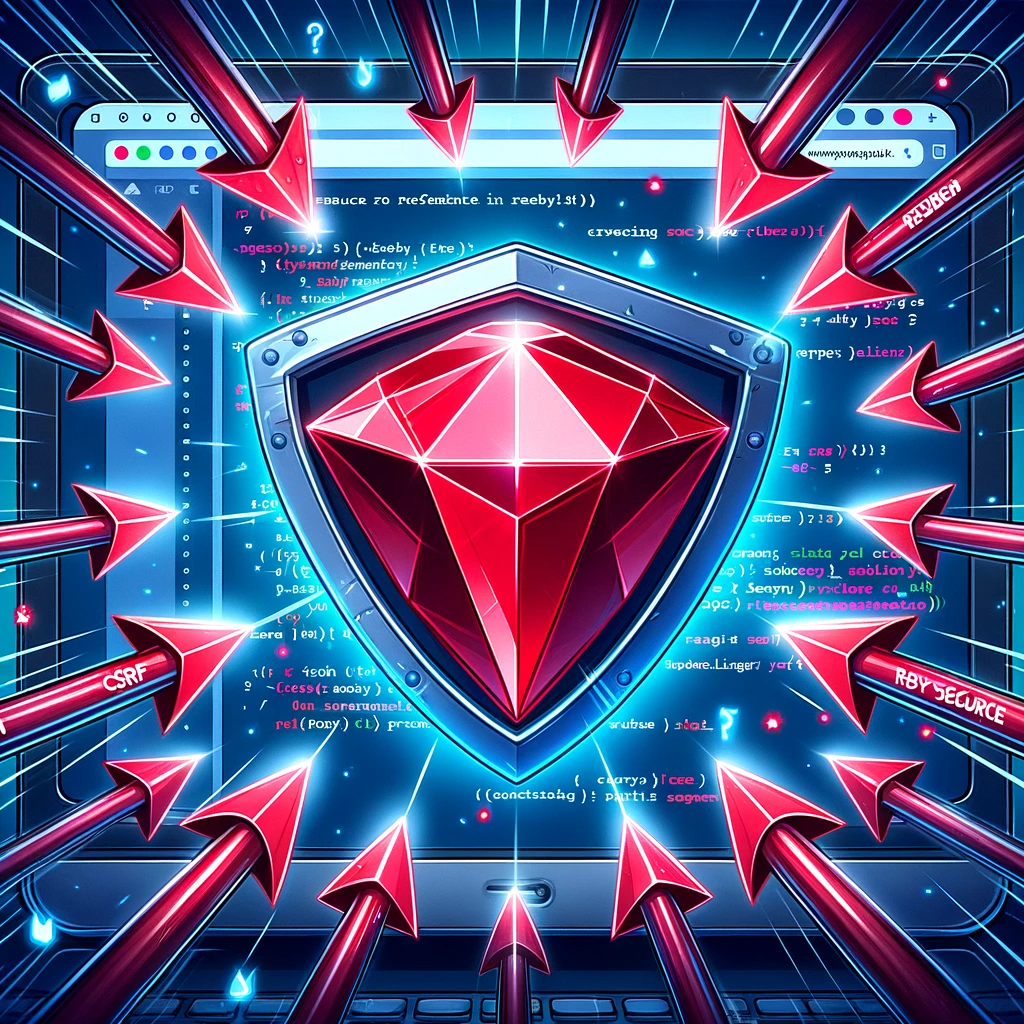
Cross-Site Request Forgery (CSRF) is a security vulnerability that allows attackers to trick users into performing actions they don’t intend to. Recognized as CWE-352, this weakness can have significant implications in web applications. As Ruby developers, understanding and mitigating this risk is crucial. In this post, we’ll delve into identifying and remediating CSRF vulnerabilities in Ruby applications, using practical examples and relevant code snippets.
Understanding CSRF in Ruby
Before we jump into the technical details, let’s understand the nature of CSRF attacks. In simple terms, CSRF exploits the trust that a web application has in the user’s browser. Imagine a scenario where a user is logged into a banking application. An attacker could craft a malicious link or form on another site that, when clicked by the user, performs an action on the banking site using the user’s credentials.
Ruby web applications, particularly those built with frameworks like Ruby on Rails, are not immune to this threat. Fortunately, Rails does provide built-in mechanisms to protect against CSRF. However, custom Ruby applications or improperly configured Rails apps can still be vulnerable.
Identifying CSRF Vulnerabilities
Code Review Techniques
Absence of Authenticity Token: Rails uses an authenticity token to prevent CSRF attacks. This token ensures the request the backend receives came from the expected, first-party site rather than some third-party site controlled by an attacker. In your code review, check for the presence of
csrf_meta_tags
in the application’s layouts. This tag generates a random token for each session and validates it with each form submission.# app/views/layouts/application.html.erb <%= csrf_meta_tags %>
Unsafe HTTP Methods: Ensure that state-changing operations (like creating or deleting resources) are performed using HTTP methods like POST, PUT, DELETE, and PATCH. GET requests should be idempotent and free from side effects.
Skipping Verification: Look for instances where
skip_before_action :verify_authenticity_token
is used. This bypasses CSRF protection and should be avoided unless absolutely necessary and properly secured.
Debugging Techniques
Manual Testing: Manually test your application by disabling cookies. If actions that should require authentication still work, that’s a red flag.
Logging: Implement logging of failed CSRF checks. This can help in identifying unexpected behavior or attacks.
# config/initializers/csrf_logger.rb ActiveSupport::Notifications.subscribe('verified_request_failed.action_controller') do |name, start, finish, id, payload| Rails.logger.warn("CSRF verification failed: #{payload[:reason]}") end
Remediation Techniques
Implementing CSRF Tokens
If your Ruby application lacks CSRF protection, the first step is to implement authenticity tokens. In Rails, this is handled automatically, but it’s good practice to understand how it works.
Add Authenticity Token to Forms: Ensure all forms include the authenticity token.
# In your form views <%= hidden_field_tag :authenticity_token, form_authenticity_token %>
Validate Token in Controllers: The application should validate the token with each state-changing request.
class ApplicationController < ActionController::Base protect_from_forgery with: :exception end
Custom Ruby Applications
For custom Ruby applications not using Rails, you’ll need to manually implement CSRF protection.
- Generate a Session Token: Create a secure, random token when the user session starts.
- Store the Token: Save the token in the user’s session and include it in forms and AJAX requests.
- Verify the Token: On receiving a request, compare the token from the request with the one stored in the session.
# Example of generating a token
require 'securerandom'
def generate_csrf_token
session[:csrf_token] ||= SecureRandom.hex(64)
end
# Example of verifying the token
def verify_csrf_token
unless session[:csrf_token] && session[:csrf_token] == params[:csrf_token]
halt 403, 'CSRF token verification failed'
end
end
Enhancing Security with SameSite Cookies
Modern browsers support the SameSite cookie attribute, which helps mitigate CSRF attacks. By setting the SameSite attribute to Lax
or Strict
, you instruct the browser to only send cookies in a first-party context.
In Rails, you can configure this in your application:
# config/initializers/session_store.rb
Rails.application.config.session_store :cookie_store, key: '_your_app_session', same_site: :strict
Regular Auditing and Testing
Beyond implementing these technical solutions, regular code audits and security testing are vital. Automated tools like Brakeman can scan your Ruby code for security vulnerabilities. Additionally, include CSRF attack scenarios in your penetration testing plans.
Conclusion
In conclusion, protecting you Ruby application from CSRF attacks is essential. By understanding how to identify vulnerabilities through code review and debugging, and implementing effective remediation techniques, you can significantly enhance your application’s security posture.
Remember, security is an ongoing process, not a one-time setup. Regularly update your practices and stay informed about the latest security trends and threats.
For more information and best practices in Ruby application security, refer to the official Ruby on Rails Security Guide and the OWASP CSRF Prevention Cheat Sheet.
Stay secure and keep coding!