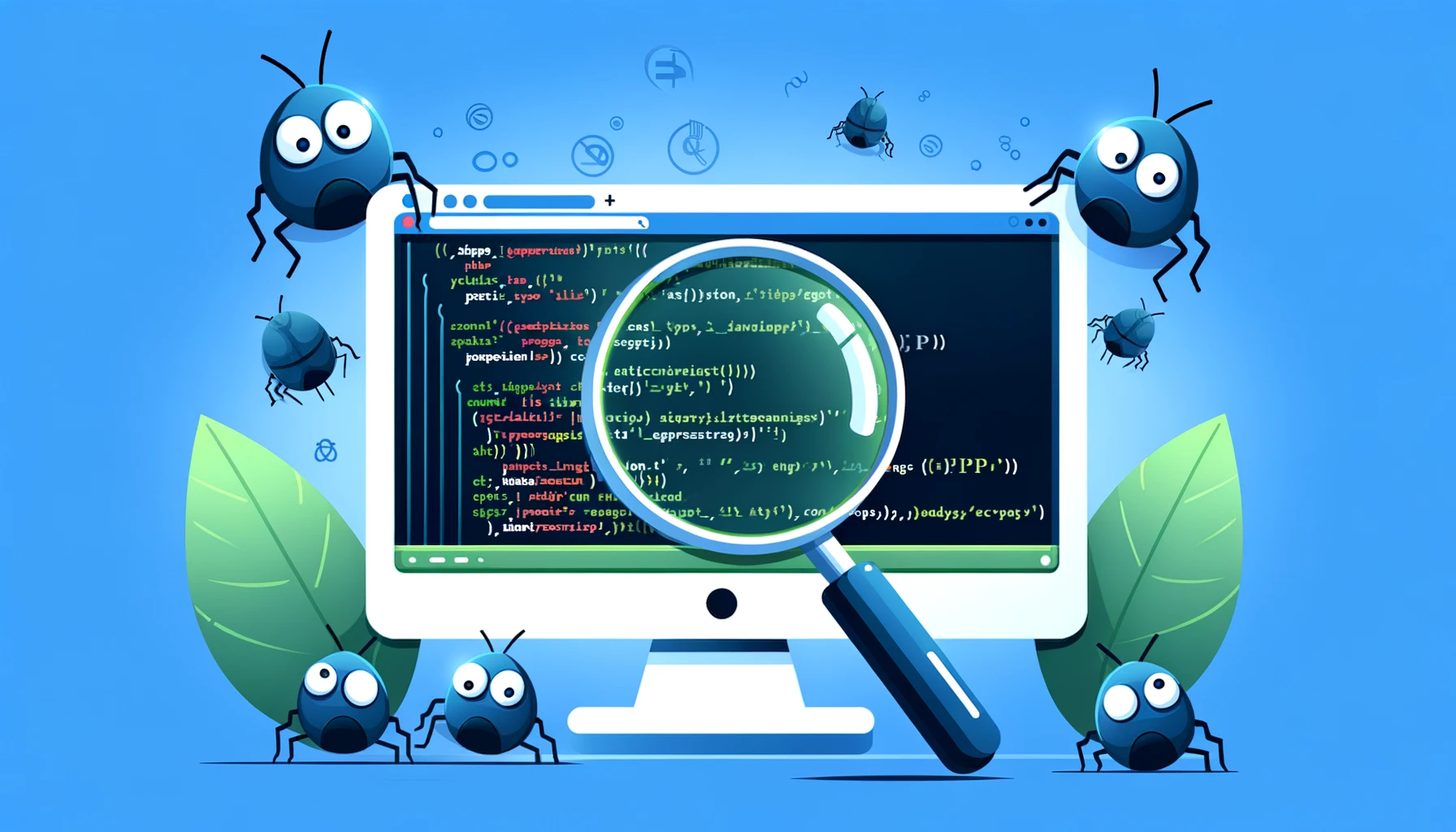
Managing dates in PHP can be surprisingly tricky, even when using libraries like Carbon in Laravel. A common pitfall occurs with the subMonth()
function, which can lead to unexpected results, particularly towards the end of longer months.
Take, for instance, the scenario where you’re manipulating dates and want to find the start of the previous month and the current date is December 31st. You might expect to be able to subtract a month to get to November, and then use startOfMonth()
to get the start of the month. However, this approach will fail, as we’ll see in the next section.
The December Dilemma
Let’s delve into this with a practical example. You’re working with a date, say December 31st, and you want to subtract a month from it and then get the start of the month. Using Carbon, you might write:
$date = new Carbon('2022-12-31');
echo $date->subMonth()->startOfMonth()->toDateString(); // Outputs: "2022-12-01"
Surprisingly, the output is December 1st, not November 1st, a whole month off!
However if we run the same operations in a different order, we get the expected result:
$date = new Carbon('2022-12-31');
echo $date->startOfMonth()->subMonth()->toDateString(); // Outputs: "2022-11-01"
Here, the output is November 1st, as expected. To understand why this occurs we need to see what subMonth()
does on its own.
$date = new Carbon('2022-12-31');
echo $date->subMonth()->toDateString(); // Outputs: "2022-12-01"
Surprisingly, the output is December 1st (the same month that we started with). This occurs because subMonth()
tries to maintain the day component of the date. Since November has only 30 days, subtracting a month from the 31st day results in an overflow, which Carbon resolves by wrapping back to the start of the month. If you first do the startOfMonth()
operation, you’re working with the first day of the month, so there’s no overflow since every month has at least twenty-eight days.
Other solutions
While this approach is functional, it alters the day component, which might not be desirable in all cases. A more versatile solution is to use subMonthNoOverflow()
. This function subtracts a month but prevents the day component from overflowing, ensuring a more intuitive result:
$date = new Carbon('2022-12-31');
echo $date->subMonthNoOverflow()->toDateString(); // Outputs: "2022-11-30"
Here, the output is November 30th, as one would logically expect.
Conclusion
When working with dates in PHP, particularly with libraries like Carbon in Laravel, it’s crucial to be mindful of how different functions handle edge cases. The subMonth()
function, while useful, can lead to unexpected results due to its handling of month-end overflow. To ensure more accurate date calculations, consider using startOfMonth()
for resetting to the month’s start or subMonthNoOverflow()
for a direct approach that respects month boundaries. Understanding these subtleties can significantly enhance the reliability and clarity of your date-related code in PHP.