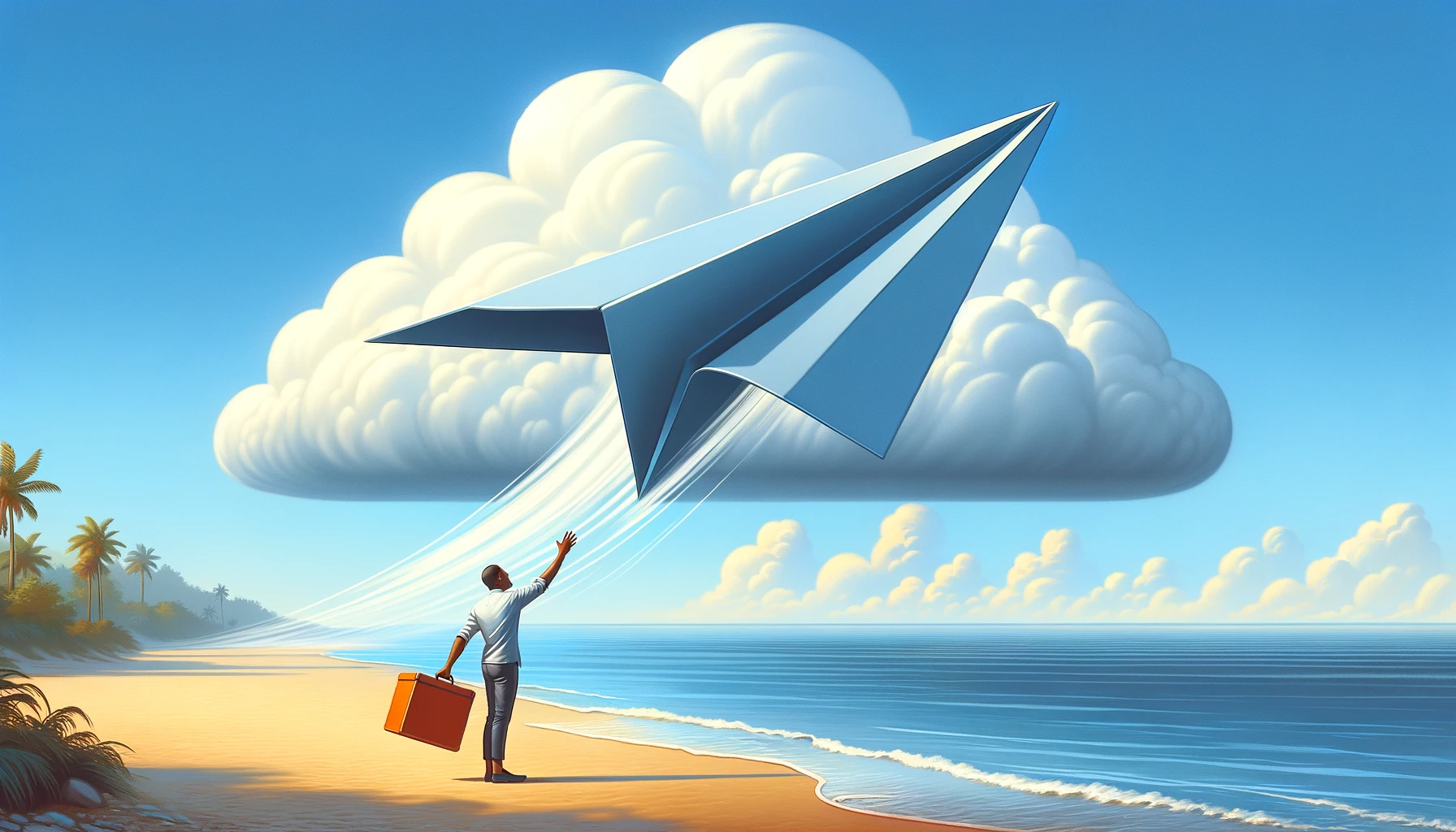
Uploading large files, especially those spanning multiple gigabytes, from a React application can be a challenging task, both in terms of performance and security. A common approach involves using Amazon S3 pre-signed URLs, provided by a backend service. This technique can offload the heavy lifting of direct file transfers to a robust cloud storage service like AWS S3.
Understanding AWS S3 Pre-signed URLs
Pre-signed URLs are a game-changer in secure file uploads. These URLs are generated by your backend, which has authenticated access to your AWS S3 bucket. Each URL is valid for a limited time and allows the client (your React app) to perform a specific action, such as uploading a file, without needing direct AWS credentials. This method keeps your S3 bucket secure, as the access permissions and duration can be tightly controlled.
Generating Pre-signed URLs
Here’s a quick look at generating a pre-signed URL using AWS SDK in a Node.js backend:
const AWS = require("aws-sdk");
// Make sure AWS is configured with your credentials or IAM role
const s3 = new AWS.S3();
function generatePresignedUrl(fileName, fileType) {
// TODO: You should validate filename and type here
const params = {
Bucket: "YOUR_BUCKET_NAME",
Key: fileName,
Expires: 60, // Expires in 60 seconds
ContentType: fileType,
ACL: "bucket-owner-full-control",
};
return s3.getSignedUrl("putObject", params);
}
Implementing in React
With your backend ready to provide pre-signed URLs, your React application can now securely upload large files. Here’s a simplified example:
import React, { useState } from "react";
import axios from "axios";
function FileUploader() {
const [file, setFile] = useState(null);
const handleFileChange = (event) => {
setFile(event.target.files[0]);
};
const uploadFile = async () => {
if (!file) return;
try {
// Request a pre-signed URL from your backend
const response = await axios.get(
`https://your-backend.com/presigned-url?${new URLSearchParams({
fileName: file.name,
fileType: file.type,
})}`
);
const { url } = response.data;
// Upload the file using the pre-signed URL
await axios.put(url, file, {
headers: {
"Content-Type": file.type,
},
});
alert("File uploaded successfully!");
} catch (error) {
console.error("Error uploading file:", error);
}
};
return (
<div>
<input type="file" onChange={handleFileChange} />
<button onClick={uploadFile}>Upload</button>
</div>
);
}
export default FileUploader;
Security Considerations
Client-side Validation: Always validate the file type and size on the client side to prevent unnecessary network traffic and server load.
HTTPS: Always use HTTPS for communication. This prevents man-in-the-middle attacks and keeps the pre-signed URL secure while in transit.
URL Expiration: Keep the pre-signed URL expiration time as short as possible. This limits the window in which an exposed URL could be misused.
Logging and Monitoring: Implement logging on your server for the generation of pre-signed URLs. Monitoring these logs helps in identifying and responding to any unusual activity.
CORS Configuration: Configure CORS on your S3 bucket appropriately. It should only allow requests from your domain to prevent unauthorized cross-domain requests.
Enhancing Performance
For very large files, consider implementing a chunked upload mechanism. This splits the file into smaller chunks, uploading them in sequence or parallel, and can resume if the upload is interrupted. AWS S3 supports multipart uploads, which is ideal for this scenario.
Conclusion
Uploading large files in a React application, using AWS S3 pre-signed URLs, provides a secure and efficient way to handle file transfers. By offloading the actual file transfer to AWS S3, you reduce the load on your server, and by using pre-signed URLs, you maintain a high level of security. Always remember to balance security with usability to ensure a smooth user experience.
For further reading, check out the AWS SDK documentation and the React documentation. Happy coding!