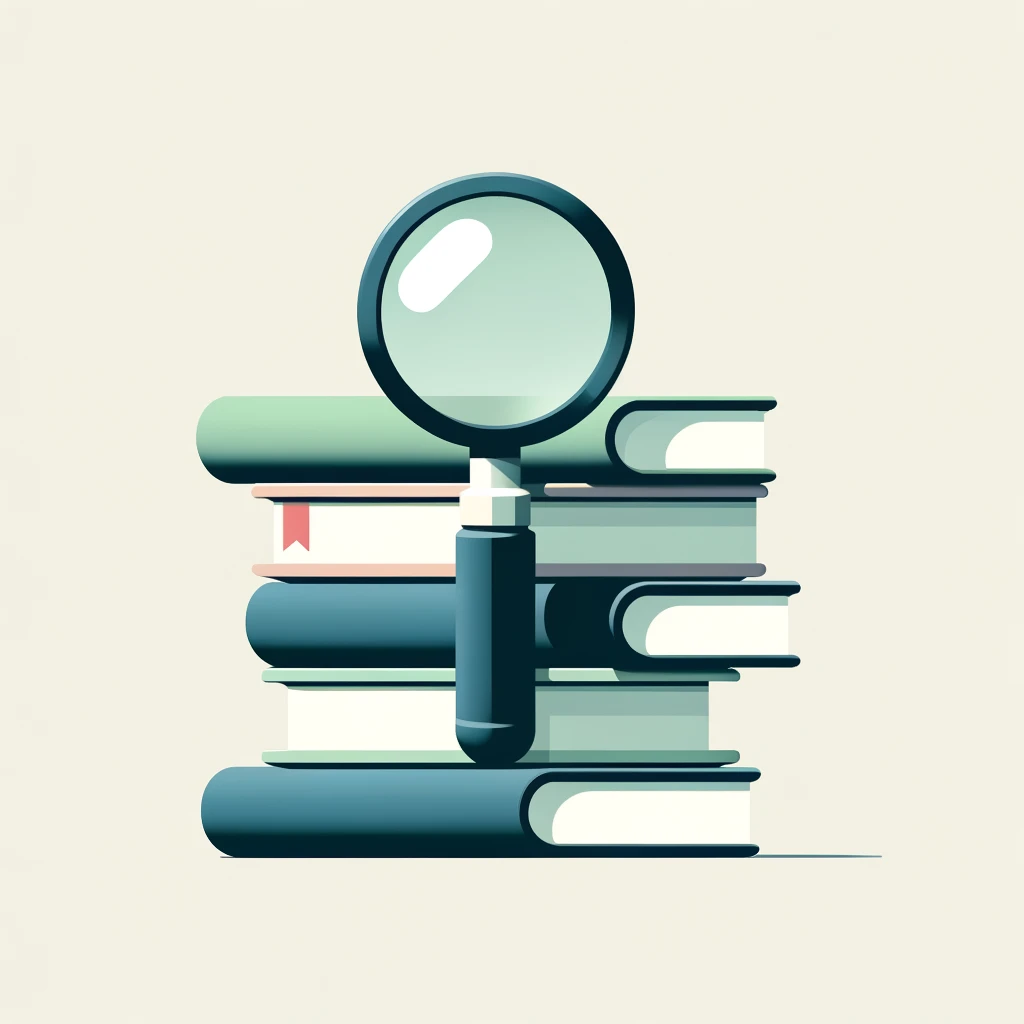
In the realm of software development, particularly within dynamic languages like JavaScript, maintaining clear and comprehensive documentation is paramount. JSDoc emerges as a cornerstone tool for developers aiming to achieve this, providing a structured approach to documenting code which, in turn, enhances maintainability, scalability, and understanding across teams. This post delves into the core of JSDoc—its significance, how to employ it effectively, and best practices that elevate the quality of your JavaScript code documentation.
Understanding JSDoc
JSDoc is a documentation syntax for JavaScript, similar to JavaDoc for Java or PyDoc for Python, enabling developers to annotate their code with comments that can later be transformed into a detailed documentation website. This tool doesn’t just facilitate better code comprehension; it fosters a culture of documentation-first that can significantly reduce the learning curve for new team members and streamline the code review process. It also integrates with popular editors like VS Code and JetBrains, displaying documentation in-editor when developers hover over a specific field or method.
Getting Started with JSDoc
To integrate JSDoc into your project, you’ll need Node.js installed on your machine. With Node.js ready, JSDoc can be included in your project as a dev dependency via npm:
npm install --save-dev jsdoc
Once installed, you can start documenting your code using JSDoc annotations. Here’s a simple example to get you started:
/**
* Calculates the sum of two numbers.
* @param {number} a The first number.
* @param {number} b The second number.
* @returns {number} The sum of the two numbers.
*/
function add(a, b) {
return a + b;
}
In this snippet, the JSDoc comments provide a clear description of what the add
function does, its parameters, and its return value.
Generating Documentation
With your code annotated, generating the documentation is straightforward. Add a script to your package.json
:
"scripts": {
"docs": "jsdoc -r ."
}
Running npm run docs
will generate a out/
directory by default, containing your project’s documentation in HTML format. You can view this by opening the index.html
file in a web browser.
Enhancing Your Documentation
JSDoc is highly versatile, supporting a wide array of tags to enrich your documentation. Here are a few essential tags to incorporate:
@constructor
: Marks a function as a constructor.@deprecated
: Indicates that a method is deprecated.@throws
or@exception
: Documents an exception thrown by a function.@see
: Provides a reference to another symbol in the documentation.@todo
: Highlights tasks to be completed in the code.
Utilizing these tags not only makes your documentation more informative but also assists in code quality tools and IDEs to better understand your code, offering improved autocomplete suggestions and warnings about deprecated usage or potential errors.
Best Practices for Using JSDoc
To maximize the benefits of JSDoc, consider the following best practices:
- Document as You Code: Make documentation a part of your development process, not an afterthought. This ensures your documentation stays up-to-date with your codebase.
- Be Descriptive but Concise: While it’s important to be thorough, avoid overly verbose descriptions. Aim to provide clear, succinct explanations.
- Use Markdown for Richer Formatting: JSDoc supports Markdown within comments, allowing for richer formatting options such as lists, code blocks, and links.
- Leverage Plugins: Extend JSDoc’s functionality with plugins. For example,
jsdoc-babel
enables JSDoc to understand modern JavaScript syntaxes that might not be supported out-of-the-box.
ESLint with JSDoc
There are linters that leverage JSDoc type hints to improve the analysis of JavaScript code. One of the most notable tools is ESLint, a highly configurable linter for JavaScript, which can be extended with plugins to utilize JSDoc comments for type checking and more robust code analysis. ESLint itself doesn’t directly use JSDoc for type checking. However, through the use of plugins such as eslint-plugin-jsdoc, it can enforce consistent JSDoc comments and, to some extent, use the type information specified in JSDoc comments for validating code. This plugin checks the correctness of JSDoc comments with respect to syntax and, optionally, their alignment with the actual code.
npm install eslint --save-dev
npm install eslint-plugin-jsdoc --save-dev
Then, in your .eslintrc.json
, include:
{
"plugins": ["jsdoc"],
"extends": ["eslint:recommended", "plugin:jsdoc/recommended"],
"rules": {
"jsdoc/check-alignment": "error", // Example rule: enforce JSDoc comments alignment
"jsdoc/check-param-names": "error", // Checks that parameter names match those in the function declaration
"jsdoc/check-tag-names": "error", // Ensure that JSDoc tags exist
"jsdoc/check-types": "error", // Enforces using consistent types
"jsdoc/require-jsdoc": ["error", { // Require JSDoc comments for certain nodes
"require": {
"FunctionDeclaration": true,
"MethodDefinition": true,
"ClassDeclaration": true
}
}]
}
}
Now, you can use eslint
:
npx eslint your-file.js
TypeScript as a Type Checker with JSDoc
Another interesting approach involves using TypeScript in conjunction with JSDoc. TypeScript can infer types from JSDoc comments in regular .js
files when certain compiler options are enabled (like checkJs
in the tsconfig.json
or // @ts-check
at the top of a JavaScript file). This allows developers to benefit from TypeScript’s powerful type system and tooling without fully converting their projects to TypeScript. It can catch type-related errors based on JSDoc annotations, making it a powerful tool for developers who prefer to stay within the JavaScript ecosystem but want improved type checking.
Conclusion
Incorporating JSDoc into your JavaScript projects is a step towards more maintainable, understandable, and collaborative code. By following the practices outlined in this post, you can create a robust documentation culture within your team, reducing the time spent onboarding new developers and increasing the overall quality of your codebase. Remember, well-documented code is as vital as the code itself; it ensures your work remains accessible, extensible, and transparent to both your current team and future contributors.
For further exploration, the official JSDoc website offers comprehensive guides and the full list of supported tags, providing everything you need to master this powerful documentation tool.