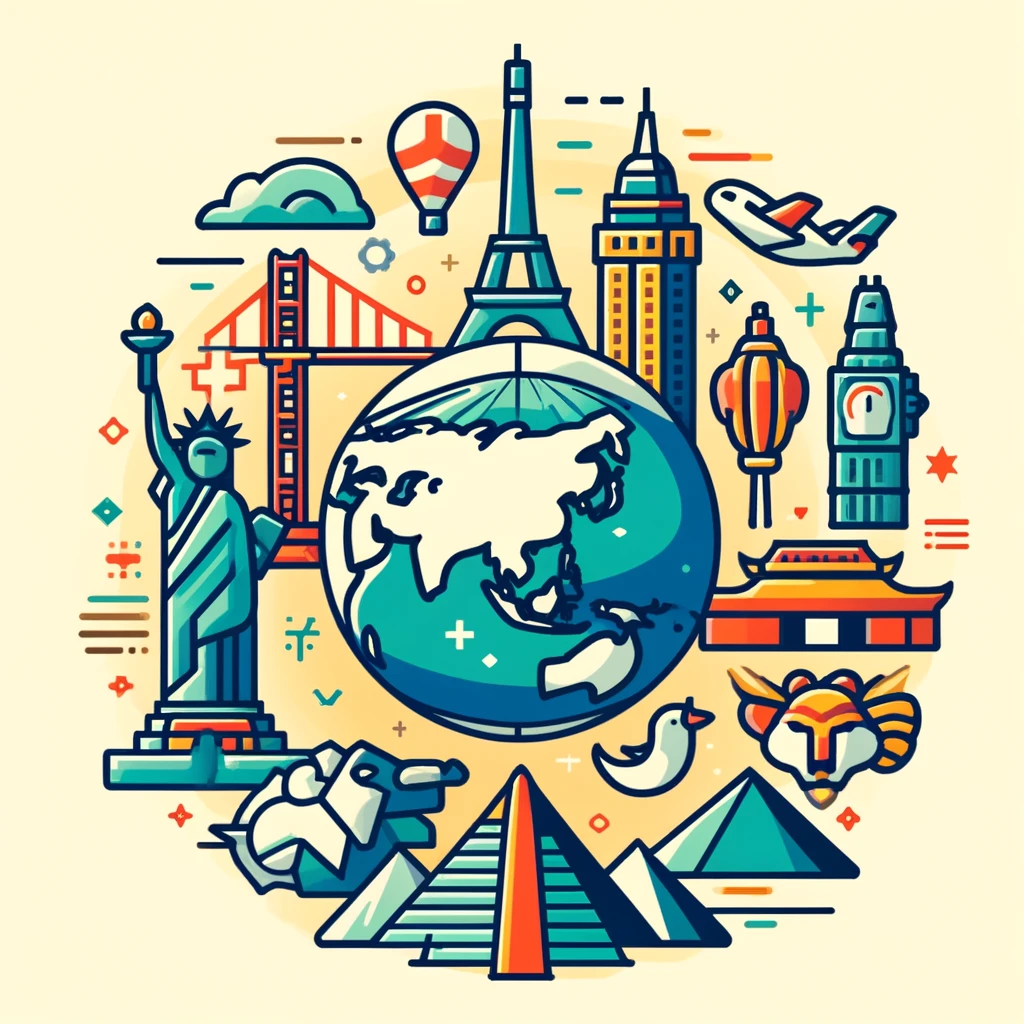
In the rapidly expanding digital world, the ability to adapt software for global markets is more crucial than ever. Internationalization (often abbreviated as i18n because there are eighteen characters between the “i” and the “n”) stands at the forefront of this challenge, enabling developers to create flexible applications that can be easily localized for users in different countries. This post dives into the essence of i18n, shedding light on its significance, key concepts, and practical steps to integrate it into your development process.
Understanding Internationalization
At its core, internationalization is the process of designing and building your software application in a way that makes it possible to adapt to various languages and regions with minimal engineering changes. It’s a proactive measure that ensures your software can reach a wider audience, breaking the barriers of language and cultural differences.
The primary goal of i18n is to abstract all locale-specific elements from your application, such as date formats, currencies, and text direction, making it straightforward to adapt or localize your software for a specific audience. This approach not only amplifies your market reach but also streamlines the development process, as it separates the concerns of functionality and localization.
Key Concepts and Strategies
1. Locale Identification
A locale represents a user’s language, country, and any special variant preferences that the user wants to see in their user interface. Typically, a locale is identified by a code, such as en-US
for English as used in the United States or fr-CA
for French as used in Canada. Recognizing and using these codes effectively is the first step in internationalizing your software.
2. Text and Content Externalization
Hard-coding text strings in your application makes it difficult to translate and adapt content for different markets. Internationalization involves externalizing text strings and other content into separate files or databases, which can then be easily translated without altering the codebase. Libraries such as GNU gettext or frameworks like i18next for JavaScript applications are popular tools that help in managing these externalized strings.
GNU gettext in Python:
import gettext
gettext.install('myapp', localedir='/path/to/my/locale/directory')
_ = gettext.gettext
print(_("Hello, world!"))
i18next in JavaScript:
import i18next from 'i18next';
i18next.init({
lng: 'en', // default language
resources: {
en: {
translation: {
"hello_world": "Hello, World!"
}
},
es: {
translation: {
"hello_world": "¡Hola, Mundo!"
}
}
}
});
console.log(i18next.t('hello_world')); // Output: Hello, World!
3. Date, Time, and Number Formatting
Different regions have different conventions for displaying dates, times, and numbers. For example, while the US uses month/day/year format for dates, many European countries use day/month/year. Internationalization requires abstracting these formats so that they can be localized according to the user’s locale settings. Java’s DateFormat
and JavaScript’s Intl
object are examples of how programming languages help handle these variations.
Java’s DateFormat
example:
import java.text.DateFormat;
import java.util.Date;
import java.util.Locale;
public class DateFormatExample {
public static void main(String[] args) {
// Create a date instance for the current date and time
Date currentDate = new Date();
// Format the date for the US locale
DateFormat usFormat = DateFormat.getDateInstance(DateFormat.LONG, Locale.US);
System.out.println("US format: " + usFormat.format(currentDate));
// Format the same date for the German locale
DateFormat deFormat = DateFormat.getDateInstance(DateFormat.LONG, Locale.GERMANY);
System.out.println("German format: " + deFormat.format(currentDate));
}
}
Javascript’s Intl
example:
// Format a date for the US locale
const usDate = new Intl.DateTimeFormat('en-US', {
year: 'numeric',
month: 'long',
day: 'numeric'
}).format(new Date());
console.log("US format: " + usDate);
// Format the same date for the German locale
const deDate = new Intl.DateTimeFormat('de-DE', {
year: 'numeric',
month: 'long',
day: 'numeric'
}).format(new Date());
console.log("German format: " + deDate);
In the above examples, passing undefined
as instead of a locale will default to the user’s configured locale.
4. Handling Plural Forms and Gender
Languages have various rules for pluralization and gender, which can affect how sentences are constructed. Software internationalization must account for these differences, ensuring that plural forms and gender-specific references are correctly handled in each language. This often involves creating multiple versions of a sentence or using library support for pluralization and gender in your strings.
plural in i18next:
i18next.init({
lng: 'en', // Setting default language to English
resources: {
en: {
translation: {
key: "You have {{count}} unread message",
key_plural: "You have {{count}} unread messages"
}
},
ru: { // Example with Russian, which has more complex pluralization rules
translation: {
key_0: "У вас {{count}} непрочитанное сообщение",
key_1: "У вас {{count}} непрочитанных сообщения",
key_2: "У вас {{count}} непрочитанных сообщений"
}
}
}
});
// Example usage:
console.log(i18next.t('key', { count: 1 })); // English, 1 message
console.log(i18next.t('key', { count: 3 })); // English, multiple messages
console.log(i18next.changeLanguage('ru')); // Switching to Russian
console.log(i18next.t('key', { count: 1 })); // Russian, 1 message
console.log(i18next.t('key', { count: 3 })); // Russian, 2-4 messages
console.log(i18next.t('key', { count: 5 })); // Russian, 5+ messages
gender in i18next:
i18next.init({
resources: {
en: {
translation: {
greeting_male: "Welcome, Mr. {{name}}!",
greeting_female: "Welcome, Ms. {{name}}!",
greeting_other: "Welcome, {{name}}!"
}
}
}
});
// Example usage:
console.log(i18next.t('greeting', { context: 'male', name: 'John' })); // Male context
console.log(i18next.t('greeting', { context: 'female', name: 'Jane' })); // Female context
console.log(i18next.t('greeting', { context: 'other', name: 'Alex' })); // Not disclosed/other context
Practical Steps to Implement Internationalization
- Start Early: Integrating i18n at the beginning of your development process is much easier and cost-effective than retrofitting it into an existing codebase.
- Use Unicode: Adopt Unicode (UTF-8) as your encoding standard to support a wide range of characters from different languages.
- Leverage Libraries and Frameworks: Take advantage of i18n libraries and frameworks compatible with your development stack. They provide the necessary functions to handle locale detection, string interpolation, and formatting issues.
- Externalize Resources: Move all localizable resources out of your code. This includes strings, date formats, images, and any content that varies by locale.
- Test Thoroughly: Testing your application with various locales is essential to ensure that your i18n efforts are successful. Consider both functional testing and user interface testing to cover all aspects of localization.
- Plan for Right-to-Left (RTL) Languages: If your target audience includes speakers of RTL languages like Arabic or Hebrew, ensure your design and layout can adapt accordingly.
Conclusion
Internationalization is a fundamental step in preparing your software for a global audience. By adhering to the principles of i18n, developers can ensure their applications are accessible, user-friendly, and ready for localization into any language or region. This not only enhances user experience but also opens up new markets and opportunities for growth. As the digital world continues to become more interconnected, the importance of internationalization in software development cannot be overstated. With the right approach and tools, your software can transcend linguistic and cultural barriers, reaching users worldwide.