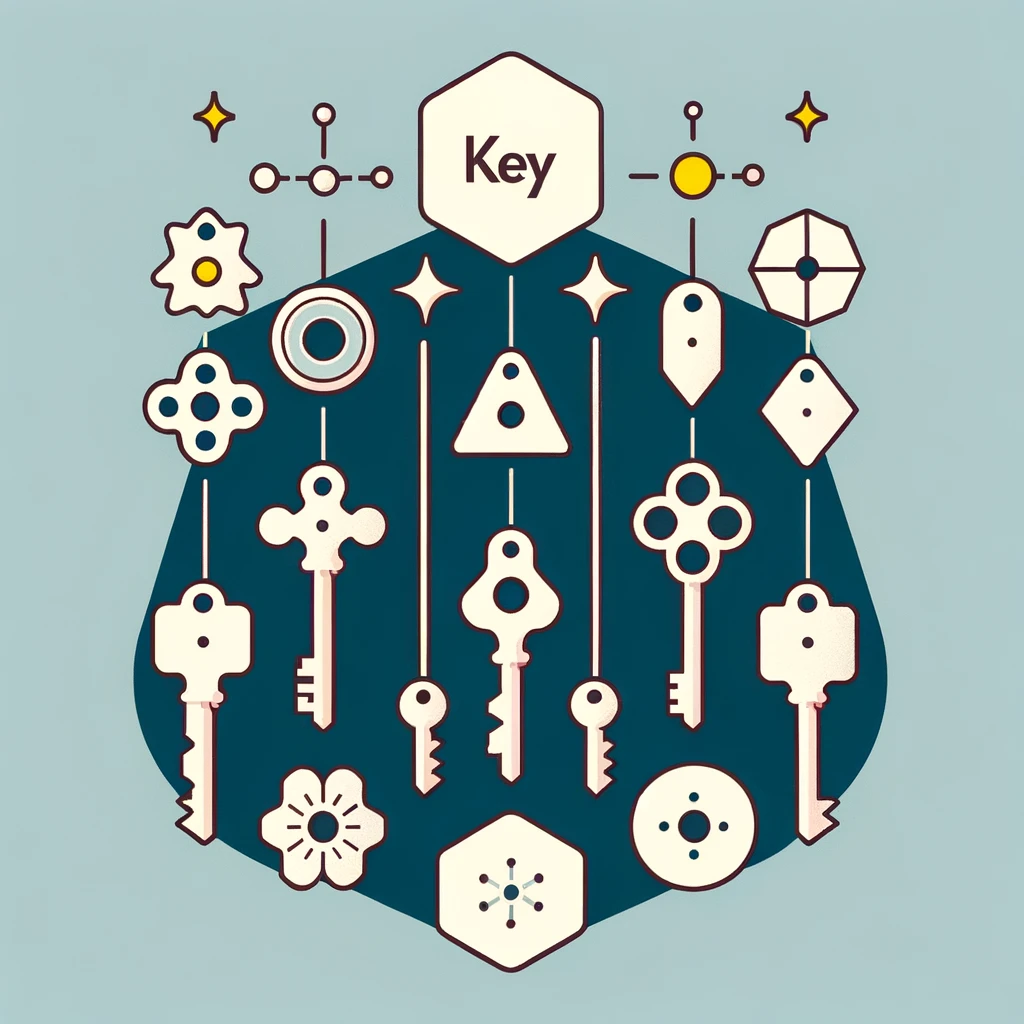
React, a prominent library for building user interfaces, simplifies the creation of interactive web applications. A core aspect of React’s efficiency in rendering lists of elements is the “key” property. This article delves into the significance of the “key” property, its impact on performance, and best practices for using it in your React applications.
Understanding the “key” Property
When rendering a list of elements, React uses the “key” property to track each component’s identity across re-renders. This unique identifier helps React determine which items have changed, been added, or been removed, facilitating efficient updates to the UI without unnecessary re-renders.
function TodoList({ todos }) {
return (
<ul>
{todos.map((todo) => (
<li key={todo.id}>{todo.text}</li>
))}
</ul>
);
}
In this example, todos
is an array of todo items, where each item is an object with id
and text
properties. By assigning todo.id
as the key
for each li
element, we provide React with a stable identity for each component in the list. This allows React to efficiently update the UI by re-rendering only the items that have changed, been added, or removed, rather than re-rendering the entire list on every update.
Without the key
property, if we were to add a new todo item to the list, React would not know which elements have actually changed and might unnecessarily re-render all existing items in addition to the new one. This would lead to performance issues and a potentially sluggish user experience in more complex applications.
Choosing the Right Key
Selecting an appropriate key is crucial for optimizing performance. Here are some guidelines:
Use Unique Identifiers
The best keys are unique and stable identifiers such as database IDs. These ensure that React can accurately track elements over time.
const userList = users.map((user) => (
<li key={user.id}>{user.name}</li>
));
Avoid Index as Key
Using the array index as a key is a common mistake. While indexes are unique, they’re not stable identifiers because they can change as the array is modified, leading to potential issues in performance and component state.
// Not recommended
const userList = users.map((user, index) => (
<li key={index}>{user.name}</li>
));
For components that do not have stable IDs
Consider constructing a unique identifier from their content combining several properties that are guaranteed to stay the same:
const eventLlist = events.map((event) => (
<li key={`${event.time}-${event.user_name}`}>{event.description}</li>
));
In this way, even if there is no unique id
, the key can be “unique enough” combining two or more data points.
Other Common Pitfalls and Tips
- Do not use Math.random() as a key; it defeats the purpose by generating a new key on every render.
- Ensure keys are unique among siblings, but they can be reused in different arrays
Advanced Use Cases
Composite Components
In composite components, the “key” property works in just the same way:
const postList = posts.map((post) => (
<PostComponent key={post.id} title={post.title} content={post.content} />
));
Reordering and Filtering Lists
When lists are dynamic, with filtering or sorting operations, “keys” become even more critical. They help React maintain item consistency despite changes in order or presence.
Handling Keys in Fragments
React also supports keys in fragments, a common pattern for grouping a list of children without adding extra nodes to the DOM.
const tableRows = data.map((item) => (
<React.Fragment key={item.id}>
<td>{item.name}</td>
<td>{item.value}</td>
</React.Fragment>
));
Conclusion
The “key” property plays a pivotal role in optimizing React applications, enabling efficient updates and enhancing overall performance. By adhering to best practices in selecting keys, developers can avoid common pitfalls and ensure their applications are more responsive and performant. Whether dealing with simple lists or complex dynamic content, understanding and correctly applying the “key” property is a vital skill in a React developer’s toolkit.
For more detailed information on the “key” property and other React performance tips, refer to the React documentation. Embracing these practices will lead to more performant and maintainable code, contributing to smoother user experiences and faster applications.