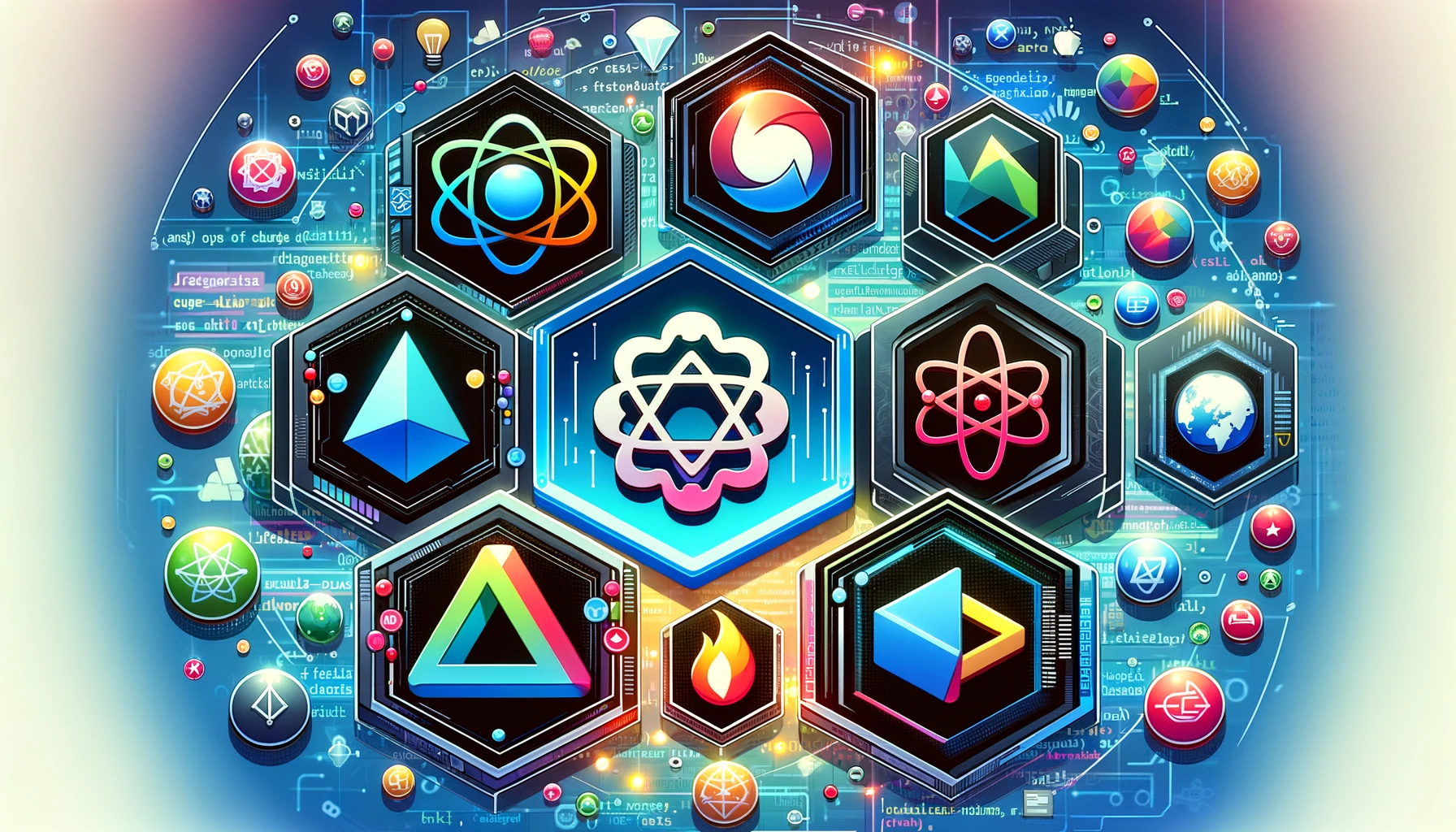
In the evolving landscape of web development, choosing the right backend framework can be a critical decision that impacts not just the functionality of an application but also its security. Two prominent players in this domain are NestJS and Express. While Express has been a long-time favorite for Node.js developers, NestJS is rapidly gaining popularity for its out-of-the-box architecture and scalability features. This comparative analysis aims to delve into the nuances of both frameworks, focusing on their security aspects, ease of use, and suitability for various project sizes.
The Essence of Express: Simplicity and Flexibility
Express.js, often referred to simply as Express, is an unopinionated, minimalist web framework for Node.js. It’s known for its simplicity and flexibility, allowing developers to write their server-side logic in JavaScript. A key feature of Express is its use of middleware, which are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle.
Example: Basic Express Server
const express = require("express");
const app = express();
app.get("/", function (req, res) {
res.send("Hello World!");
});
app.listen(3000, function () {
console.log("Example app listening on port 3000!");
});
Express does not enforce any specific architecture, making it very flexible but also putting the onus of structure and security entirely on the developer. This flexibility can be a double-edged sword, especially in large-scale applications where consistency and best practices in code structure are crucial.
NestJS: A Blend of Efficiency and Structure
NestJS, on the other hand, is a framework for building efficient, reliable, and scalable server-side applications. It is built on top of Express (but can also work with Fastify) and adds an extra layer of abstraction with its out-of-the-box application architecture. NestJS is heavily inspired by Angular, adopting a modular approach and making use of decorators, which can make the learning curve steeper for those unfamiliar with these concepts.
Example: Basic NestJS Server
import { Module } from "@nestjs/common";
import { NestFactory } from "@nestjs/core";
import { AppModule } from "./app.module";
async function bootstrap() {
const app = await NestFactory.create(AppModule);
await app.listen(3000);
}
bootstrap();
One of the standout features of NestJS is its embrace of TypeScript by default, offering strong typing and reducing the likelihood of runtime errors. Additionally, its structure promotes clean code and makes it inherently easier to maintain and scale, especially for large teams and projects.
Database Interactions: ORMs and Beyond
A crucial aspect of web development is how a framework interacts with databases. Both Express and NestJS offer flexibility in choosing database solutions, but their approaches differ, especially when it comes to Object-Relational Mapping (ORM) integration.
Express and Database Integration
Express, true to its minimalistic nature, does not prescribe any specific database or ORM. Developers have the freedom to choose any database – SQL (like PostgreSQL, MySQL) or NoSQL (like MongoDB) – and integrate it using their preferred library or ORM. Common choices include Mongoose for MongoDB, Sequelize for SQL databases, and the more recent Prisma, which supports multiple databases.
Example: Integrating Sequelize with Express
const Sequelize = require("sequelize");
const sequelize = new Sequelize("database", "username", "password", {
host: "localhost",
dialect: "mysql",
});
// Define a model
const User = sequelize.define("user", {
username: Sequelize.STRING,
birthday: Sequelize.DATE,
});
sequelize
.sync()
.then(() =>
User.create({
username: "janedoe",
birthday: new Date(1980, 6, 20),
})
)
.then((jane) => {
console.log(jane.toJSON());
});
This flexibility allows developers to tailor their database interaction precisely to the needs of the application but requires manual setup and configuration.
NestJS: A Structured Approach to ORMs
NestJS, in contrast, provides an integrated approach to database interactions, with first-class support for TypeORM and Sequelize out of the box. It leverages decorators and TypeScript to provide a more structured and maintainable way of handling database operations. NestJS’s modules system organizes the codebase, making it more maintainable, especially in large applications with complex database interactions.
Example: Using TypeORM with NestJS
import { Entity, Column, PrimaryGeneratedColumn } from "typeorm";
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number;
@Column()
firstName: string;
@Column()
lastName: string;
@Column()
isActive: boolean;
}
NestJS also supports MikroORM and Prisma, offering developers a wide range of choices. The integrated ORM approach in NestJS aligns with its overall philosophy of providing a structured and scalable framework.
Security Considerations
When it comes to security, both frameworks offer basic protection but have different approaches.
Express: Being minimalistic, Express requires developers to manually implement security measures or integrate third-party libraries. Common practices include using helmet for securing HTTP headers, implementing rate limiting, and ensuring proper error handling. However, the responsibility of setting up and maintaining these security measures lies with the developer.
NestJS: Thanks to its architecture, NestJS provides a more guided approach to security. It integrates well with Passport for authentication, and its dependency injection system can enhance security by reducing risks associated with middleware dependency conflicts. NestJS’s use of TypeScript also adds a layer of security by catching errors at compile time.
Suitability for Different Project Sizes
Express is ideal for small to medium-sized projects or for developers who prefer complete control over the architecture and flow of their application. Its minimalistic nature makes it easy to set up and deploy, making it a favorite for quick prototypes and small applications.
NestJS shines in larger, more complex applications. Its structured approach is beneficial for long-term maintenance and scalability. For teams familiar with Angular or TypeScript, NestJS will feel right at home.
Conclusion
In summary, both NestJS and Express have their own set of strengths and ideal use cases. Express, with its minimalistic and unopinionated nature, offers greater flexibility but requires more effort in maintaining structure and security in larger applications. NestJS, with its Angular-inspired architecture and TypeScript integration, provides a robust framework that encourages best practices and scalability, making it a suitable choice for larger, more complex applications.
For further reading and a deeper dive into these frameworks, the official documentation of Express and NestJS can be excellent resources. Additionally, exploring community forums and tutorials can provide practical insights and best practices shared by experienced developers in the field.