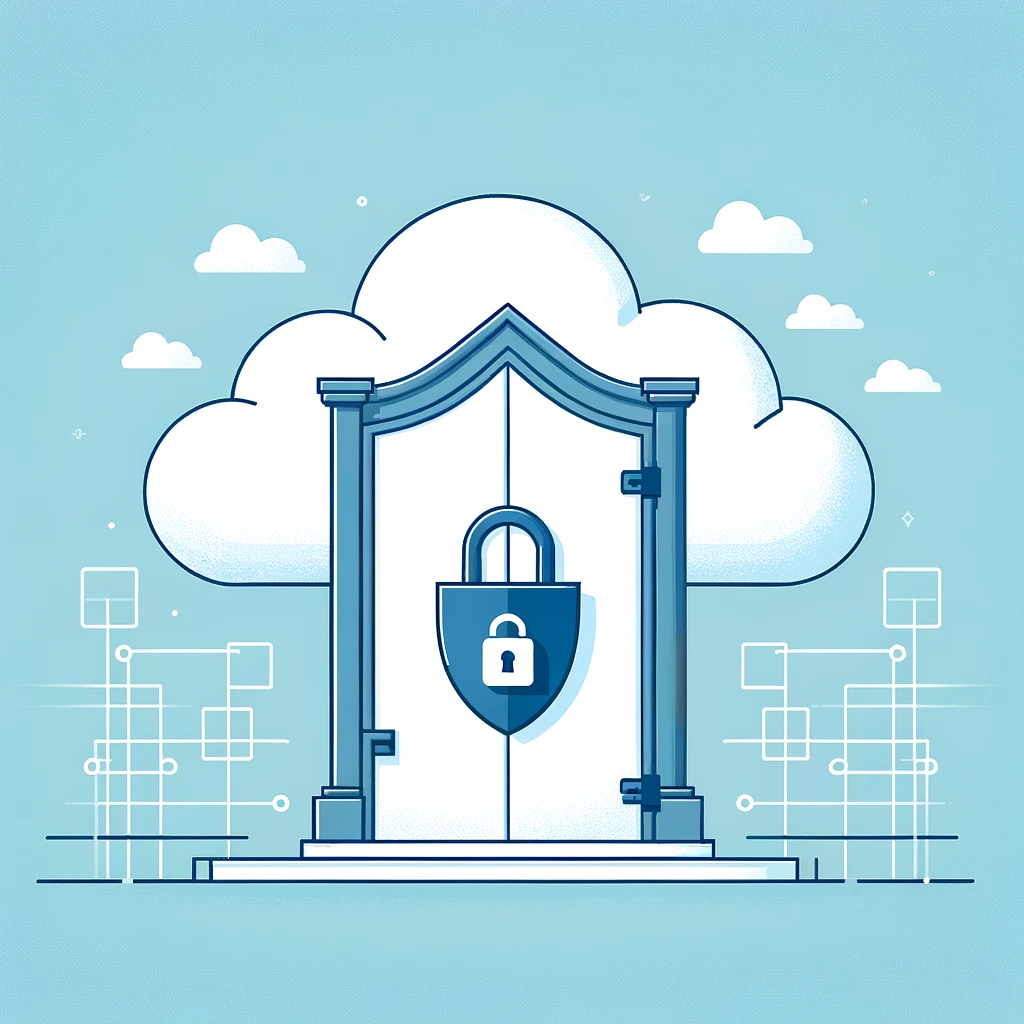
In the realm of modern web development, securing and managing traffic to backend services has become more crucial than ever. The introduction of API gateways as a pivotal component in the architecture of microservices has revolutionized the way developers secure, manage, and scale API traffic. This post delves into the implementation of API gateways to bolster security and streamline traffic management, focusing on the use of Infrastructure as Code (IaC) through the AWS Cloud Development Kit (CDK) for deploying robust solutions.
Understanding API Gateways
An API gateway acts as a reverse proxy, sitting between client applications and backend services. It’s responsible for request routing, composition, and protocol translation, with features aimed at providing a central point for enhancing security, monitoring, and managing traffic. By abstracting the backend services, it also facilitates a more manageable way to implement APIs, service versioning, and introduces a layer where security policies can be enforced.
Key Features and Benefits
- Security: Centralizing security features such as SSL terminations, OAuth, API keys, and rate limiting to protect against common threats.
- Traffic Management: Load balancing, request throttling, and routing based on various criteria to efficiently manage the flow of information.
- Monitoring and Analytics: Collecting detailed analytics on API usage, performance metrics, and error rates to improve service visibility and control.
Leveraging AWS CDK for API Gateway Deployment
The AWS Cloud Development Kit (CDK) allows you to define your cloud resources using familiar programming languages. This approach not only streamlines the process of deploying API gateways but also aligns with best practices in IaC, promoting repeatability, version control, and automation in cloud resource provisioning.
Setting Up Your Environment
Before diving into the implementation, ensure you have the AWS CDK installed and configured in your environment. The AWS CDK supports several programming languages, including TypeScript, Python, Java, and .NET. For this example, we’ll use TypeScript for its robust tooling and community support.
First, install the AWS CDK CLI globally using npm:
npm install -g aws-cdk
Next, bootstrap your CDK project:
cdk init app --language=typescript
Defining the API Gateway
With your CDK project initialized, the next step is to define the API Gateway resource. AWS CDK provides a high-level construct library for Amazon API Gateway called @aws-cdk/aws-apigateway
that simplifies the creation of API Gateways with various configurations.
Install the necessary CDK library for API Gateway:
npm install @aws-cdk/aws-apigateway
In your CDK stack (lib/your-stack-name.ts
), you can define an API Gateway with a simple HTTP endpoint as follows:
import * as cdk from '@aws-cdk/core';
import * as apigateway from '@aws-cdk/aws-apigateway';
export class YourStackName extends cdk.Stack {
constructor(scope: cdk.Construct, id: string, props?: cdk.StackProps) {
super(scope, id, props);
// Define the API Gateway
const api = new apigateway.RestApi(this, 'MyApiGateway', {
restApiName: 'MyService',
description: 'API Gateway for MyService.',
});
// Define a resource and method for the API Gateway
const resource = api.root.addResource('myresource');
resource.addMethod('GET'); // Example method
}
}
This snippet creates an API Gateway with a single resource (/myresource
) and a GET method. You can further customize the API Gateway by integrating it with other AWS services like Lambda functions, enabling CORS, setting up authorizers, and defining usage plans.
Enhancing Security
Security is paramount when exposing your services to the web. AWS CDK facilitates the implementation of security measures such as rate limiting, authorization, and access control.
For instance, to add a usage plan and API keys to your gateway for controlling and monitoring access, you can extend the previous example as follows:
// Create an API Key
const apiKey = new apigateway.ApiKey(this, 'MyApiKey', {
apiKeyName: 'MyServiceApiKey',
description: 'API Key for accessing MyService',
});
// Create a usage plan
const plan = api.addUsagePlan('UsagePlan', {
name: 'Basic',
description: 'Basic usage plan with quota limit',
apiKey: apiKey,
throttle: {
rateLimit: 10,
burstLimit: 2,
},
quota: {
limit: 1000,
period: apigateway.Period.MONTH,
},
});
// Associate the usage plan with a stage
plan.addApiStage({
stage: api.deploymentStage,
});
This code snippet demonstrates how to create an API key and a usage plan with specific throttle and quota limits, providing a basic level of security and control over API access.
Traffic Management Strategies
Effective traffic management ensures that your backend services remain responsive and available, even under heavy load or during maintenance periods. The AWS CDK enables the configuration of traffic management features such as stage environments, canary deployments, and throttling.
For example, implementing a canary deployment to gradually shift traffic to a new version of your API can be accomplished by configuring the deployment preferences in your CDK stack:
const deployment = new apigateway.Deployment(this, 'MyApiDeployment', {
api: api,
// Additional deployment settings
});
const stage = new apigateway.Stage(this, 'MyApiStage', {
deployment: deployment,
stageName: 'prod',
// Enable canary deployment
canarySettings: {
percentTraffic: 10, // Start with 10% of traffic
stageVariableOverrides: {
// Stage variables for canary
},
},
});
This configuration directs 10% of traffic to the canary release, allowing you to monitor the new version’s performance and stability before fully rolling it out.
Monitoring and Analytics
Gaining insights into API performance and usage patterns is critical for maintaining service quality and availability. The AWS CDK, integrated with Amazon CloudWatch, offers a straightforward approach to setting up logging and monitoring for your API Gateway:
// Enable CloudWatch logs for the API Gateway
const logGroup = new logs.LogGroup(this, 'ApiGatewayLogGroup');
api.addGatewayResponse('Default4XX', {
type: apigateway.ResponseType.DEFAULT_4XX,
responseHeaders: {
'Access-Control-Allow-Origin': "'*'",
'Access-Control-Allow-Headers': "'Content-Type,X-Amz-Date,Authorization,X-Api-Key,X-Amz-Security-Token'",
},
templates: {
application/json: '{"message":$context.error.messageString}',
},
statusCode: '400',
});
This example enables default 4XX responses with custom headers and message templates, facilitating better client-side error handling and providing valuable insights through CloudWatch logs.
Conclusion
Implementing an API Gateway using the AWS Cloud Development Kit is a powerful strategy for enhancing the security, reliability, and scalability of your microservices architecture. By centralizing the management of security policies, traffic routing, and analytics, you create a robust foundation for your backend services. The AWS CDK further streamlines this process, offering a code-first approach to defining and deploying cloud resources, thereby accelerating development cycles and ensuring best practices in cloud infrastructure management.
As applications grow in complexity, the role of API gateways will continue to evolve, becoming more integral to achieving operational excellence and providing secure, high-performing services. By leveraging the AWS CDK for your API Gateway deployments, you embrace a modern infrastructure-as-code paradigm that sets the stage for future innovations and scalable growth.