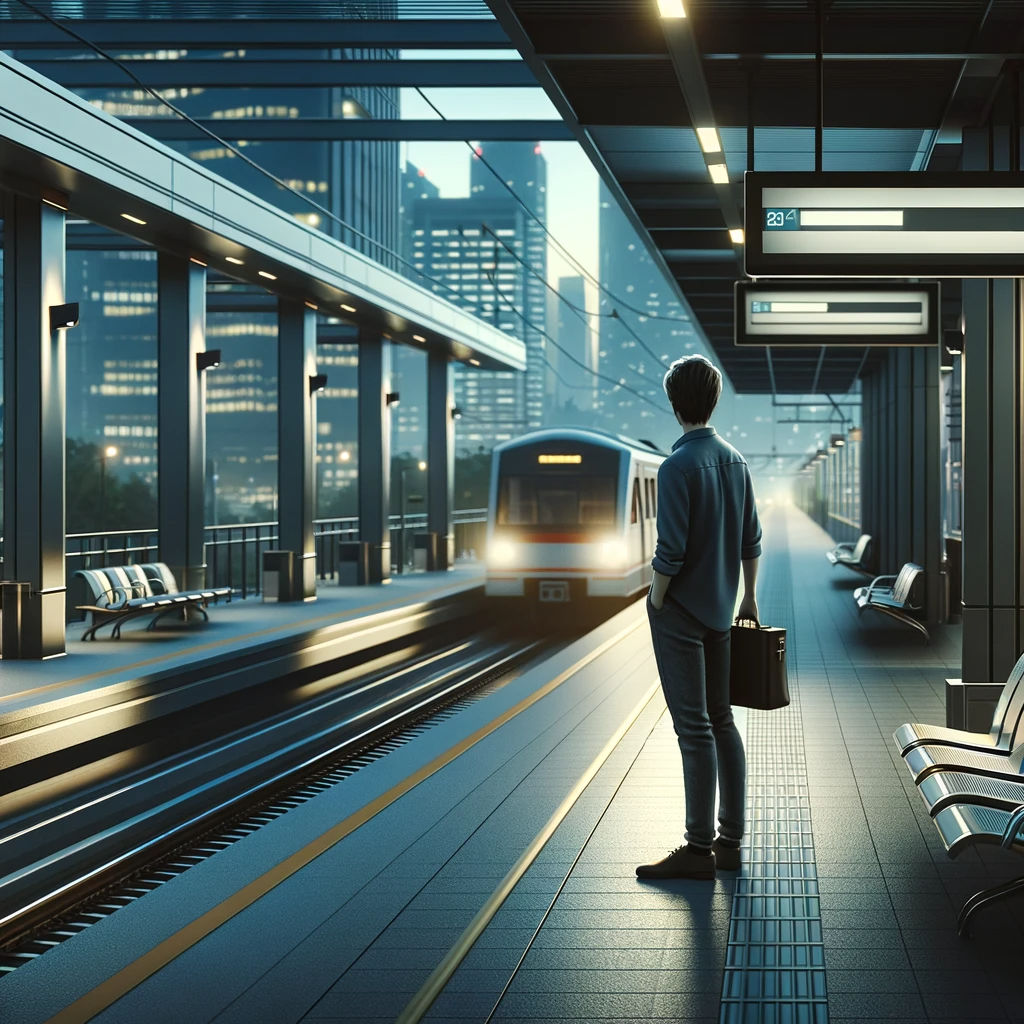
In the realm of ASP.NET, the async/await pattern stands as a powerful tool to enhance the performance and responsiveness of web applications. This approach, central to modern software development, allows developers to perform non-blocking operations, thereby improving the efficiency of resource utilization and application scalability. In this article, we’ll delve into practical strategies and code snippets that demonstrate how to effectively use async/await in ASP.NET, focusing on common pitfalls and secure remedies.
Understanding Async/Await
At its core, the async/await pattern in C# is designed to handle asynchronous operations without the complexity that traditionally accompanies asynchronous programming. The async
keyword marks a method for asynchronous operations, while await
is used to suspend the execution of the method until the awaited task is completed.
Here’s a basic example:
public async Task<ActionResult> GetDataAsync()
{
var data = await SomeAsyncOperation();
return View(data);
}
In this snippet, SomeAsyncOperation
is an asynchronous method that returns a Task
. The await
keyword ensures that the execution of GetDataAsync
is paused until SomeAsyncOperation
completes, without blocking the thread.
Efficient Use of I/O Bound Operations
One of the prime scenarios for async/await in ASP.NET is handling I/O bound operations like database calls, file reads/writes, or web requests. These operations can significantly benefit from asynchronous execution as they often involve waiting for external resources.
Consider the following example of a database operation using Entity Framework:
public async Task<ActionResult> GetUsersAsync()
{
using (var context = new UserDbContext())
{
var users = await context.Users.ToListAsync();
return View(users);
}
}
Here, ToListAsync()
is an async version of ToList()
, ensuring that the database operation does not block the thread while it’s waiting for the response.
Avoiding Common Pitfalls
Deadlocks
One of the most common issues with async/await is deadlocks. These occur when the synchronization context waits for an async operation to complete, but that operation is waiting for the context to be free.
To avoid deadlocks, follow these guidelines:
- Avoid using
.Result
or.Wait()
on tasks; these methods can cause deadlocks in an ASP.NET context. - Always use
ConfigureAwait(false)
when the context is not needed to resume execution, as in library code.
var result = await SomeAsyncMethod().ConfigureAwait(false);
Overuse of Async/Await
While async/await is powerful, it’s not a silver bullet. Overusing it, especially for CPU-bound work, can lead to performance degradation. Remember, async/await is best used for I/O-bound operations.
Enhancing Scalability with Async/Await
Async/await improves scalability by freeing up threads while waiting for I/O operations to complete. This is particularly beneficial in web applications, where each request typically consumes a thread. By using async/await, you can serve more requests with the same number of threads.
Best Practices
Error Handling
Asynchronous methods should include proper error handling to manage exceptions. Use try/catch blocks to catch exceptions from awaited tasks:
public async Task<ActionResult> SafeGetDataAsync()
{
try
{
var data = await SomeAsyncOperation();
return View(data);
}
catch (Exception ex)
{
// Handle exception
return View("Error", ex);
}
}
Using Asynchronous Libraries
Whenever possible, use asynchronous versions of library methods. For instance, if you’re working with Entity Framework, use ToListAsync
instead of ToList
, or ReadAsStringAsync
instead of ReadToEnd
in HttpClient.
Monitoring Performance
Keep an eye on your application’s performance metrics to understand the impact of asynchronous programming. Tools like Application Insights in Azure can be invaluable for this purpose.
Async/Await with ASP.NET Core
If you’re working with ASP.NET Core, the benefits are even more pronounced due to its asynchronous nature. ASP.NET Core is designed to work with asynchronous programming models, making it easier to integrate async/await throughout your application.
Conclusion
The async/await pattern in ASP.NET is a powerful paradigm that, when used correctly, can significantly enhance the performance and scalability of your web applications. By understanding its proper use, avoiding common pitfalls, and adhering to best practices, you can ensure that your application remains responsive and efficient under load.
For more in-depth reading, Microsoft’s official documentation on async/await in ASP.NET is an excellent resource, providing detailed explanations and additional examples.
Remember, while async/await is a powerful tool, it’s not a panacea. It should be used judiciously, especially in scenarios involving I/O bound operations, to truly reap its benefits in terms of performance and scalability.