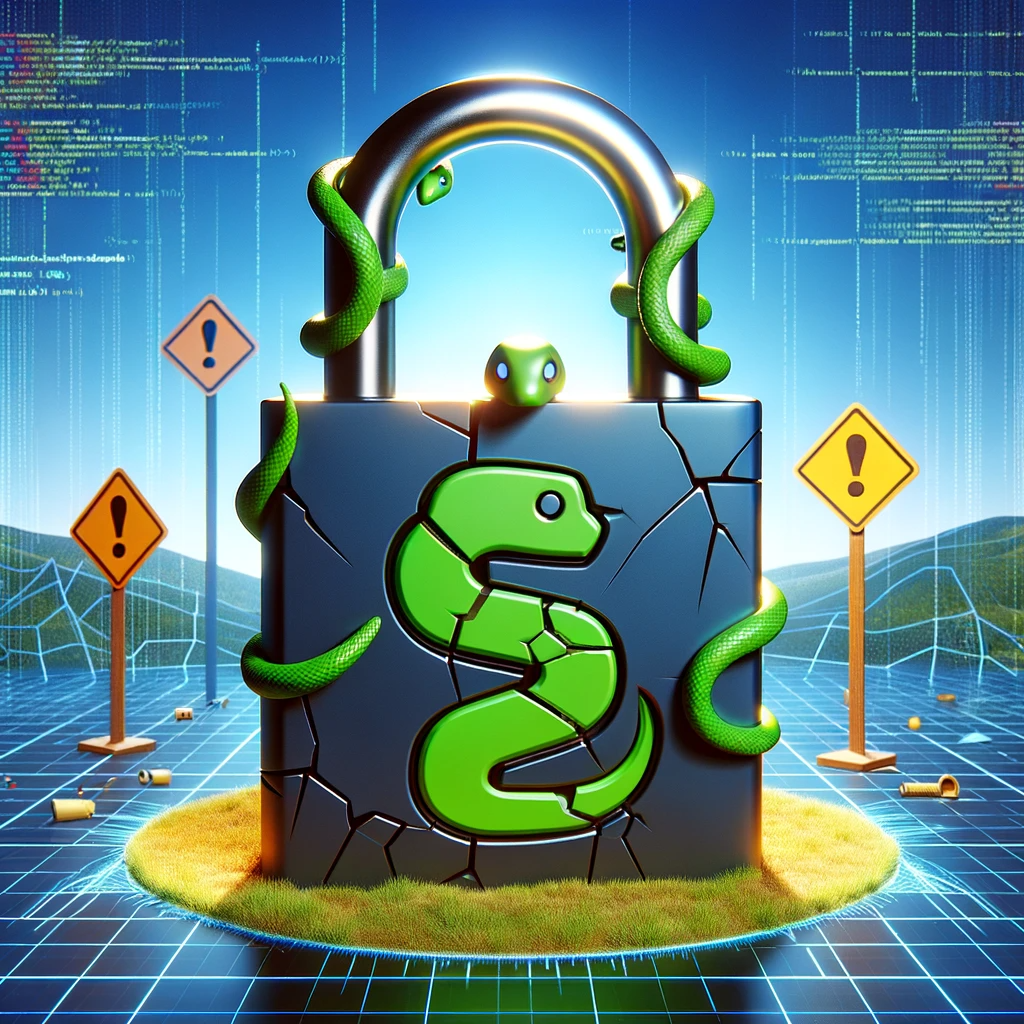
In the world of web development, security is a paramount concern, especially when it comes to handling sensitive data. One common vulnerability often overlooked is the CWE-328, or the Reversible One-Way Hash. This weakness occurs when a system designed to create one-way hashes (hashing algorithms that turn data into a fixed-size string of characters) implements them in a way that makes them reversible, or decodable, defeating their primary purpose of securing data.
Understanding CWE-328 in the Context of Django
Django, a high-level Python Web framework, is widely used for rapid development and clean, pragmatic design. However, even in Django applications, security pitfalls such as reversible hashes can exist, particularly when developers create custom authentication or data protection mechanisms without fully understanding the implications of their hashing choices.
Common Causes in Django
- Use of Weak Hashing Algorithms: Older or less secure hashing algorithms like MD5 or SHA1, which are more susceptible to collision attacks, can make hashes reversible.
- Improper Salting Techniques: Salting is the process of adding unique data to each hashed password. Incorrect salting or using predictable salts can compromise the hash’s security.
- Custom Hash Functions: Sometimes developers try to create their own hash functions, which often leads to insecure implementations.
Identifying CWE-328 in Django
To identify a reversible one-way hash in a Django application, look for the following:
- Hashing Algorithm Used: Review the code to see if it uses known weak hashing algorithms. In Django, this can often be found in the settings.py file or in custom user models.
- Custom Hash Functions: Look for any homemade hash functions or modifications to existing ones.
- Salting Procedures: Check how salts are applied. They should be unique and unpredictable - ideally using a cryptographically secure random number generator, such as Python’s
os.urandom()
function.
Example of Vulnerable Code
Let’s consider an example of a Django model that uses a custom method to hash data:
import hashlib
class User(models.Model):
username = models.CharField(max_length=100)
password = models.CharField(max_length=100)
def set_password(self, raw_password):
self.password = hashlib.md5(raw_password.encode()).hexdigest()
In this snippet, the set_password
method uses the MD5 hashing algorithm, which is a common red flag for reversible hashes.
Remediation Strategies
The key to remediation lies in understanding and implementing secure hashing practices. Here’s how you can address the issues in Django:
Switch to Stronger Hashing Algorithms
Using stronger, non-reversible hashing algorithms is critical. Django supports PBKDF2 and bcrypt, which are much more secure than MD5 or SHA1.
Implementation
To use PBKDF2, you can modify your Django settings:
PASSWORD_HASHERS = [
'django.contrib.auth.hashers.PBKDF2PasswordHasher',
'django.contrib.auth.hashers.PBKDF2SHA1PasswordHasher',
]
This change ensures that Django will use PBKDF2 for password hashing. Learn more about PBKDF2 in our post titled Understanding the Benefits of Key Derivation Functions: A Deep Dive into PBKDF2.
Proper Salting Techniques
Django’s built-in password hashers automatically handle salting in a secure manner. By using these hashers, you ensure that each password hash is properly salted.
Example
Here’s an updated version of the earlier User model using Django’s built-in functionalities:
from django.contrib.auth.models import AbstractBaseUser
class User(AbstractBaseUser):
# ...
def set_password(self, raw_password):
super().set_password(raw_password)
Avoid Custom Hash Functions
Unless you have a deep understanding of cryptography, avoid writing your own hash functions. Stick with Django’s built-in solutions or well-established libraries.
Continuous Vigilance and Best Practices
Regularly updating your Django application and dependencies ensures you benefit from the latest security improvements. Also, code reviews focusing on security can help catch vulnerabilities like CWE-328 early in the development cycle.
Tools and Resources
- Django Documentation: Refer to the official Django documentation for insights on authentication and password management.
- OWASP Guide: The OWASP guide to password storage offers comprehensive best practices.
- Security Auditing Tools: Tools like Bandit or PyUp can scan your Django applications for security issues, including weak hashing implementations.
Conclusion
CWE-328 is a subtle yet critical vulnerability that can compromise the security of your Django applications. By understanding its causes, actively looking for indications of weak hashing, and implementing robust remediation strategies, you can significantly bolster your application’s security posture. Embrace Django’s built-in functionalities, stay updated with the latest security practices, and make code security an integral part of your development process.