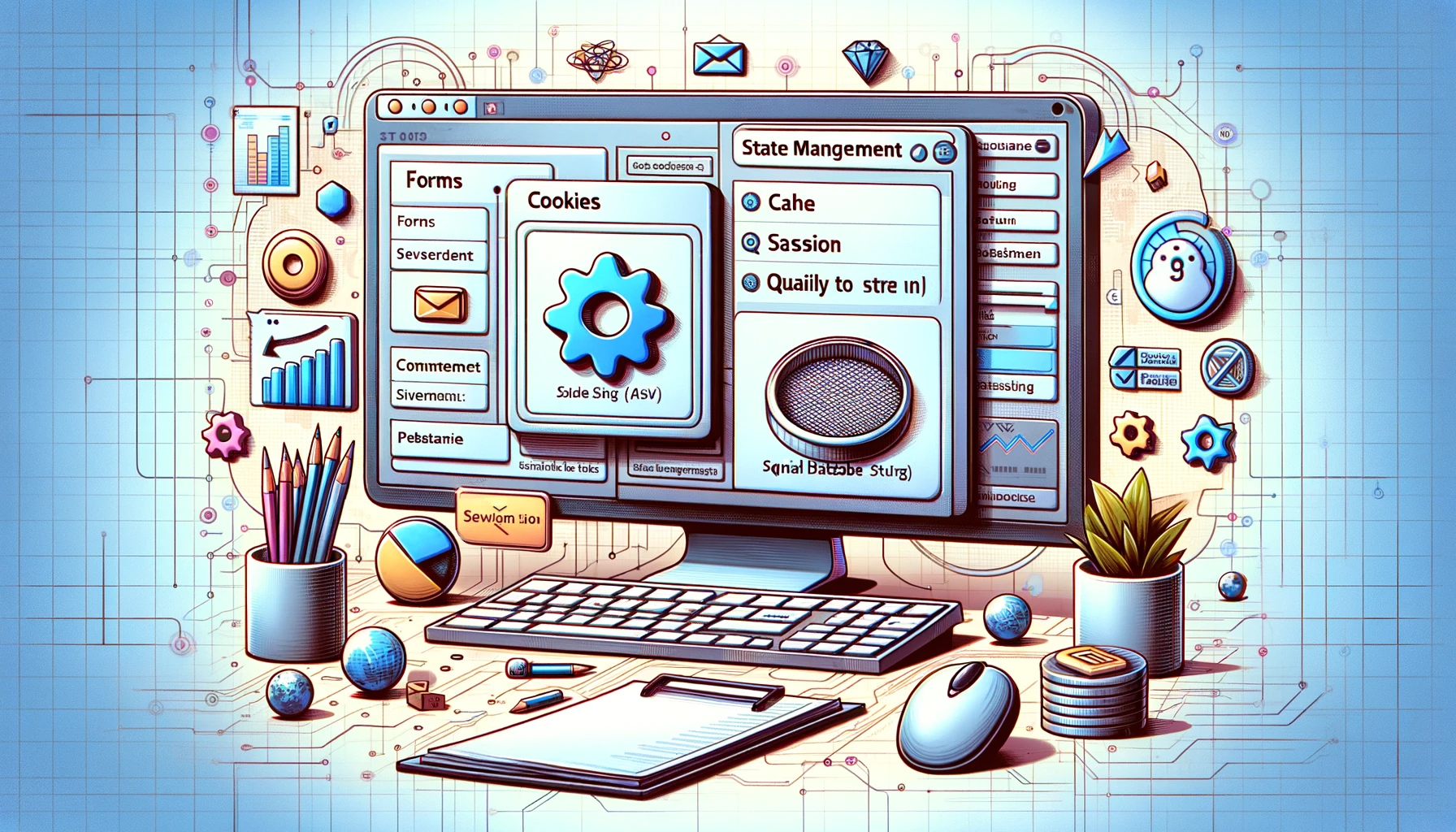
When developing web applications with ASP.NET, state management is a critical aspect that determines the efficiency, scalability, and security of the application. Traditionally, ASP.NET developers have leaned heavily on Session and ViewState for state management. However, with evolving application architectures and user demands, it’s time to reassess their usage. This blog post aims to shed light on state management in ASP.NET, emphasizing why we should consider alternatives to Session and ViewState, except when absolutely necessary.
Understanding State Management in ASP.NET
State management in web applications is fundamentally about maintaining data (or state) across multiple requests. In the context of ASP.NET, there are various ways to manage state:
- Client-Side State Management: Using mechanisms like Cookies and Hidden Fields.
- Server-Side State Management: Including Session State, Application State, and Database Storage.
The choice of state management strategy impacts performance, scalability, and the user experience. Two popular mechanisms, Session State and ViewState, have been widely used but come with their set of drawbacks.
The Drawbacks of Session State and ViewState
Session State
Session State stores data on the server side and associates it with a unique session identifier for each user. Here’s why relying heavily on Session State might not be the best approach:
- Memory Overhead: Each user’s session consumes server memory, which can be a problem for applications with a large number of concurrent users.
- Scalability Issues: In a web farm scenario, managing session state across multiple servers can be complex and requires additional configurations, such as a state server or SQL Server to store session data.
- Security Concerns: If not securely implemented, session hijacking can be a risk.
ViewState
ViewState, on the other hand, maintains state on the client side by storing data in a hidden field on the page. While it’s useful for preserving data across postbacks, it has its pitfalls:
- Increased Page Load: ViewState data is sent back and forth between the client and server, increasing page load times, especially for data-heavy pages.
- Security Risks: ViewState can be a vector for attacks if not properly encrypted and validated.
- Limited to Page Scope: ViewState is only available to the page it belongs to, limiting its utility for application-wide state management.
Alternatives to Session and ViewState
Considering these limitations, let’s explore some effective alternatives for state management in ASP.NET applications.
1. Cookies
Cookies are a client-side state management technique that store small amounts of data on the user’s browser. They are ideal for storing user preferences or tracking user sessions without overloading the server. However, they are limited in size and are vulnerable to manipulation and theft, necessitating careful handling of sensitive data.
// Setting a cookie in ASP.NET
HttpCookie userInfo = new HttpCookie("userInfo");
userInfo["UserName"] = "JohnDoe";
userInfo["UserColorPref"] = "Blue";
userInfo.Expires = DateTime.Now.AddDays(30);
Response.Cookies.Add(userInfo);
2. Cache
Caching can significantly improve the performance of your application by temporarily storing frequently accessed data in memory. ASP.NET Cache is more flexible and efficient than Application State, as it allows for expiration policies and dependencies.
// Using Cache in ASP.NET
Cache["UserData"] = userObject;
3. Query Strings
Query strings can be used to pass small amounts of data between pages. They are appended to the URL and can be easily accessed across different pages. However, they are visible to the user and have size limitations, making them unsuitable for sensitive or large data.
// Reading a query string in ASP.NET
string userId = Request.QueryString["UserId"];
4. Local Storage
For modern web applications, HTML5 Local Storage provides a way to store data on the client’s browser similar to cookies but with a much larger capacity. It’s ideal for storing non-sensitive data that needs to persist across sessions.
// Setting data in Local Storage
localStorage.setItem("userName", "JohnDoe");
One caveat is that some browsers have a “private mode” that limits the functionality of localStorage
.
5. Database Storage
For complex applications with a need for large, secure, and persistent data storage, using a database is the most reliable method. Data can be retrieved and stored efficiently with proper database design and querying techniques.
When to Use Session and ViewState
Despite their limitations, there are scenarios where Session and ViewState are still appropriate:
- Session State: Use for short-lived data that is specific to a single user and does not require persistence after the session ends. For instance, temporary data in multi-page forms.
- ViewState: Suitable for maintaining state in server controls between postbacks. It’s convenient for data that is not sensitive and does not need to be shared across different pages.
Best Practices for State Management in ASP.NET
- Choose the Right Tool for the Job: Assess the needs of your application and choose the state management tool that aligns best with those needs.
- Minimize Server Load: Favor client-side state management to reduce server load and improve application scalability.
- Security: Always encrypt sensitive data and validate input to protect against security vulnerabilities.
- Performance: Consider the impact of state management on application performance and optimize accordingly.
Conclusion
In conclusion, while Session and ViewState have their place in ASP.NET development, it’s essential to consider the broader range of state management options available. By understanding the limitations and appropriate use-cases for each method, developers can create more efficient, scalable, and secure ASP.NET applications. Remember, the key to effective state management is choosing the right tool for the specific needs of your application.
For further reading on state management in ASP.NET, you can refer to the official Microsoft documentation. Additionally, exploring modern ASP.NET frameworks like ASP.NET Core can provide insights into more advanced state management strategies.