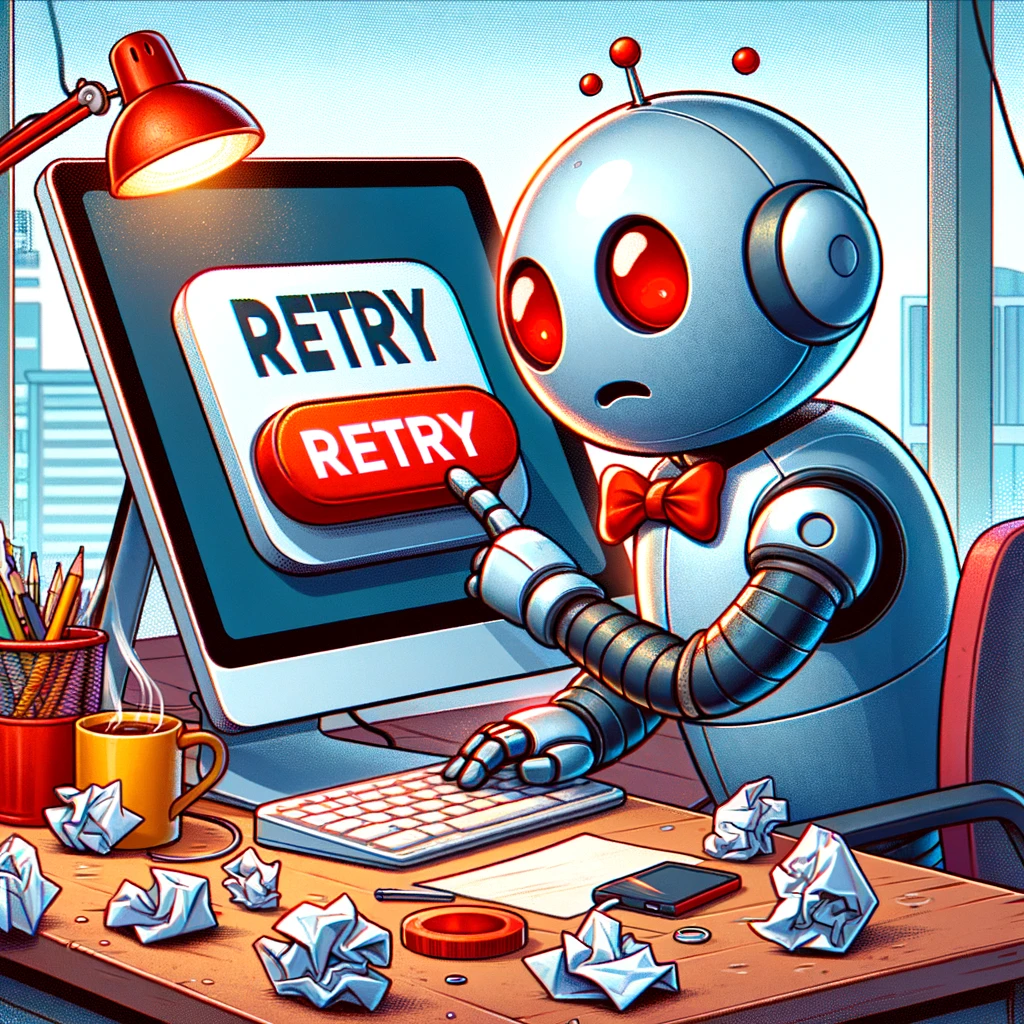
In networked applications, the adage “try and try again” is not just a motivational phrase but a practical necessity. However, mere retries are not enough; how and when we retry can make the difference between a robust application and one that crumbles under pressure. This is where the concepts of retrying and exponential backoff come into play, ensuring our applications are resilient in the face of transient errors and fluctuating network conditions.
Understanding the Need for Retrying
To begin, let’s consider a common scenario: Your application is trying to fetch data from a remote server. This operation can fail for a myriad of reasons – network issues, server overload, brief disruptions in service, etc. If the application simply gives up after the first failure, it risks missing out on the opportunity to succeed in a subsequent attempt. This is where retrying comes in as a basic yet crucial mechanism.
import requests
def fetch_data(url):
try:
response = requests.get(url)
response.raise_for_status()
return response.json()
except requests.RequestException as e:
print(f"Error fetching data: {e}")
return None
In this simple Python example, if the network call fails, the application logs the error and returns None
. However, this approach doesn’t attempt a retry, which could potentially resolve a transient error.
Naive Retrying
A naive approach to retrying would be to simply wrap the operation in a loop and retry until it succeeds. Here’s how you might implement this in Python:
import requests
def fetch_data(url, max_retries=5):
for attempt in range(max_retries):
try:
response = requests.get(url)
response.raise_for_status()
return response.json()
except requests.RequestException as e:
print(f"Error fetching data: {e}")
return None
The Power of Exponential Backoff
Constant retries can be problematic – imagine if every client hitting a temporarily overwhelmed service just kept trying without pause. This could lead to further strain on the service, exacerbating the problem. This is where exponential backoff, a more sophisticated strategy, comes into play.
Exponential backoff involves increasing the delay between retry attempts exponentially. This approach balances the need to retry operations with the need to reduce load on the service or network. It works by making the delay period longer after each failed attempt, reducing the probability of many clients retrying simultaneously.
Here’s how you might implement a basic exponential backoff in Python:
import time
import random
def fetch_with_backoff(url, max_retries=5):
retry_delay = 1 # Initial delay in seconds
for attempt in range(max_retries):
try:
response = requests.get(url)
response.raise_for_status()
return response.json()
except requests.RequestException:
time.sleep(retry_delay)
retry_delay *= 2 # Double the delay for the next attempt
retry_delay += random.uniform(0, 1) # Add jitter
raise Exception("Maximum retry attempts reached")
In this example, the delay before the next retry attempt doubles each time a request fails. The addition of a random “jitter” avoids the scenario where many clients, following the same exponential backoff algorithm, retry simultaneously.
Best Practices and Considerations
While implementing retries and exponential backoff, there are several best practices to consider:
- Limit the Number of Retries: Always set a maximum limit on retries to prevent infinite loops. If you do need to retry indefinitely, ensure that there is a maximum retry_delay to avoid the exponential backoff from growing too large (weeks, months, etc.).
- Consider the Type of Error: Not all errors are worth retrying. For example, retrying after a 404 (Not Found) error usually doesn’t make sense.
- Use Jitter: Jitter helps prevent synchronized retries from many clients, which can create additional load at regular intervals.
- Be Mindful of Timeout Settings: Ensure that your overall timeout settings for the operation are adjusted to account for the time spent in retries.
- Logging and Monitoring: Log retry attempts and monitor the rate of retries to understand the health of the external services and the network.
Real-World Application
Let’s consider a real-world application of these concepts. Imagine you’re developing a microservice architecture where services constantly communicate over the network. Implementing exponential backoff in inter-service communication can significantly enhance the resilience of your system. It helps in gracefully handling spikes in traffic, temporary service degradations, and network instabilities.
Conclusion
Retrying with exponential backoff is a simple yet powerful pattern for building more reliable and resilient applications. It’s an essential tool in the arsenal of any software developer, particularly in the realm of networked applications and microservices. Implementing this pattern correctly can drastically reduce the impact of transient errors and improve the user experience.
Remember, the goal isn’t to eliminate errors but to manage them intelligently, ensuring that your application remains robust and responsive under varying conditions. With these strategies in place, your software is not only prepared to face failure but is also designed to learn from it and adapt accordingly.