About the author
Code Habit is an online community of developers maintained by experienced software engineers. The following article was written by one of the core maintainers of the Code Habit community.
Check out more great development resources on Twitter at @habitcode
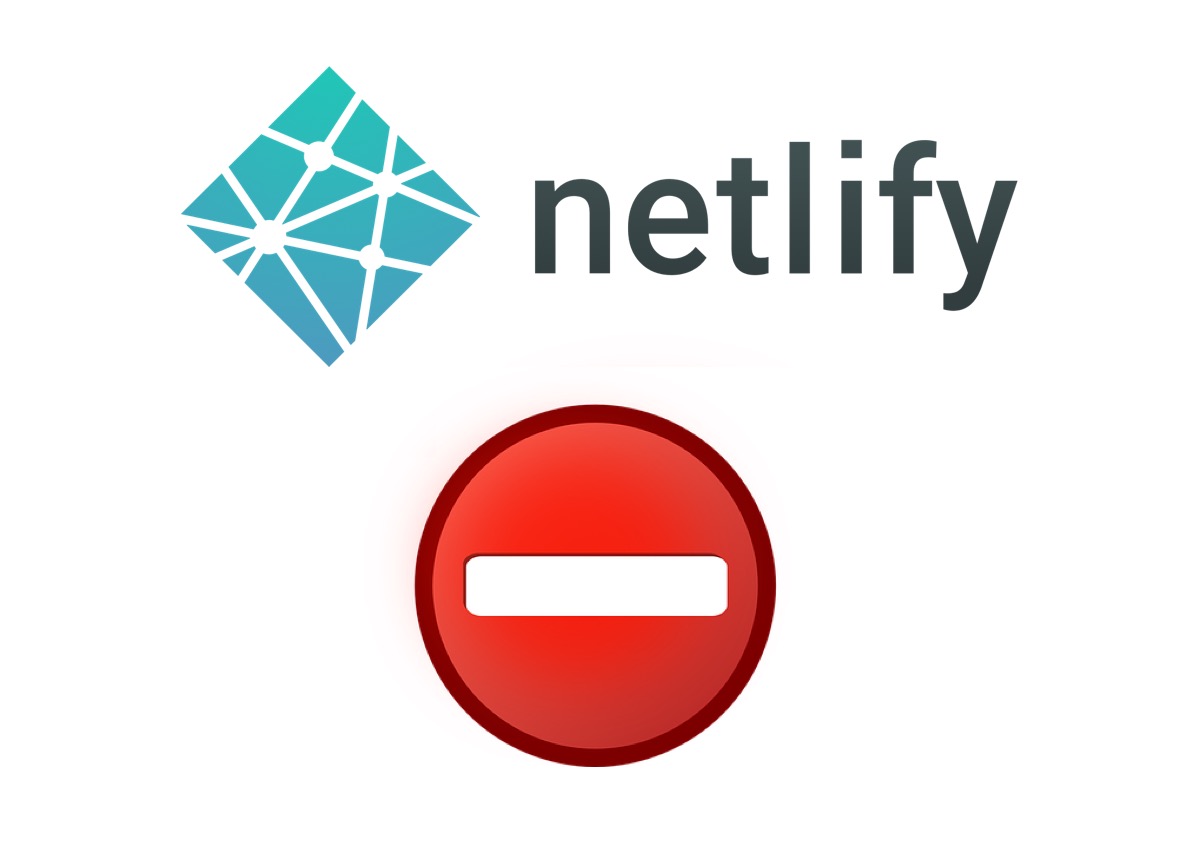
What are Netlify Functions?
Netlify Functions are serverless Lambda functions without the need for an AWS account. The function management is handled directly within Netlify.
These functions can be used to write full API endpoints. Today we are going to make one that checks whether a website is up or not.
Configuration
First, you need to create a Netlify account, if you don’t already have one. With the account created, connect Netlify to your local environment following the next steps:
- Install Netlify’s CLI globally:
npm install netlify-cli -g
- Check if the CLI is available by running
netlify
then authenticate to your Netlify’s account by running:
netlify login
Once Netlify has been connected, create a new project with the following contents:
netlify.toml:
[build]
functions = "lambda"
This tells Netlify to use lambda
as the folder for serverless functions when building.
lambda\checkURL\checkURL.js:
exports.handler = async (event, context, callback) => {
if (event.httpMethod !== 'POST') {
callback(null, {
statusCode: 400,
body: 'You are not allowed to do that.',
});
return;
}
callback(null, {
statusCode: 200,
body: 'Hello world'
});
return;
};
The handler
is our main function of the endpoint. This one will be deployed and served as a serverless function.
Our function has 3 parameters:
- event: data sent by the initiator of the request (body, http method, etc.)
- callback: used to return a response to the initiator of the request.
- context: not needed in our example, it holds information about the context in which the serverless function was called, like certain Identity user information, for example.
As of now, the function does two things.
- It checks if the received request was done through a
POST
method. - Returns a message based on the type of the request (“Hello world” for
POST
requests / “You are not allowed to do that” for any other type).
Let’s see if it works by running netlify dev
. Once the server starts, you’ll be able to access the endpoint under
this http://localhost:8888/.netlify/functions/checkURL
(be aware that 8888 is the port which is being used on my machine, on yours, it might be different).
To check the endpoint, we are going to use Postman.
Before making a request, add Content-Type
as application/x-www-form-urlencoded
to your headers. Sending the request, ‘Hello world’ is coming back as a response. Analogously, looking into our server’s console, we’ll see the request being handled.
Request from ::1: POST /.netlify/functions/checkURL
Let’s also add a parameter to our request:
Add https://google.com
as raw
data on Body. Add console.log(event.body)
right before the final callback then
send. The console will now show up our URL result: https://google.com
.
Now that we know Netlify’s functions work, let’s build our endpoint.
Building the endpoint
Start off by running npm init
then install Axios & Validator npm install axios, validator
. We’ll need these
libraries to validate the input and make requests.
Next, import the libraries and replace the console log, storing the value in a variable:
const axios = require("axios");
const validator = require('validator');
...
const url = event.body;
console.log(url);
Now, let’s check if the URL is an actual link. In case it isn’t, return with a 400 code and error message.
if(!url || !validator.isURL(url)) {
callback(null, {
statusCode: 400,
body: 'URL parameter is missing or is not correct',
});
return;
}
Testing the endpoint once again https://google.com
returns “Hello world” while bananas
returns “URL parameter is
missing or is not correct.”
Moving a step forward, we’re going to make a request for the given URL and see if it’s currently active.
const timeout = 3000;
const response = await axios.get(url, {timeout}).catch(error => console.log(error));
const isDown = response && response.status ? ![200, 201, 202, 301, 302].includes(response.status) : true;
This makes a request using Axios and, based on the response, determines whether the URL is accessible
or not. Codes such as 200
, 201
, 202
mean the URL is okay, 301
and 302
indicate a redirect, but still up. If
there’s any other status code, we can assume the given URL is down. We also limit the request to timeout after 3
seconds so we don’t make the user wait for too long.
The last thing to do is to modify the callback to return the answer, so instead of “Hello world” send the url
and
isDown
variables.
callback(null, {
statusCode: 200,
body: JSON.stringify({
url,
isDown,
})
});
Testing once again, for Google we get isDown = false
, for something like https://googleeeeex.com
we get true.
Great!
Deploying to Netlify
Now, in order to use this function anywhere you’d like, you have to deploy it to Netlify. The process itself is very simple; follow this guide for implementation.
Once deployed, you’ll find your function under https://app.netlify.com/sites/your-app-name/functions, its URL will be
https://your-app-name.netlify.app/.netlify/functions/checkURL
Next steps
As I usually say, this is only the tip of the iceberg. You can enhance this endpoint to return more information about the link, such as the request time, previous checks, etc. You could also create the frontend interface which takes the URL and passes it to the endpoint.
Check out more great development resources on Twitter at @habitcode