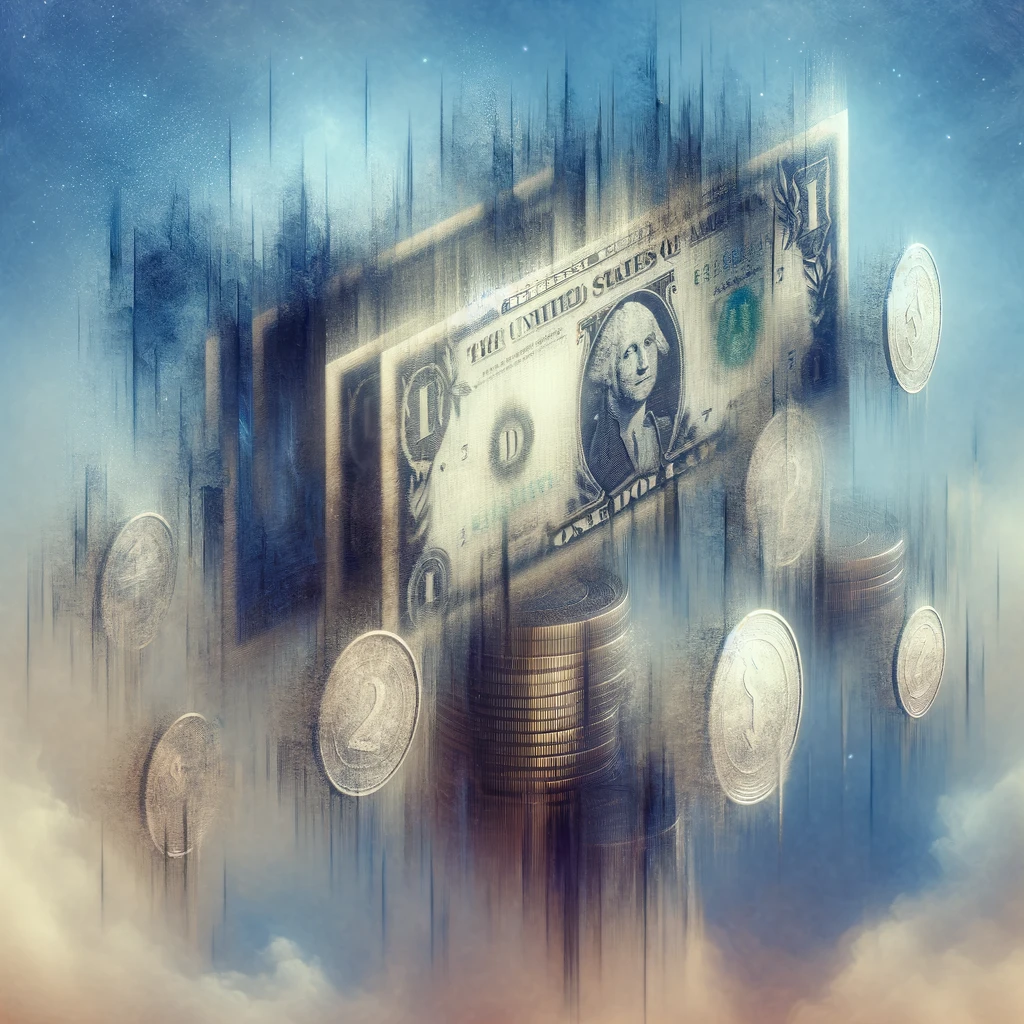
In the world of software security and financial transactions, the precision of data handling is not just a preference, it’s a necessity. One common question that arises in this domain is whether to represent monetary values as floating-point dollar amounts or as integer values in cents. Here, we delve into the reasons why using cents, as opposed to floating-point dollar values, is often the more secure and accurate approach for recording transaction amounts.
The Perils of Floating-Point Arithmetic
Here, I will go over some of the issues that can be encountered when performing floating point arithmetic.
Apparent Rounding Errors
To understand the issue at hand, let’s first dive into the nature of floating-point arithmetic. A floating-point number is a way to represent a real number in computing, allowing for the representation of fractions. However, this comes with a cost: precision. Floating-point numbers are notorious for what appear to be rounding errors but are actually limitations of the underlying implementation.
Consider this simple Python example:
# Floating-point arithmetic example
total = 0.1 + 0.2
print(total) # Often outputs: 0.30000000000000004
This seemingly straightforward sum does not yield the exact result due to the way floating-point numbers are handled in computers. They are stored in binary, and many decimal fractions cannot be represented exactly as binary fractions. This inaccuracy can lead to significant problems in financial transactions where every cent counts.
Truncation of Large Numbers
In this example, we’ll use Python to demonstrate a scenario where floating-point arithmetic leads to a noticeable error due to its limited precision when dealing with very large numbers:
# Example of precision issue with large floating-point numbers
large_number = 1e18 # A large number (10^18)
increment = 1.0 # A small increment
# Adding a small increment to a large floating-point number
new_number = large_number + increment
# Displaying the results
print(f"Original large number: {large_number}") # Outputs: Original large number: 1e+18
print(f"Number after adding 1.0: {new_number}") # Outputs: Number after adding 1.0: 1e+18
In this scenario, 1e18
is a large floating-point number (equivalent to 10^18). When we add a small increment (1.0
) to this large number, we might expect the result to be 1e18 + 1
. However, due to the limitations of floating-point precision, the small increment may not affect the large number as expected, leading to a result that’s unexpectedly the same as the original large number.
Explaining the Issue
Floating-point numbers are represented in a computer using a fixed number of binary digits. The precision of a floating-point number is limited by the number of digits it can use. When dealing with very large numbers, the precision available for the fractional part becomes insufficient to represent small changes accurately. This is why adding 1.0
to a very large floating-point number might not change the value in the way you would expect with real-world arithmetic.
Integer Arithmetic: A Safer Bet
Now, let’s look at the alternative: using integer values to represent money in cents. Here, each transaction amount is recorded as an integer, representing the total number of cents. This method is inherently more precise because integers represent whole numbers exactly, without any rounding errors.
Here’s how the same operation looks using integer values:
# Integer arithmetic example
total_cents = 10 + 20 # Representing 0.10 and 0.20 dollars
print(total_cents) # Outputs: 30
This approach eliminates the risk of rounding errors that can accumulate over transactions and lead to significant discrepancies.
Real-World Implications and Best Practices
The implications of choosing the right data type for financial transactions are far-reaching. Inaccuracies in financial software can lead to legal issues, financial loss, and damage to a company’s reputation.
To avoid these pitfalls, here are some best practices:
Always use integer types for monetary values. This is a common practice in financial software development for handling currencies.
Be aware of the limitations of your programming language. Different languages have different ways of handling numbers, and being aware of these can help you avoid common pitfalls.
Use dedicated libraries when available. Many programming languages offer libraries specifically designed for precise financial calculations. For instance, Python has the
decimal
module, which provides a Decimal datatype for precise arithmetic.Regularly test and audit your software. Ensure your financial calculations are accurate and secure by implementing thorough testing and auditing practices.
Stay informed about the legal requirements in your region. Different countries and industries have regulations regarding financial software and data handling. Ensure your practices are compliant.
Conclusion
In conclusion, when it comes to recording transaction amounts in software, the choice between floating-point numbers and integers can have significant implications. Opting for integers to represent amounts in cents is a safer, more precise method that avoids the pitfalls of floating-point arithmetic. This approach is essential for maintaining the accuracy and integrity of financial data, which is crucial in the high-stakes world of financial transactions.
For further reading on best practices in software security and financial transactions, consider visiting OWASP’s guidelines on secure coding and the IEEE standard for floating-point arithmetic.
In your own projects, always remember: precision in data representation isn’t just about getting the numbers right; it’s about maintaining trust, accuracy, and security in the digital financial world.