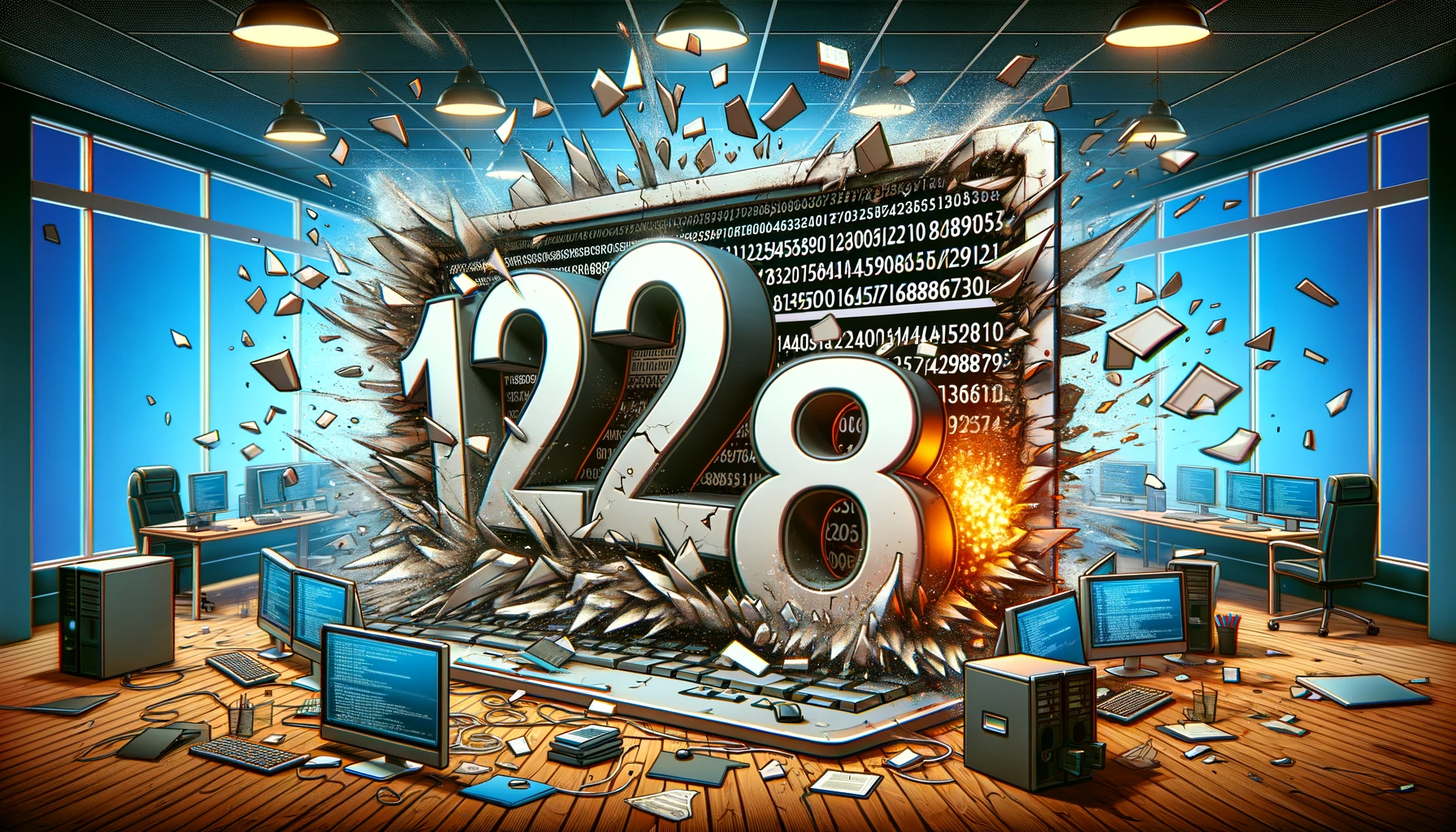
In the world of software development, the transmission of data in a structured, reliable format is crucial. One of the most ubiquitous data interchange formats is JSON (JavaScript Object Notation). While JSON is versatile and user-friendly, it has its nuances, especially when dealing with large integers. Below, we’ll dive into the challenges presented when serializing and deserializing large integers in JSON, and offer some practical guidance to ensure you don’t run into any issues in your code.
Understanding JSON’s Limitations with Large Integers
JSON, while flexible, does not specify or enforce any limits on number precision. This means that the handling of large numbers can vary between different JSON parsers and JavaScript engines. In JavaScript, for instance, the Number
type follows the IEEE 754 standard for floating-point arithmetic, which can safely represent integers up to 2^53 - 1. Beyond this, precision issues can arise.
Precision Loss
Consider a scenario where your application needs to process a large integer, such as a 64-bit identifier from a database. In JavaScript:
// A large integer
const largeInt = 12345678901234567890;
// Converting to JSON
const jsonString = JSON.stringify({ id: largeInt });
// Parsing back to JavaScript
const parsedData = JSON.parse(jsonString);
console.log(parsedData.id); // Output may have less precision (eg., 12345678901234567890)
In this example, parsedData.id
may not accurately represent the original integer due to precision loss.
Strategies for Handling Large Integers
To mitigate these issues, we can employ several strategies.
1. Stringify Large Integers
A common practice is to treat large integers as strings:
const largeInt = 12345678901234567890n; // Using BigInt for representation
const jsonString = JSON.stringify({ id: largeInt.toString() });
const parsedData = JSON.parse(jsonString);
const originalBigInt = BigInt(parsedData.id);
console.log(originalBigInt === largeInt); // true
Rather than doing this manually each time, JSON.parse
has a custom reviver parameter that can automate the process of reviving BigInts
from strings. See MDN: JSON.parse Reviver Parameter.
2. Custom JSON Parsers
Some libraries offer custom JSON parsers that can handle large integers gracefully. For instance, json-bigint
is a popular npm package that parses large integers as BigInt
objects in JavaScript.
const JSONbig = require('json-bigint');
const jsonString = '{"id": 12345678901234567890}';
const parsedData = JSONbig.parse(jsonString);
console.log(typeof parsedData.id); // 'bigint'
Conclusion
Handling large integers in JSON requires careful consideration to avoid precision loss. By stringifying large integers or using custom JSON parsers, we can ensure data integrity. To delve deeper into this topic, explore JavaScript’s BigInt and npm packages like json-bigint. Remember, attention to these details can significantly impact the functionality and reliability of your software.