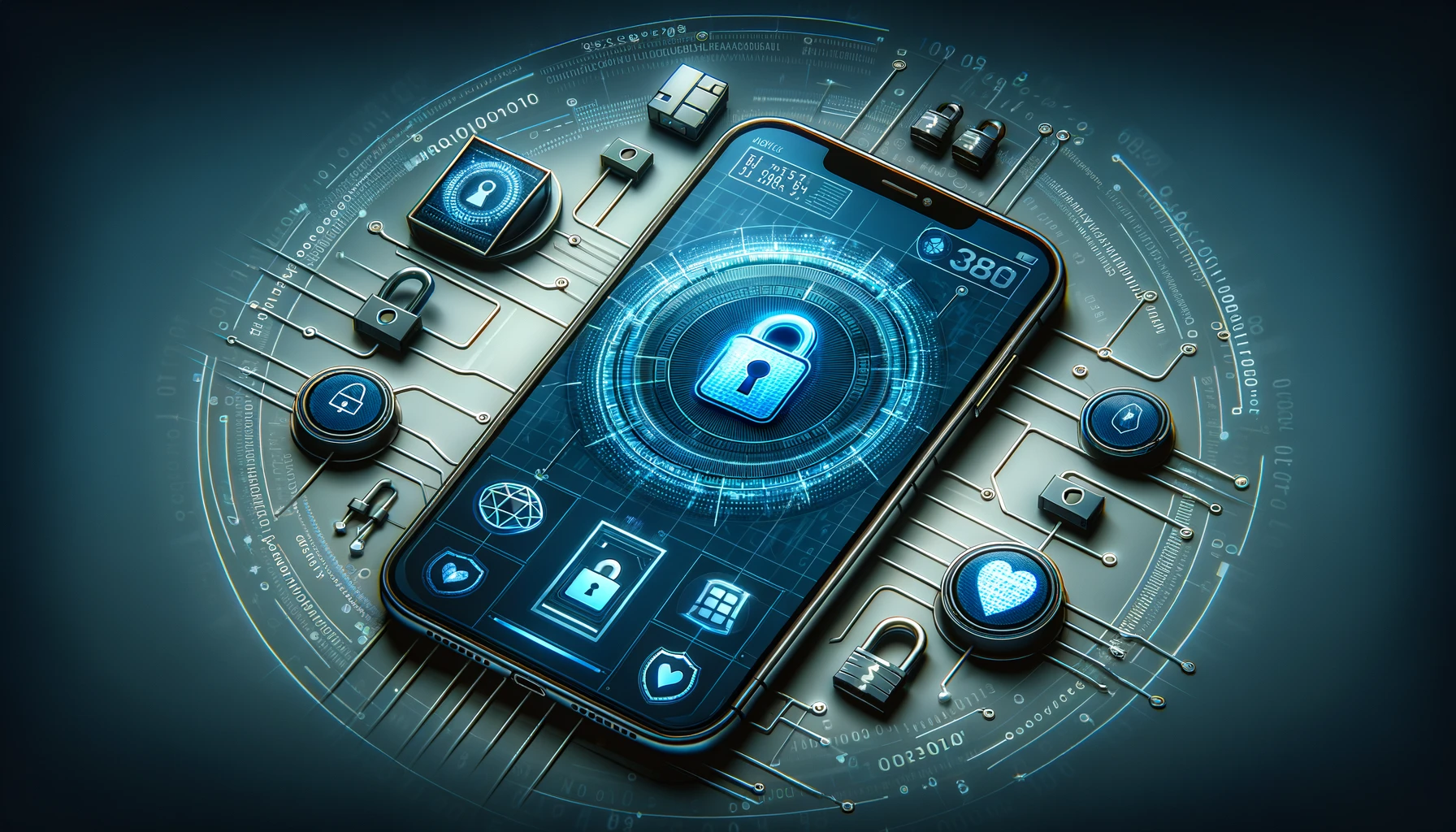
In the realm of iOS development, managing application secrets like API keys, database passwords, and third-party service credentials is crucial yet often overlooked. This post delves into the critical aspects of iOS app secret management, outlining best practices and strategies to ensure your app’s security and data integrity.
Understanding the Risks of Poor Secret Management
Before jumping into the solutions, let’s address why proper secret management is vital. Hardcoding secrets in your app’s source code, or even bundling them with your app, exposes your application to significant security risks. Decompilation tools can easily extract these secrets, leading to unauthorized access and potential data breaches.
Strategies for Storing Secrets Safely
1. Environment Variables and Build Configurations
One way to keep secrets out of your codebase is using environment variables and different build configurations. Xcode’s build settings and configuration files (like .xcconfig
files) can be used to manage environment-specific settings and variables. These configurations can be excluded from version control to keep sensitive information out of your source code repository.
Here is an example of how to use .xcconfig
files to manage secrets:
// Development
API_KEY = EXAMPLE_API_KEY
OTHER_VARIABLE = EXAMPLE_VARIABLE
2. Secure Enclaves and Keychains
For storing secrets at runtime, iOS provides a secure and encrypted storage mechanism: the Keychain. The Keychain is ideal for storing small pieces of sensitive data, like tokens and passwords. It’s encrypted with the user’s passcode, making it a robust option for securing credentials.
Here’s a basic example of using the Keychain in Swift:
import Security
class KeychainManager {
func store(key: String, value: String) {
let query: [String: Any] = [
kSecClass as String: kSecClassGenericPassword,
kSecAttrAccount as String: key,
kSecValueData as String: value.data(using: .utf8)!
]
SecItemAdd(query as CFDictionary, nil)
}
func retrieve(key: String) -> String? {
let query: [String: Any] = [
kSecClass as String: kSecClassGenericPassword,
kSecAttrAccount as String: key,
kSecReturnData as String: kCFBooleanTrue!,
kSecMatchLimit as String: kSecMatchLimitOne
]
var item: CFTypeRef?
if SecItemCopyMatching(query as CFDictionary, &item) == noErr {
if let data = item as? Data {
return String(data: data, encoding: .utf8)
}
}
return nil
}
}
This code provides a straightforward way to store and retrieve secure data using the iOS Keychain.
3. Avoid Storing Secrets Whenever Possible
If you can avoid storing secrets on the client side, do so. For instance, use OAuth for user authentication, where tokens are granted by an authentication server and can be revoked when needed.
Managing Server-Side Secrets
Fetching Secrets Securely
When an iOS app needs to fetch secrets from a server, like an access token after logging in, it’s crucial to do so securely. Ensure communication with your backend is over HTTPS, using TLS to protect the data in transit.
Refresh Tokens and Short-Lived Access Tokens
Implementing short-lived access tokens and refresh tokens is a good practice. Access tokens expire after a short time, reducing the risk if they are compromised. The so called ‘refresh tokens’, with a longer life, stay on the device and are used to obtain new access tokens.
Biometric Authentication for Accessing Secrets
Leverage iOS’s biometric authentication features like Face ID or Touch ID for accessing sensitive information. This adds an extra layer of security, ensuring only the authenticated user can access the stored secrets.
Tools and Libraries to Enhance Security
Keychain Access Library
For simplifying interactions with the Keychain, consider using a library like KeychainAccess. It provides a more straightforward API for storing and retrieving data securely.
CryptoSwift for Encryption
If additional encryption is needed, CryptoSwift offers a comprehensive suite of cryptographic algorithms and utilities for Swift.
Network Security Configuration
Utilize iOS’s Network Security Configuration to enforce HTTPS and specify trusted certificates, enhancing the security of your app’s network communications.
Implementing Continuous Security Checks
Code Reviews and Static Analysis Tools
Regular code reviews focusing on how secrets are handled can prevent security mishaps. Static analysis tools can automatically detect hardcoded secrets or misconfigurations in your code.
Penetration Testing
Regular penetration testing can uncover vulnerabilities in how your app handles secrets, both on the client and server sides. Penetration testing is where security experts simulate attacks to identify and fix potential security issues. You can pay for professional penetration testing services (including HackerOne’s).
Conclusion
Effective secret management is a cornerstone of iOS app security. By avoiding hardcoded secrets, leveraging iOS’s secure storage options, and implementing robust server-side strategies, developers can significantly reduce the risk of sensitive data exposure. Regular security audits, penetration testing, and using reliable third-party libraries can further fortify your app’s defenses. Remember, the goal is not just to protect the secrets but to safeguard the users and their data, maintaining trust and compliance.
For further reading and resources, Apple’s official Keychain Services documentation and the CryptoSwift GitHub repository provide extensive insights and examples. Additionally, exploring OAuth 2.0 specifications and best practices will enhance your understanding of secure authentication mechanisms.