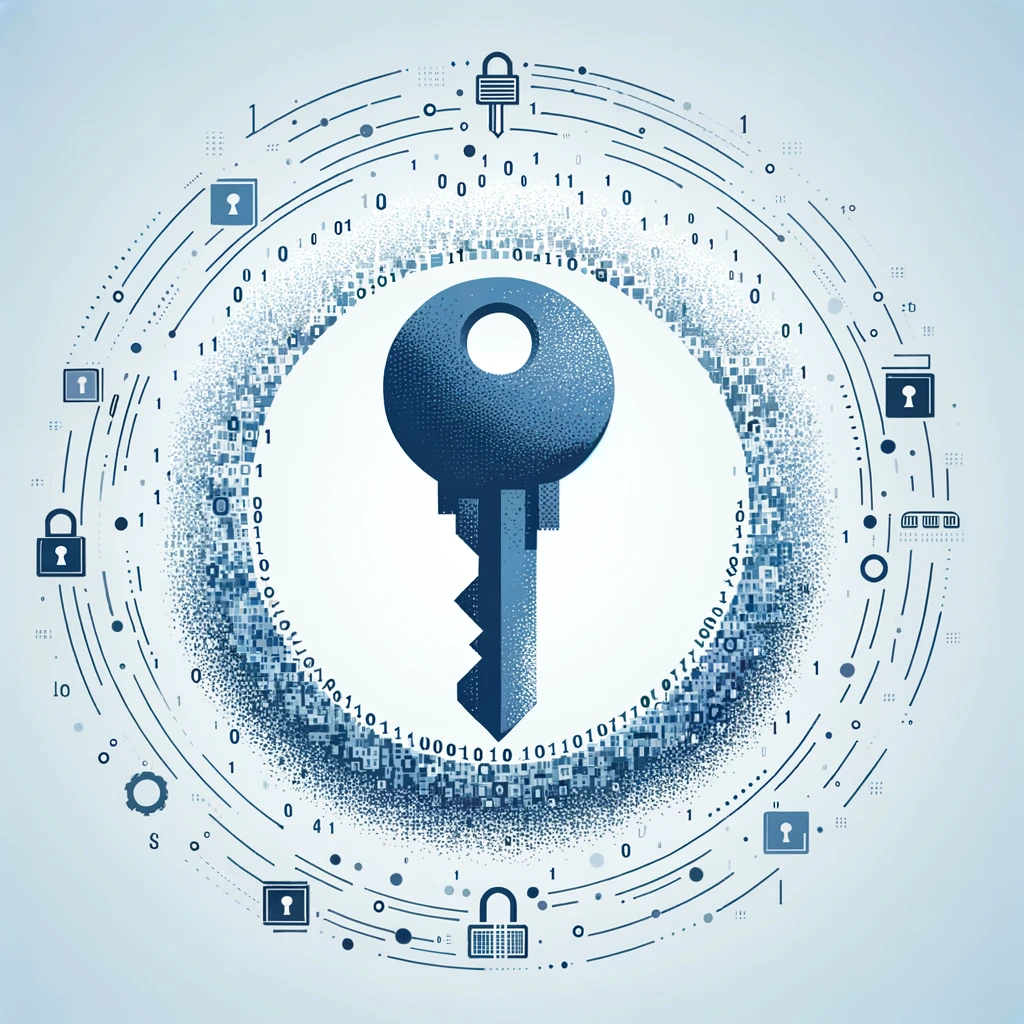
The security of sensitive information within web applications is paramount, especially in today’s digital age where data breaches and cyber threats are on the rise. One common security risk that developers encounter is the Cleartext Storage of Sensitive Information, classified under CWE-312. This vulnerability occurs when sensitive data, such as passwords, personal identification numbers (PINs), or cryptographic keys, is stored in an unprotected, unencrypted form. The Laravel framework, like all other frameworks, is not immune to this risk if not properly handled. In this post, we’ll explore how to identify and remediate CWE-312 in Laravel applications through effective code review and debugging techniques.
Identifying CWE-312 in Laravel
Code Review: The First Line of Defense
Code review is a critical step in identifying vulnerabilities like CWE-312. It involves systematically examining the source code to find errors overlooked in the initial development phase. Here’s what to look for in a Laravel application:
Environment Files: Laravel stores its environment-specific variables in
.env
files. Check if any sensitive information is directly embedded in the codebase instead of being referenced through the.env
file. Sensitive data should never be hard-coded. In addition, these.env
files with sensitive information should never be committed to the code base.Database Migrations and Models: Inspect your migrations and models to ensure that any fields meant to store sensitive information are set up to use encryption. Laravel offers encrypted casting for Eloquent models, which is crucial for fields storing sensitive data.
Logging Configuration: Laravel’s logging capabilities can inadvertently capture sensitive information if not properly configured. Ensure that log levels and channels are set up to exclude sensitive data. Review the configuration in
config/logging.php
to prevent logging sensitive information in cleartext.
Debugging Techniques: Uncovering Hidden Vulnerabilities
Debugging is not just for fixing errors; it can be a proactive tool in identifying security vulnerabilities. Laravel’s debug mode provides detailed error output that can inadvertently reveal sensitive information if enabled in production. Ensure debug mode is disabled in production by setting APP_DEBUG=false
in your .env
file.
Use Laravel’s logging to help identify any instances where sensitive information might be logged or outputted. Temporarily increase log verbosity during development to catch any unintended data exposure. Remember to revert to safer log levels in production.
Remediation Strategies
Encrypting Sensitive Data
To remediate CWE-312, any sensitive information stored must be encrypted. Laravel provides several ways to encrypt data securely:
- Using Laravel’s Encryption Facilities: Laravel offers out-of-the-box encryption through its
Crypt
facade, utilizing the AES-256 and AES-128 encryption algorithms. Use it to encrypt sensitive data before storing it and decrypt it when accessing. Here’s a quick example:
use Illuminate\Support\Facades\Crypt;
$encrypted = Crypt::encryptString('Sensitive Information');
$decrypted = Crypt::decryptString($encrypted);
- Eloquent Attribute Casting: For database fields, use Eloquent’s encrypted casting feature to automatically encrypt and decrypt attributes. This is perfect for storing sensitive data in your database securely. Simply define the attribute casting in your model:
protected $casts = [
'sensitive_field' => 'encrypted',
];
Secure Database Practices
In addition to application-level encryption, ensure that your database is configured to use encrypted connections and that any backups are also encrypted. For MySQL and PostgreSQL, Laravel supports SSL connection options out of the box, which can be configured in your database configuration file config/database.php
.
Auditing and Logging
Proper auditing and logging are essential in monitoring access to sensitive information. However, ensure that the logs themselves do not contain sensitive information in cleartext:
Log::info('User logged in', ['user_email' => $user->email]); // Unsafe
// Avoid logging sensitive information directly
Log::info('User logged in', ['user_id' => $user->id]); // Safe
Laravel also allows for customizing the log channels and what gets logged. Use this feature to filter out sensitive data from logs:
'log_level' => env('APP_LOG_LEVEL', 'info'),
In this way, a user could log some information as “debug” for development purposes, but it won’t reach the production logs since the minimum level is “info”.
Implement regular audits of your logging and database storage practices to ensure compliance with security policies and identify potential areas of improvement.
Best Practices and Preventive Measures
- Regular Code Reviews: Make code reviews a part of your development cycle. They are essential for identifying security vulnerabilities like CWE-312 early in the development process.
- Automated Security Scans: Utilize tools like Laravel Shift, and other PHP security scanners to automatically detect vulnerabilities in your codebase.
- Educate Your Team: Ensure that all team members are aware of the risks associated with storing sensitive information in cleartext and the best practices for securing data.
- Stay Updated: Regularly update your Laravel framework and dependencies to their latest versions to benefit from security patches and improvements.
Conclusion
Protecting sensitive information is a critical responsibility of software developers. In Laravel applications, identifying and remediating the cleartext storage of sensitive information requires vigilance, adherence to best practices, and a proactive approach to security. By conducting thorough code reviews, employing effective debugging techniques, and implementing robust encryption measures, developers can significantly mitigate the risk of CWE-312 and enhance the security of their applications. Remember, security is not a one-time effort but a continuous process of improvement and adaptation to new threats.