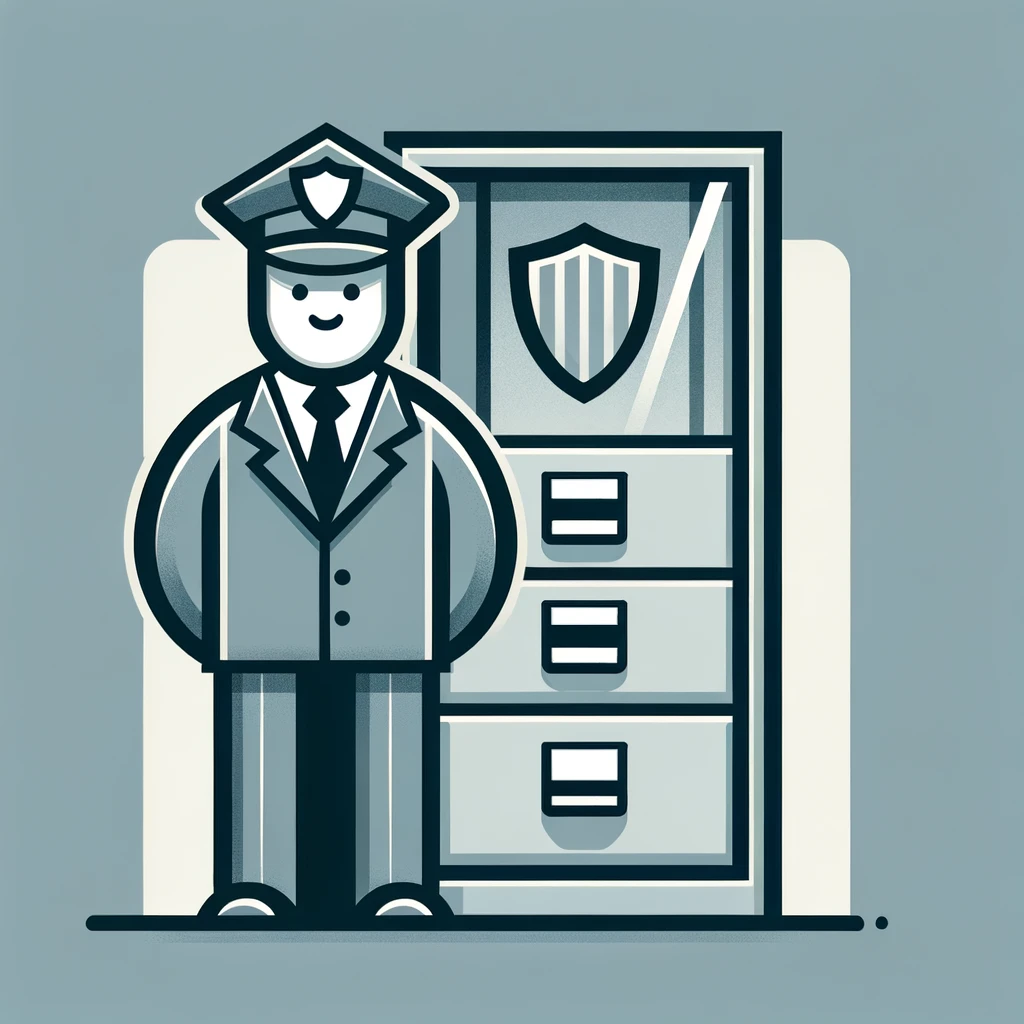
Directory traversal attacks, also known as path traversal attacks, exploit web applications by accessing files and directories that are stored outside the web root folder. By manipulating variables that reference files with dot-dot-slash (../) sequences, attackers can access arbitrary files and directories stored on the file system, including application source code, data, and sensitive system files. This vulnerability can lead to unauthorized access, information disclosure, and even full system compromise. Therefore, securing your application against directory traversal attacks is paramount for maintaining its integrity and protecting sensitive information.
Understanding the Vulnerability
A directory traversal attack occurs when an attacker manipulates file paths in a way that allows them to access directories and files they shouldn’t be able to. This is often possible due to insufficient input validation and sanitization when user input is used to construct file paths for file operations like open, read, and write.
Consider a simple file retrieval feature in a web application that allows users to view files by specifying a filename in a query parameter, like so: http://example.com/getFile?filename=report.txt
. An attacker can exploit this feature by requesting a file outside the intended directory with a request like http://example.com/getFile?filename=../../../../etc/passwd
, aiming to access the system’s password file.
Mitigation Techniques
Validate and Sanitize Input
Input validation is the first line of defense against directory traversal attacks. Ensure that your application validates user input strictly, rejecting any request containing illegal characters or patterns. For file access operations, specifically disallow characters such as “..” and “/”, or sequences that are known to be part of directory traversal payloads.
Sanitizing input by removing or encoding dangerous characters before processing can further reduce the risk. However, validation should always be your primary strategy, with sanitization serving as an additional layer of security.
Use Allow Lists
Implementing allow lists (previously known as whitelists) for file retrieval operations is an effective way to prevent directory traversal attacks. By explicitly specifying which files or directories are accessible, you can ensure that only intended resources can be accessed, regardless of any manipulation attempts by attackers.
Employ Built-in Functions for File Access
Many programming languages and frameworks offer built-in functions that automatically handle path normalization and can effectively mitigate directory traversal vulnerabilities. For example, using functions like realpath()
in PHP or Path.Combine()
in .NET ensures that file paths are resolved to their absolute path, stripping out any potentially malicious sequences.
Utilize Chroot Jails and Virtual Directories
Isolating your application’s execution environment using chroot jails (in Unix-like systems) or virtual directories (in web servers) can limit the scope of accessible directories for the application. This containment strategy ensures that even if an attacker manages to exploit a directory traversal vulnerability, they are confined to a controlled environment, significantly reducing the potential impact.
Regularly Update and Patch
Keeping your software and dependencies up-to-date is crucial in protecting against known vulnerabilities, including those that could be exploited for directory traversal. If one of your dependencies has a vulnerability, then your application has a vulnerability. Regularly applying patches and updates to your operating system, web server, application server, and any frameworks or libraries you are using makes your project a moving target for any would-be hackers.
Implement Access Control Measures
Proper access control mechanisms can restrict the files and directories accessible to different users or processes. Implementing least privilege access principles ensures that even if an attacker bypasses other defenses, they cannot access sensitive files or directories without the necessary permissions.
Monitor and Log File Access
Monitoring and logging file access attempts can help in detecting and responding to potential directory traversal attacks. By keeping an eye on unusual patterns or repeated access attempts to sensitive files, you can identify malicious activity and take appropriate action before any significant damage occurs.
Practical Examples
To illustrate some of these principles, let’s look at practical examples in a couple of popular programming languages.
Example in Python
Python’s os.path
module offers utilities for safely constructing file paths. The os.path.join
function ensures that the resulting path is constructed correctly according to the operating system’s conventions, while os.path.abspath
can be used to resolve the absolute path, mitigating directory traversal attempts.
import os
def safe_file_access(file_name, base_directory="/var/www/documents"):
# Construct the full filepath
file_path = os.path.join(base_directory, file_name)
# Resolve the absolute path and ensure it's within the base_directory
absolute_path = os.path.abspath(file_path)
if not absolute_path.startswith(base_directory + os.path.sep):
raise ValueError("Invalid filename")
# Proceed with file access
with open(absolute_path, 'r') as file:
return file.read()
Example in PHP
PHP’s realpath()
function is useful for resolving absolute pathnames and eliminating any “..” sequences. This can be used to check if the resolved path starts with an expected directory path.
<?php
function safeFileAccess($fileName, $baseDirectory = "/var/www/documents") {
// Ensure the base directory path is absolute
$baseDirectory = realpath($baseDirectory);
// Resolve the absolute path of the requested file
$filePath = realpath($baseDirectory . '/' . $fileName);
// Check if the file is within the base directory
if (strpos($filePath, $baseDirectory) === 0) {
// Proceed with file access
return file_get_contents($filePath);
} else {
throw new Exception("Attempted Directory Traversal Detected");
}
}
Conclusion
Preventing directory traversal attacks is critical for securing web applications from unauthorized access and data breaches. By applying a combination of input validation, allow lists, using built-in functions, employing chroot jails and virtual directories, and adhering to best practices for software maintenance and access control, developers can significantly mitigate the risk of these attacks. Regular monitoring and logging of file access can also aid in early detection of suspicious activities, enabling prompt response to potential security threats. Remember, security is an ongoing process, and staying informed about the latest threats and mitigation techniques is key to protecting your applications and users.