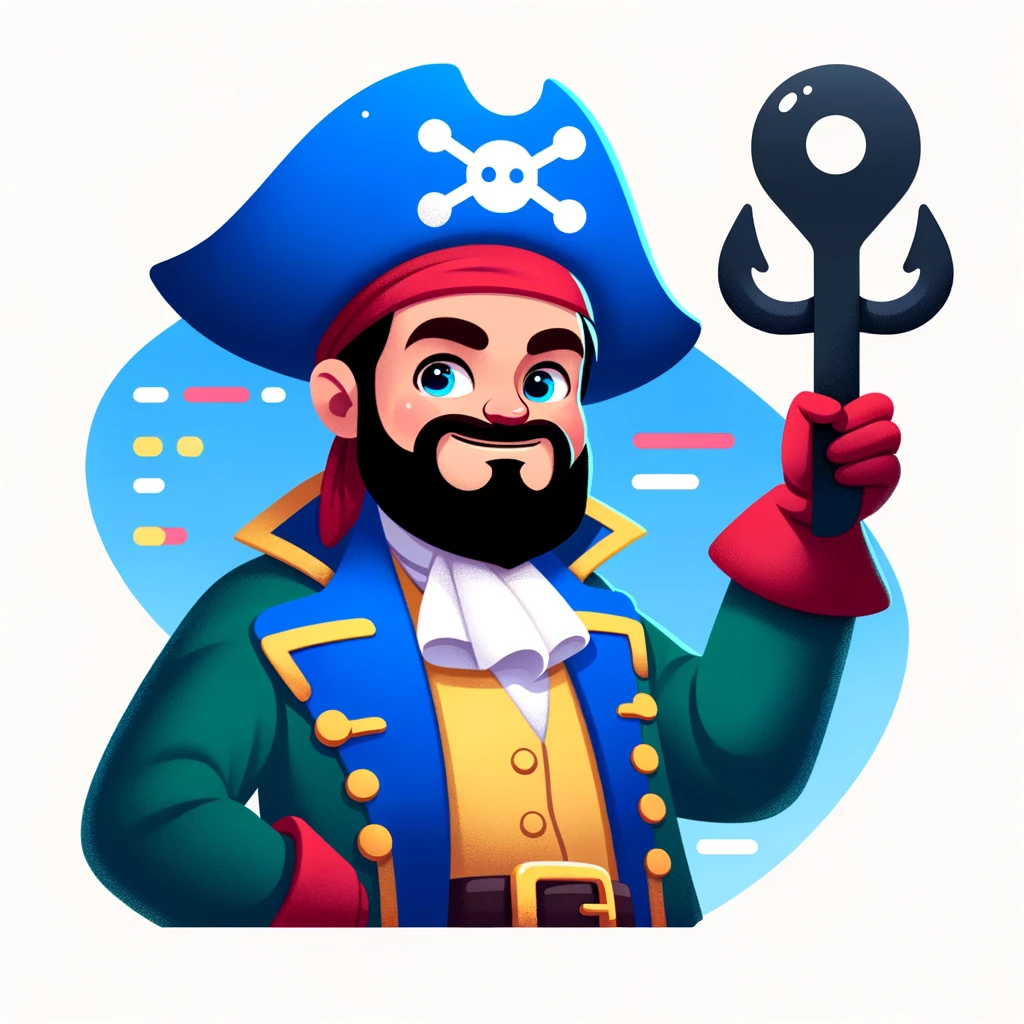
React, a popular JavaScript library for building user interfaces, offers a powerful feature called hooks. Hooks allow you to use state and other React features without writing a class. In this post, we’ll dive into creating your own React hooks, focusing on a custom hook for adding and removing event listeners. This approach not only enhances the readability and maintainability of your code but also encapsulates complex logic, making it reusable across components.
Understanding the Basics of Custom Hooks
Before we delve into our example, it’s crucial to grasp what custom hooks are and why they’re beneficial. A custom hook is essentially a JavaScript function whose name starts with “use” and that can call other hooks. It’s a mechanism to reuse stateful logic, not state itself, allowing you to extract component logic into reusable functions. React provides several built-in hooks like useState
, useEffect
, and useCallback
. Custom hooks leverage these built-in hooks to encapsulate and reuse logic across components.
Why Create a Custom Hook for Event Listeners?
Managing event listeners in a React component can get complicated, especially when it comes to adding and removing them. A custom hook can encapsulate this logic, making your components cleaner and more focused on rendering.
Crafting a Custom Hook for Event Listeners
Let’s create a custom hook named useEventListener
. This hook will handle the addition and removal of an event listener, ensuring that the listener is removed when the component unmounts, which is crucial for preventing memory leaks.
import { useEffect } from 'react';
function useEventListener(eventName, handler, element = window) {
useEffect(() => {
// Ensure the element supports addEventListener
const isSupported = element && element.addEventListener;
if (!isSupported) return;
// Add event listener
element.addEventListener(eventName, handler);
// Remove event listener on cleanup
return () => {
element.removeEventListener(eventName, handler);
};
}, [eventName, element, handler]);
}
Usage of useEventListener
To use this hook, you simply call it within a functional component, passing the event name and the handler function you want to execute.
function MyComponent() {
// NOTE: you ideally want to memoize this handler with something like
// useCallback to prevent repeatedly adding/removing the event listener.
const logScroll = () => console.log('window scrolled');
useEventListener('scroll', logScroll);
return <div>Content</div>;
}
In this example, useEventListener
is used to add a scroll listener to the window object.
Best Practices When Implementing Custom Hooks
Naming Convention: Start the name with
use
to indicate it’s a hook and follow with a name that describes its purpose, likeuseEventListener
.Encapsulation: Encapsulate only one piece of logic per hook. This enhances reusability and keeps your custom hook focused.
Minimize State: If your hook needs to manage state with
useState
, try to keep the state minimal. This ensures that the hook remains focused and reusable.Dependencies Array: Pay close attention to the dependencies array in the
useEffect
hook. It should include all variables used inside the effect to ensure it runs correctly when dependencies change.Testing: Write unit tests for your custom hooks. This ensures they work correctly and remain maintainable.
Documentation: Comment your custom hooks, explaining what they do and how to use them. This is invaluable for future you and other developers who might use your hook.
Conclusion
Creating custom hooks in React not only streamlines your code but also encapsulates and reuses logic across your components. The useEventListener
hook we created is a practical example of how you can manage event listeners efficiently. Remember, the key is to keep your hooks focused, well-documented, and tested. This practice leads to cleaner, more maintainable, and reusable code, enhancing your React applications' overall quality.
For further reading and more advanced practices, the React documentation on Hooks is an excellent resource.