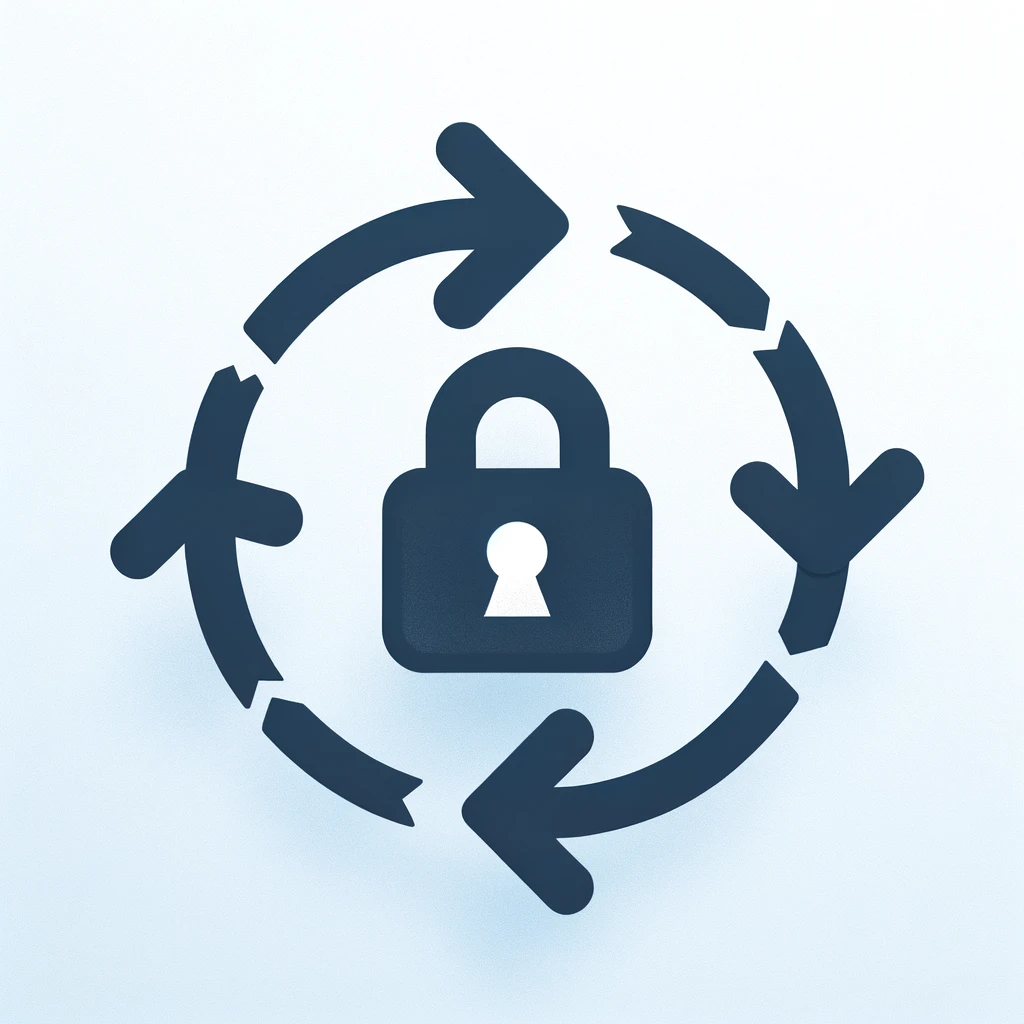
Rust, a system programming language focused on safety and performance, has garnered significant attention for its unique approach to managing memory and concurrency. Concurrency, the ability for a computer program to do many tasks at once, is notorious for its complexity and potential security vulnerabilities. However, Rust’s ownership, types, and concurrency models offer a refreshing paradigm for writing secure, concurrent applications. In this post, we’ll dive into how Rust enables developers to embrace concurrency without the usual fears of data races, memory safety vulnerabilities, and the daunting complexity that can come with high-performance threading.
The Rust Guarantee: Safety and Control
At the heart of Rust’s concurrency model is the promise of memory safety without sacrificing performance. Rust achieves this through its ownership system, which ensures that memory is automatically cleaned up when it’s no longer needed, and through strict compile-time checks that prevent data races. A data race occurs when two or more threads access the same memory location concurrently, at least one of the accesses is a write, and at least one of them is unsynchronized (The Rustnomicon: “Data Races and Race Conditions”). Rust’s type system and borrowing rules make these situations impossible at compile time, rather than trying to find them at runtime.
Ownership, Borrowing, and Lifetimes: The First Line of Defense
Ownership in Rust is a set of rules that the compiler checks at compile time, which means that it doesn’t have to use up any runtime cycles, which would add unnecessary overhead to your program. Here’s a quick rundown:
- Ownership: Each value in Rust has a single owner – the variable that’s responsible for the value’s memory. When the owner goes out of scope, the memory is freed up.
- Borrowing: Rust allows references to data without taking ownership of it, enabling both mutable and immutable references. However, it enforces a strict rule: you can either have one mutable reference or any number of immutable references to a particular piece of data, but not both. This rule by itself eliminates a whole class of concurrency bugs.
- Lifetimes: Rust gives the code author the ability to specify regions of the code in which the value can be assumed to be valid. Generally, these lifetimes can resemble scopes (blocks of code between
'{'
and'}'
characters), however they can also get more complicated than that. This feature is out of the scope of this article, but you can read about it here: The Rustnomicon: “Lifetimes”.
Threads in Rust: Safe Concurrency in Action
Rust’s standard library provides a thread API that allows you to run code concurrently by creating new threads. Here’s a simple example of creating a new thread:
use std::thread;
fn main() {
thread::spawn(|| {
// Perform some work in a new thread
println!("Hello from a new thread!");
});
println!("Hello from the main thread!");
}
This code snippet demonstrates Rust’s ease of use when it comes to spinning up new threads. The real power, however, lies in Rust’s ability to prevent data races through its type system and ownership rules.
Sharing State Safely Between Threads
When it comes to sharing state between threads, Rust provides several tools, such as Mutex
, RwLock
, and atomic types, which ensure that memory safety is maintained. Let’s focus on Mutex
(mutual exclusion), a fundamental tool for thread safety.
A Mutex
allows only one thread to access some data at any given time. To access the data, a thread must first acquire the mutex’s lock. Here’s an example:
use std::sync::{Arc, Mutex};
use std::thread;
fn main() {
let counter = Arc::new(Mutex::new(0));
let mut handles = vec![];
for _ in 0..10 {
let counter = Arc::clone(&counter);
let handle = thread::spawn(move || {
let mut num = counter.lock().unwrap();
*num += 1;
});
handles.push(handle);
}
for handle in handles {
handle.join().unwrap();
}
println!("Result: {}", *counter.lock().unwrap());
}
In this example, Arc
(atomic reference counting) is used to share ownership of a Mutex
across multiple threads, and Mutex
ensures that only one thread at a time can access the inner value. Each thread acquires the lock, updates the value, and then releases the lock, ensuring safe concurrent modifications.
Leveraging Advanced Concurrency Features
Rust also offers advanced concurrency features through its ecosystem. Crates such as rayon
provide data parallelism, tokio
and async-std
for asynchronous programming, and crossbeam
for non-blocking data structures. These tools and libraries allow developers to build scalable, high-performance concurrent applications while maintaining Rust’s safety guarantees.
Best Practices for Secure Concurrency in Rust
- Leverage Rust’s Type System: Always use Rust’s type system to your advantage. Let the compiler catch errors for you.
- Prefer Immutable References: Whenever possible, prefer using immutable references. This practice naturally avoids many concurrency issues.
- Use
Arc
andMutex
Wisely: Understand when to useArc
for shared ownership andMutex
for mutual exclusion. Overuse can lead to performance bottlenecks. - Explore the Ecosystem: Familiarize yourself with Rust’s concurrency ecosystem. Libraries like
tokio
for async programming andrayon
for parallelism can significantly simplify complex concurrent tasks. - Understand Ownership, Borrowing, and Lifetimes: Deeply understanding ownership, borrowing, and lifetimes is crucial. Without understanding these concepts, you will spend an inordinate amount of time fighting them to get your program working. However, if you master all three, it will be much easier to write secure concurrent code.
Conclusion
Rust provides an empowering framework for handling concurrency with confidence. Its ownership model, combined with the borrowing rules and type system, naturally guide developers away from common pitfalls associated with concurrent programming. By leveraging Rust’s tools and ecosystem, developers can achieve high-performance concurrent applications. As concurrenty is only one aspect of secure coding practices, it would help to better familiarize yourself with other aspects of the language, and as always, make sure you have your code reviewed throrougly by experts.