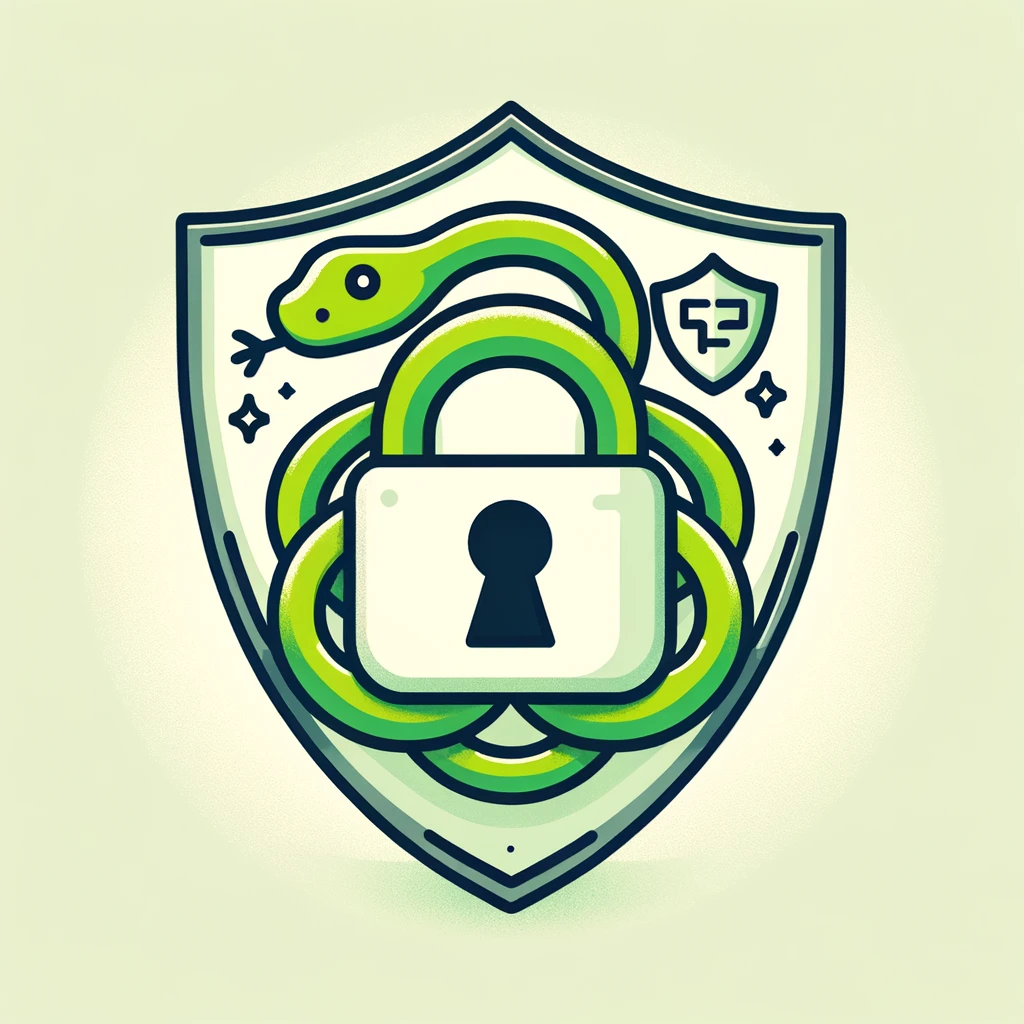
In the modern era of web development, ensuring the security of web applications is paramount. This is particularly true when it comes to transmitting sensitive data over the internet. HTTPS (Hypertext Transfer Protocol Secure) plays a crucial role in safeguarding this data by encrypting the connection between the client and the server. For web applications developed with Django, a high-level Python web framework, implementing HTTPS is essential for protecting user data and enhancing application security. This blog post delves into the best practices for implementing and auditing HTTPS in Django, offering practical examples and code snippets to guide you through the process.
Understanding HTTPS and Its Importance
Before we dive into the implementation details, let’s briefly review what HTTPS is and why it’s important. HTTPS is an extension of the HTTP protocol with an added layer of security, provided by the Transport Layer Security (TLS) or its predecessor, Secure Sockets Layer (SSL). This secure layer encrypts data exchanged between the user’s browser and the web server, making it difficult for malicious actors to intercept and decipher sensitive information.
The use of HTTPS has become a standard practice for all websites, not just those handling sensitive transactions. Apart from enhancing security, HTTPS also boosts user trust and can contribute to higher search engine rankings.
Enforcing HTTPS in Django
Django comes with built-in features that make it easier to enforce HTTPS across your web application. Here are the steps and best practices for implementing HTTPS in Django:
1. Use Django Security Middleware
Django’s security middleware includes several settings that help enforce HTTPS usage.
SECURE_SSL_REDIRECT = True
SECURE_HSTS_SECONDS = 31536000 # 1 year
SECURE_HSTS_INCLUDE_SUBDOMAINS = True
SECURE_HSTS_PRELOAD = True
- SECURE_SSL_REDIRECT: When set to
True
, this setting redirects all HTTP requests to HTTPS. This ensures that users always interact with your site over a secure connection. - SECURE_HSTS_SECONDS: This setting enables HTTP Strict Transport Security (HSTS), instructing browsers to only communicate with your application over HTTPS for a given amount of time. This prevents certain types of man-in-the-middle attacks.
- SECURE_HSTS_INCLUDE_SUBDOMAINS: This setting ensures that the HTTP Strict Transport Security policy is applied not only to the website’s domain but also to all of its subdomains, enhancing security across the entire domain hierarchy (ex.
example.com
,app.example.com
,admin.example.com
). - SECURE_HSTS_PRELOAD: This setting indicates that the website wishes to be included in the browser’s preload list for HTTP Strict TransportSecurity (HSTS), ensuring it is always accessed via HTTPS, even on the first visit.
See Django: “Settings” for more.
2. Obtain and Install an SSL/TLS Certificate
To establish a secure HTTPS connection, your Django server needs an SSL/TLS certificate. You can obtain a certificate from a Certificate Authority (CA). Let’s Encrypt is a popular choice for a free, automated, and open CA. However, these certificates are only valid for 90 days.
After acquiring the certificate, configure your web server (e.g., Nginx, Apache) to use it. Here’s an example for Nginx:
server {
listen 443 ssl;
server_name yourdomain.com;
ssl_certificate /path/to/your/fullchain.pem;
ssl_certificate_key /path/to/your/privkey.pem;
# SSL configuration...
}
3. Use Django’s Built-in Features for Secure Cookies
Cookies can contain sensitive information. To protect this data, Django offers settings to enforce secure cookies:
SESSION_COOKIE_SECURE = True
CSRF_COOKIE_SECURE = True
- SESSION_COOKIE_SECURE: When set to
True
, Django will only send the session cookie over HTTPS connections. - CSRF_COOKIE_SECURE: Similarly, setting this to
True
ensures the CSRF protection cookie is only sent over HTTPS.
See Django: “Settings” for more.
Auditing Your Django Application for HTTPS Compliance
Once HTTPS is implemented, regularly auditing your application for compliance and vulnerabilities is crucial. Here are some strategies to ensure your Django application remains secure:
1. Use Django’s check
Framework
Django’s check
framework can identify some security misconfigurations. Running python manage.py check --deploy
provides warnings about settings that might be insecure in a production environment, including HTTPS-related settings.
2. Leverage Automated Security Scanners
Automated security scanners, such as OWASP ZAP or Qualys SSL Labs, can test your application for common vulnerabilities and misconfigurations related to HTTPS. These tools provide insights and recommendations for improving your security posture.
3. Conduct Regular Code Reviews
Code reviews are an effective way to catch security issues that automated tools might miss. Look for hard-coded sensitive information, improper handling of SSL/TLS settings, and ensure all third-party packages used by your Django application are up to date and secure.
Conclusion
Implementing HTTPS in Django is not just about activating a setting; it’s about consistently ensuring your web application’s security through best practices and regular audits. By following the guidelines outlined in this post—from configuring Django’s security settings to obtaining an SSL/TLS certificate, and from enforcing secure cookies to conducting thorough security audits—you can significantly enhance the security of your Django application. Remember, security is an ongoing process, not a one-time setup. Stay informed about the latest security trends and updates to keep your application and its users safe.
For more detailed information on Django’s security practices, visit the official Django documentation at Django Project Security. Additionally, exploring resources such as OWASP’s Top 10 Web Application Security Risks can provide further insights into common vulnerabilities and how to mitigate them.
By prioritizing HTTPS and following these best practices, you contribute to a safer internet, protecting both your application and its users from potential threats.