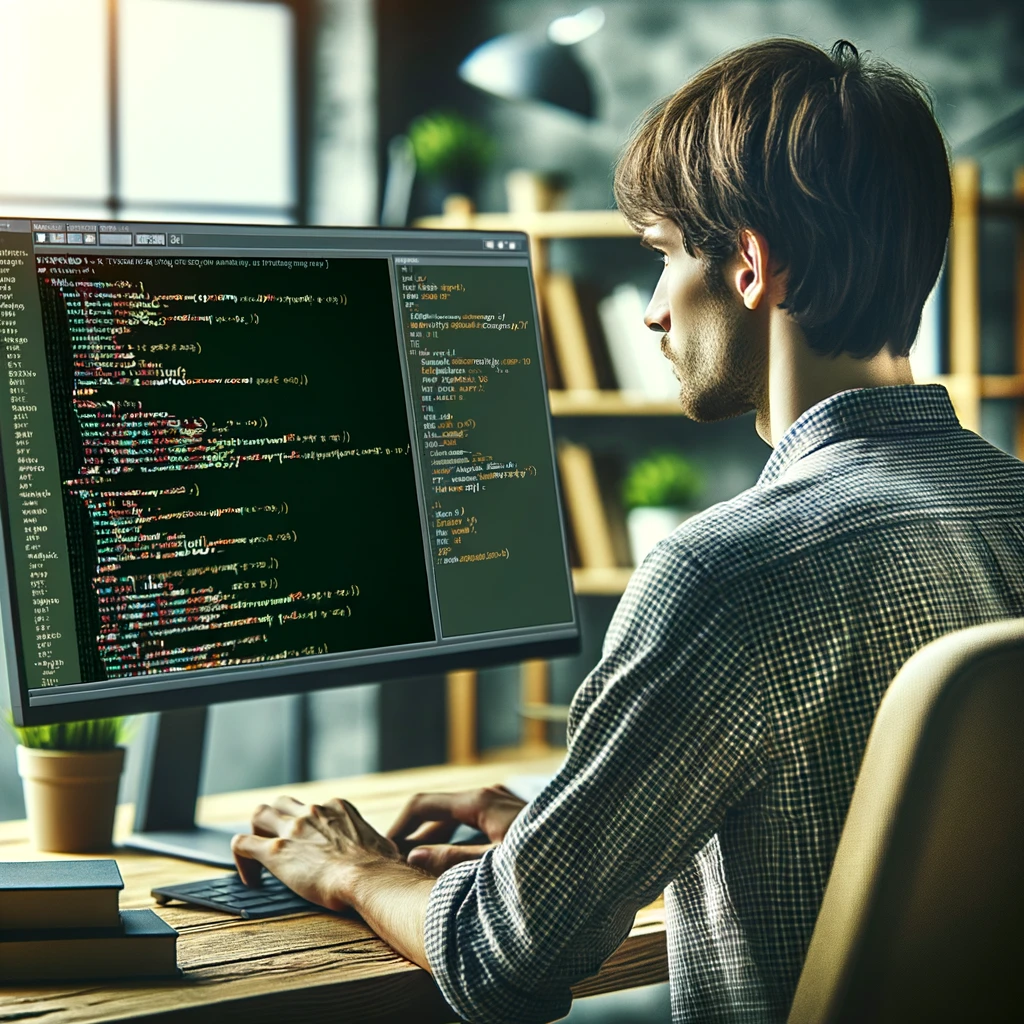
For software developers, mastering debugging is a key component of their professional skill set. Python, known for its simplicity and readability, provides a powerful yet user-friendly tool for debugging – the Python Debugger (pdb). This article explores the basics of pdb, offering insights into its functionality, and demonstrates how it can be a game-changer in your debugging process.
Understanding pdb: Your Debugging Ally
The Python Debugger, or pdb, is a module that comes bundled with the standard Python distribution. It provides an interactive debugging environment where you can set breakpoints, step through code, inspect variables, and evaluate expressions. This interactive nature allows you to understand better and fix bugs more efficiently.
Setting Up pdb in Your Code
To use pdb, you first need to import it into your Python script. This can be done by simply adding import pdb
at the beginning of your file. Once imported, you can set breakpoints in your code by calling pdb.set_trace()
. This function call pauses your program’s execution and enters the pdb interactive mode.
Consider the following simple Python script:
import pdb
def calculate_sum(a, b):
pdb.set_trace()
return a + b
result = calculate_sum(5, 3)
print(result)
When you run this script, the execution will stop at pdb.set_trace()
and you’ll be taken to the interactive debugger.
Navigating Through Your Code
Once in pdb, you have several commands at your disposal:
list
(orl
): Displays 11 lines around the current line.step
(ors
): Steps into the function call.next
(orn
): Goes to the next line in the current function.continue
(orc
): Continues execution until the next breakpoint.break
(orb
): Sets a breakpoint.
Using these commands, you can closely inspect how your code executes, line by line.
Inspecting Variables and Expressions
One of the key advantages of pdb is the ability to inspect variables and evaluate expressions on the fly. For instance, in the above example, while in pdb mode, typing print(a)
would display the value of a
. You can also evaluate expressions like a + b
or even modify variable values.
An Example Scenario
Let’s consider a more complex example. Suppose you have a function that’s supposed to calculate the factorial of a number, but it’s not working as expected:
import pdb
def factorial(n):
pdb.set_trace()
if n == 1:
return 1
else:
return n * factorial(n-1)
print(factorial(5))
By stepping through this function with pdb, you can observe how the recursion works and verify if the calculations are correct at each step.
Beyond Basics: Advanced pdb Features
While the basics of pdb are straightforward, there are more advanced features worth exploring:
- Conditional breakpoints: Set breakpoints that are triggered only when a certain condition is met.
- Post-mortem debugging: Analyze a program after it has crashed.
- Remote debugging: Debug code running on a remote machine.
These advanced features further enhance pdb’s capabilities, making it a robust tool for tackling complex debugging scenarios.
Conclusion
The Python Debugger is a versatile tool that can significantly improve your debugging efficiency. It’s user-friendly yet powerful, making it suitable for both beginners and experienced developers. By integrating pdb into your development workflow, you can gain deeper insights into your code’s behavior and swiftly resolve bugs. Remember, effective debugging is not just about fixing errors; it’s about understanding your code better. With pdb, you’re well-equipped to master this essential aspect of programming.
Stay tuned for more insights and tips on software security and code review in future posts. Happy debugging!