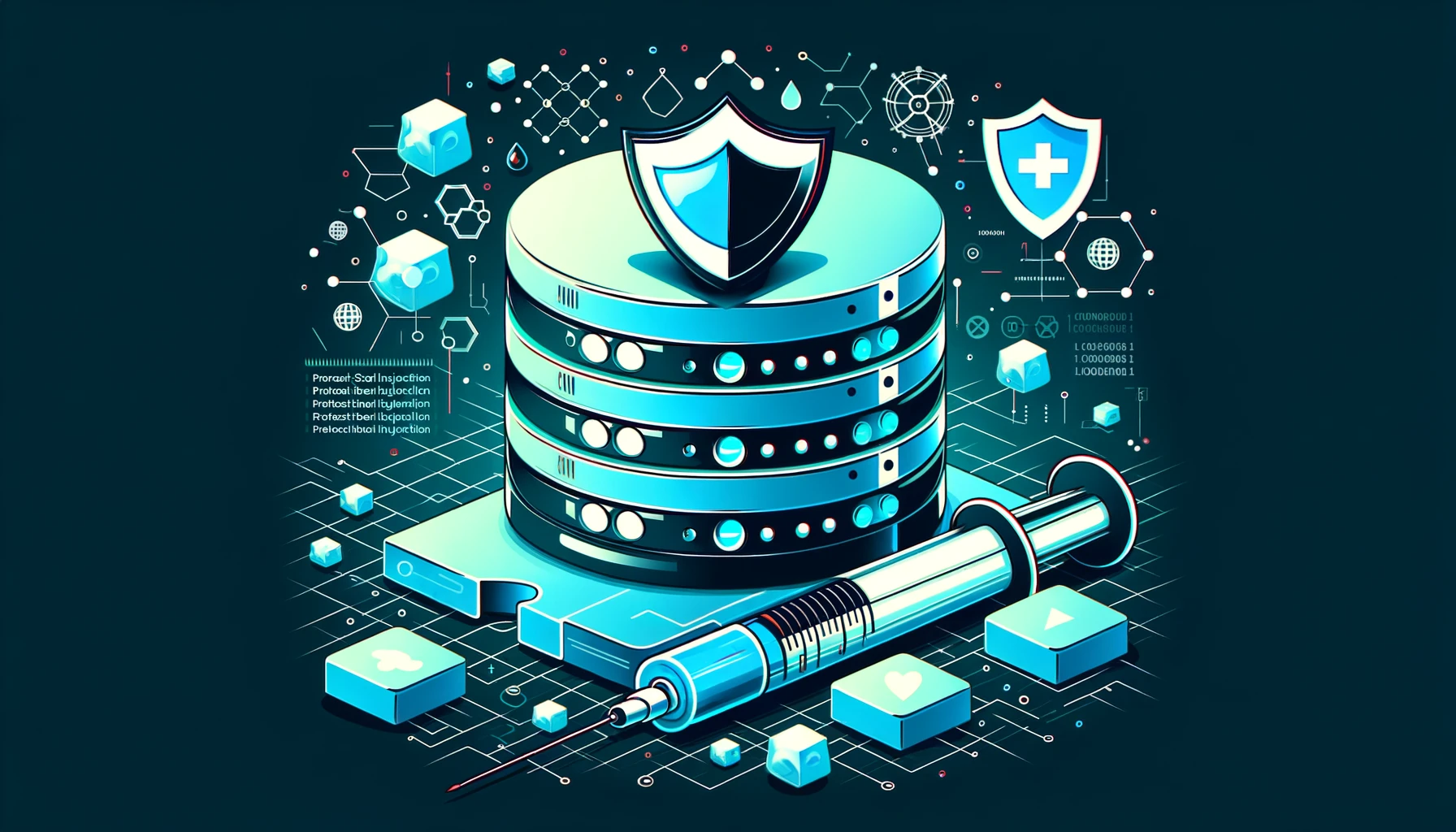
SQL injection continues to be a prominent threat in the realm of software security, posing significant risks to applications. For software developers, it’s essential to understand and effectively counteract this threat. This article explores SQL injection in the context of Golang, offering practical strategies to fortify your applications.
Understanding SQL Injection in Golang
SQL injection attacks involve the manipulation of SQL queries by injecting malicious input, potentially leading to unauthorized data access, corruption, or even system compromise. Despite Golang’s efficiency and simplicity, it remains just as susceptible to SQL injection as most other programming languages, especially when secure coding practices are disregarded.
The Risk in Context
Consider a Golang application interfacing with a database. SQL injection risk emerges when user inputs are directly used in SQL queries without adequate validation or sanitization.
Example of Vulnerable Code:
func getUser(db *sql.DB, username string) (*User, error) {
query := fmt.Sprintf("SELECT * FROM users WHERE username = '%s'", username)
return db.Query(query)
}
Here, the username
variable is concatenated directly into the SQL query. For instance, an attacker could use a username like '; DROP TABLE users;--
, which, when concatenated, would result in a malicious query that could delete the entire users table. This highlights the potential entry point for attackers.
Best Practices for Mitigation
1. Use Parameterized Queries
The foremost line of defense against SQL injection is employing parameterized queries, which safely handle user inputs.
Secure Code Example:
func getUser(db *sql.DB, username string) (*User, error) {
query := "SELECT * FROM users WHERE username = ?"
return db.Query(query, username)
}
In this method, username
is treated as a parameter, separate from the SQL command itself. The sql
package in Golang intelligently handles these parameters, ensuring they’re treated purely as data and not executable code. This process effectively mitigates the risk of SQL injection. It’s important to note that the placeholder syntax (like ?
in the example above) can vary between different databases. Some may use numbered placeholders like $1
. Developers should refer to the documentation of their specific SQL driver for accurate syntax.
2. Employ Prepared Statements
Prepared statements, a form of parameterized queries, are pre-compiled SQL statements that can be reused with varied parameters. They enhance both security and performance.
Implementation:
stmt, err := db.Prepare("SELECT * FROM users WHERE username = ?")
if err != nil {
return nil, err
}
defer stmt.Close()
user := stmt.QueryRow(username)
return user, nil
3. Input Validation and Sanitization
Validating and sanitizing user input is a critical aspect. It’s important to ensure the input matches expected formats and is cleansed of potentially harmful characters.
Example:
if !isValidUsername(username) {
return nil, errors.New("invalid username")
}
The isValidUsername
function should be designed to rigorously check against expected username patterns, potentially using regular expressions. It might also include escaping harmful SQL characters to prevent data corruption and avoiding HTML character encoding to thwart cross-site scripting (XSS) attacks.
4. Regularly Update Database Drivers and Libraries
Staying updated with the latest database drivers and libraries is crucial, as these updates often contain fixes for known vulnerabilities. Checkout the Go Wiki: SQL Database Drivers.
Conclusion
While SQL injection poses a serious threat, adherence to best coding practices can effectively mitigate this risk. Golang developers can significantly enhance their application security by employing parameterized queries, prepared statements, stringent input validation, and keeping their tools updated. Security is a continuous journey in the development process, demanding constant vigilance and proactive measures.