About the author
@catalinmpit is a software engineer, AWS community builder and technical writer based out of London. He’s currently an engineer at TypingDNA, working on applying keystroke dynamics as a means of biometrics authentication.
Check out more of his work on catalins.tech
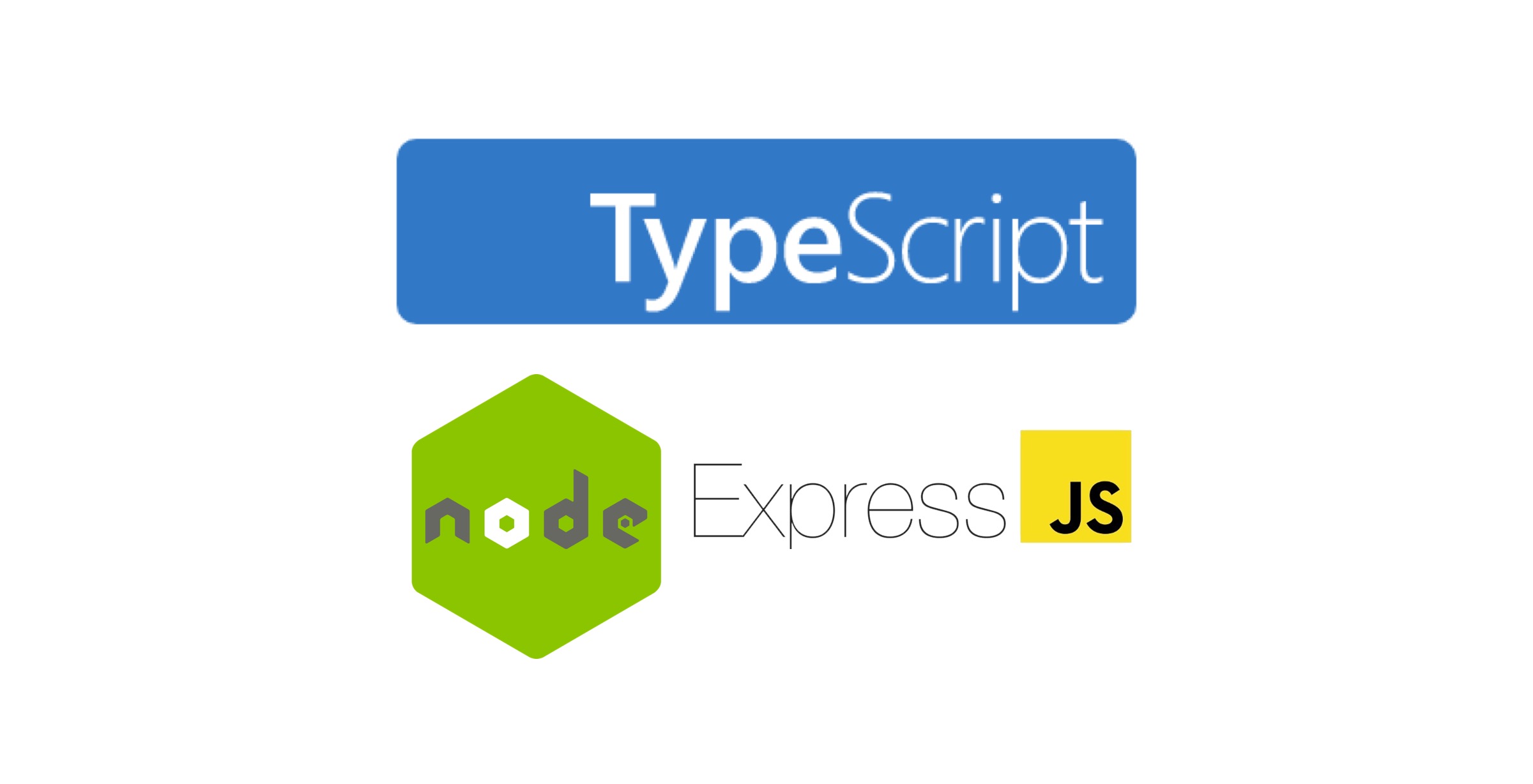
In this tutorial, you will learn how to use TypeScript in a Node.js and Express project. Note that the purpose of this article is to demonstrate how to create a project with the mentioned frameworks and programming language. Its purpose is not to advocate or debate the merits of using TypeScript.
Before getting started, note that this article assumes the reader:
- Has a basic knowledge of TypeScript, Node.js, and Express.
- Is using, or plans to use, Node.js version 12 or greater.
1. Set up the project
The first step is to create a directory for the project and initialize it. Run the following commands to create an
empty directory called typescript-nodejs
, and change the current directory to it:
mkdir typescript-nodejs
cd typescript-nodejs
From the typescript-nodejs
directory, you have to initialize our Node project. To do so, run the following command:
npm init -y
Using the -y
flag in the above command generates the package.json
file with the default values. Instead of adding
information like the name and description of the project ourselves, npm initializes the file with default values
which can be updated later.
With the project initialized, now we can add the required dependencies.
2. Add dependencies
TypeScript and the Express framework will need to be added. To do so, run the following commands:
npm install express
npm install typescript ts-node @types/node @types/express --save-dev
You’ll notice TypeScript-related dependencies are installed as devDependencies
. This is because even though code is
written in TypeScript, it will be compiled as “vanilla” JavaScript. TypeScript is not required, or used, for runtime
and is only used during development. Since the developer is the only “consumer” of TypeScript, it should be
installed as a dev dependency.
Moving forward, your package.json
should look as follows after the dependency installations are complete:
{
"name": "typescript-nodejs",
"version": "1.0.0",
"description": "",
"main": "index.js",
"dependencies": {
"express": "^4.17.1"
},
"devDependencies": {
"@types/express": "^4.17.9",
"@types/node": "^14.14.20",
"ts-node": "^9.1.1",
"typescript": "^4.1.3"
},
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
3. Configure TypeScript
So far, you only installed TypeScript, but you cannot use it yet. The reason is that you need to configure it. You
need to create a file called tsconfig.json
, which indicates that the directory is the root of a TypeScript or
JavaScript project.
npx tsc --init
Running the above command creates the tsconfig.json
file where we can customize the TypeScript configuration. The
newly created file will contain a lot of code, most of which is commented out. However, there are some settings that
are important to know:
target: using this option, you can specify which ECMAScript version to use in your project. For instance, if you set the target to ES5 and then you use arrow functions, the code is compiled to an equivalent ES5 function. The available versions are
ES3
(default),ES5
,ES2015
,ES2016
,ES2017
,ES2018
,ES2019
,ES2020
, orESNEXT
.module: with this option, you can specify which module manager to use in the generated JavaScript code. You can choose between the following values
none
,commonjs
,amd
,system
,umd
,es2015
,es2020
, orESNext
. The most common module manager and the default one iscommonjs
.outDir: with this option, we can specify where to output the “vanilla” JavaScript code.
rootDir: specifies where the TypeScript files are located.
strict: enabled by default, this toggles strict type-checking options.
esModuleInterop: this option is
true
by default; it controls interoperability between CommonJS and ES modules. It does this by creating namespace objects for all imports.
For in-depth information about all the options available, I recommend checking the TypeScript TSConfig Reference.
4. Create an Express server
With TypeScript configured, we can create the Express web server. First, create the file index.ts
(attention to
the file extension) by running touch index.ts
.
import express from 'express';
const app = express();
app.get('/', (req, res) => {
res.send('Well done!');
})
app.listen(3000, () => {
console.log('The application is listening on port 3000!');
})
Now you have a simple web server that shows “Well done!” when you access localhost:3000
in a browser. The server is
very simple and doesn’t (yet) take advantage of TypeScript. However, the purpose of this tutorial is to make
Node.js, TypeScript, and Express work together and create a boilerplate. From there, the application can be customized.
Whenever you make changes and want to run the application, you’ll need to compile TypeScript to “vanilla” JavaScript. To do that, run:
npx tsc --project ./
The command tsc
compiles TypeScript to JavaScript. The flag --project
specifies from where to pick the TS files.
Lastly, ./
specifies the root of the project.
When you access the build
folder, you should see the compiled JavaScript code. That is, the code compiled from the
TypeScript code you wrote.
5. Create scripts
It can be tedious to write npx tsc --project ./
each time the code needs to be compiled. To automate this, we can
add a script to package.json
that takes care of that for us.
To automate compiling the TypeScript code to JavaScript, Add the following line of code in package.json
under scripts:
"build": "tsc --project ./"
Now you can run npm run build
to compile the code. This way, it’s simpler and quicker.
Conclusion
Now you know how to create a TypeScript + Node.js + Express boilerplate. This is just the tip of the iceberg, so you can build any application you want from here.
Catalin regularly posts helpful development tips and guides on Twitter. Be sure to follow him at @catalinmpit