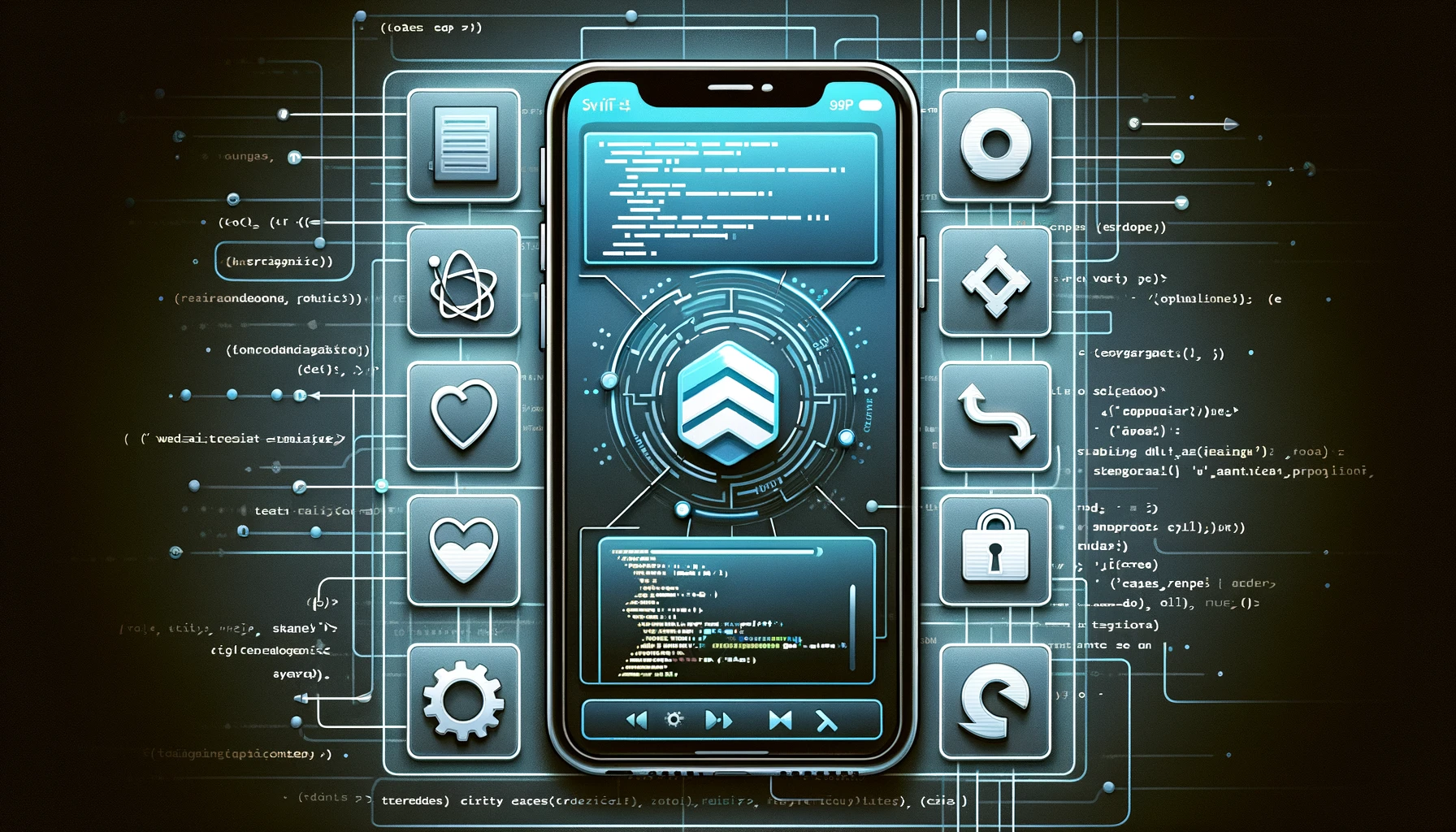
In the world of iOS app development, ensuring smooth, glitch-free performance is a top priority. However, one of the trickier issues that developers often grapple with is race conditions. These occur when the timing or sequence of events impacts your program’s correctness, leading to unpredictable and erratic behavior. This post delves into the nuances of identifying and resolving race conditions during code reviews, focusing on two critical areas: the use of serial queues and the handling of UI elements from background threads.
Understanding Race Conditions in iOS
Race conditions in iOS development typically arise when multiple threads access shared resources without proper synchronization. This can lead to corrupted data or crashes, significantly degrading the user experience. They’re often subtle and hard to replicate, which makes them a challenging issue to address during development and code review.
Serial Queues to the Rescue
One effective way to prevent race conditions is through the use of serial queues. In iOS, DispatchQueue
is a powerful tool for managing concurrent operations. You can create a serial queue to ensure tasks are completed one at a time, thus avoiding simultaneous access to shared resources. The DispatchQueue.global()
method accesses a global concurrent queue, while the DispatchQueue(label:)
method creates a serial queue by default that you can use to synchronize access to shared resources. (Note that you can also create a concurrent queue by passing the concurrent
parameter to the DispatchQueue(label:concurrent:)
method.) See more info on Apple’s documentation.
Consider the following example:
let serialQueue = DispatchQueue(label: "com.yourapp.serialQueue")
func updateSharedResource() {
serialQueue.async {
// Update shared resource here
}
}
func readSharedResource() {
serialQueue.async {
// Read shared resource here
}
}
In this snippet, both the updateSharedResource
and readSharedResource
functions use the same serial queue. This guarantees that even if these functions are called from different threads, the operations on the shared resource will not overlap, effectively preventing race conditions.
UI Updates on Main Thread
Another common source of race conditions in iOS apps involves UI updates. The golden rule is that all UI updates must be performed on the main thread. Violating this rule can lead to unpredictable UI states and crashes. However, it’s not uncommon for developers to inadvertently update UI elements from background threads.
During code review, pay special attention to asynchronous code blocks. Ensure that any code that touches the UI is dispatched back to the main thread. Here’s an example to illustrate this point:
DispatchQueue.global().async {
// Perform some background task
let data = fetchData()
DispatchQueue.main.async {
// Update UI with fetched data
self.tableView.reloadData()
}
}
func fetchData() -> [Data] {
// Fetch data from a source
}
In this example, fetchData
is called on a global (concurrent) queue, but the subsequent UI update (tableView.reloadData()
) is correctly dispatched on the main thread.
Tools and Techniques for Detecting Race Conditions
Detecting race conditions can be tricky, but there are tools and techniques that can help during the code review process:
- Code Analysis: Thoroughly review code for potential race conditions, focusing on areas where shared resources are accessed by multiple threads.
- Thread Sanitizer: Xcode’s Thread Sanitizer is an invaluable tool that can help detect data races during runtime.
- Unit Tests: Writing unit tests that simulate concurrent access can sometimes reveal race conditions.
- Logging and Monitoring: Implement detailed logging around shared resources to monitor and identify potential race conditions.
Closing Thoughts
Preventing race conditions in iOS apps requires a keen eye during code review. By utilizing serial queues for synchronized access to shared resources and ensuring UI updates are dispatched on the main thread, you can significantly reduce the risk of these elusive bugs. Always remember to leverage tools like Thread Sanitizer and unit tests to assist in identifying these issues.
For further reading on iOS concurrency and best practices, the Apple Developer Documentation provides comprehensive guides and references. Understanding and mastering these concepts is key to developing robust, reliable iOS applications.