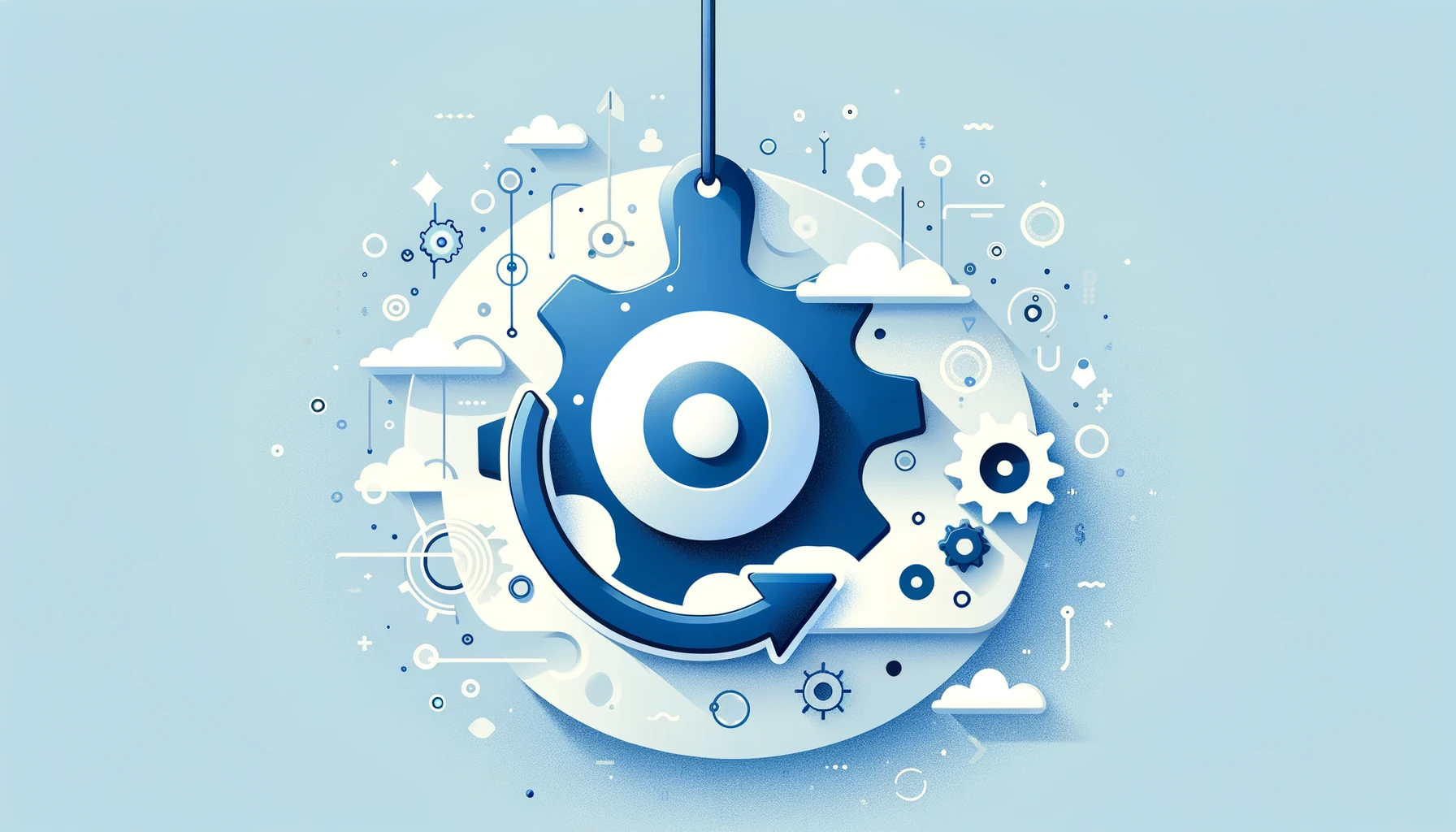
React, a popular JavaScript library for building user interfaces, is renowned for its efficiency and speed, thanks to the virtual DOM. In many cases, especially in smaller applications, React’s default behavior is sufficient for maintaining smooth performance. However, as applications grow in complexity, performance bottlenecks can emerge, particularly during rendering. This is where React hooks like useMemo
and useCallback
come into play, offering fine-tuned control over the render behavior of your components.
Understanding the Need for Optimization
Before diving into the specifics of these hooks, it’s essential to understand why optimization is sometimes necessary in React. React’s re-rendering process, though efficient, can become costly in complex applications. When a component’s state or props change, React re-renders the component and its child components. This isn’t typically an issue for simple UIs, but for complex applications with numerous components and heavy computations, unnecessary re-renders can lead to noticeable performance issues.
When to Use These Hooks
While useMemo
and useCallback
can significantly improve performance, they come with overhead and should be used judiciously. Overuse can lead to more complex code and, ironically, performance issues. The rule of thumb is to profile your application first, identify bottlenecks, and then apply these hooks where they make a tangible difference.
Enter useMemo
and useCallback
useMemo
and useCallback
are hooks that help optimize performance by memoizing values and functions, respectively. This means they remember the outputs of computations or the instances of functions, so they don’t have to be recalculated or redefined on every render.
useMemo
: Preserving Computation Results
useMemo
is ideal for scenarios where you have expensive calculations that don’t need to be recalculated every time the component renders. Consider a component that calculates the Fibonacci sequence based on an input number. This calculation can be computationally intensive, and using useMemo
can prevent unnecessary recalculations.
const fibonacci = (n) => {
// ...computationally intensive calculation...
};
const MyComponent = ({ num }) => {
const fibValue = useMemo(() => fibonacci(num), [num]);
return <div>{fibValue}</div>;
};
In this example, fibonacci(num)
is only recalculated when num
changes, thus avoiding unnecessary computations on re-renders.
useCallback
: Memoizing Functions
In React, components often pass functions as props to their child components. This is a common pattern, especially for handling events or callbacks. However, there’s a subtle issue that can arise in this pattern related to how React determines whether a component needs to re-render.
When a parent component renders, it typically creates a new instance of any functions it passes down as props. Even if the function’s code hasn’t changed, from React’s perspective, this is a new function - because functions are objects in JavaScript, and each instance is unique. Here’s where useCallback
becomes crucial.
How useCallback
works
useCallback
is a hook that memoizes function instances. This means it keeps a reference to a function across renders, as long as its dependencies haven’t changed. By wrapping a function in useCallback
, you’re telling React to reuse the same function instance unless specific inputs (the dependencies) change.
Preventing Unnecessary Child Component Rerenders
Many child components in React are optimized to reduce unnecessary renders. One common way of doing this is using React’s React.memo
higher-order component. React.memo
will only re-render a component if its props have changed. However, if a parent component passes down a new function instance on every render (even if the function does the same thing), React.memo
will see this as a change and re-render the child component.
Here’s where useCallback
plays a pivotal role. By ensuring that the function instance remains the same across renders, useCallback
prevents React.memo
and similar optimizations in child components from mistaking a new function instance for a change in props. This effectively reduces unnecessary re-renders, enhancing performance, especially in complex component trees.
const ChildComponent = React.memo(({ onClick }) => {
// ...child component...
});
const ParentComponent = () => {
const handleClick = useCallback(() => {
console.log('Clicked!');
}, []);
return <ChildComponent onClick={handleClick} />;
};
Here, React.memo
is used to optimize ChildComponent
, and useCallback
ensures that handleClick
maintains the same reference across renders unless dependencies change.
Conclusion
React’s performance is generally excellent, but certain scenarios necessitate a deeper understanding and application of optimization techniques. useMemo
and useCallback
are powerful hooks that, when used appropriately, can significantly enhance the render performance of your React applications. Remember, the key is to use these tools wisely and only in situations where they provide a real benefit. Keep profiling your application, understand its performance characteristics, and apply these hooks to create a smooth, efficient user experience.