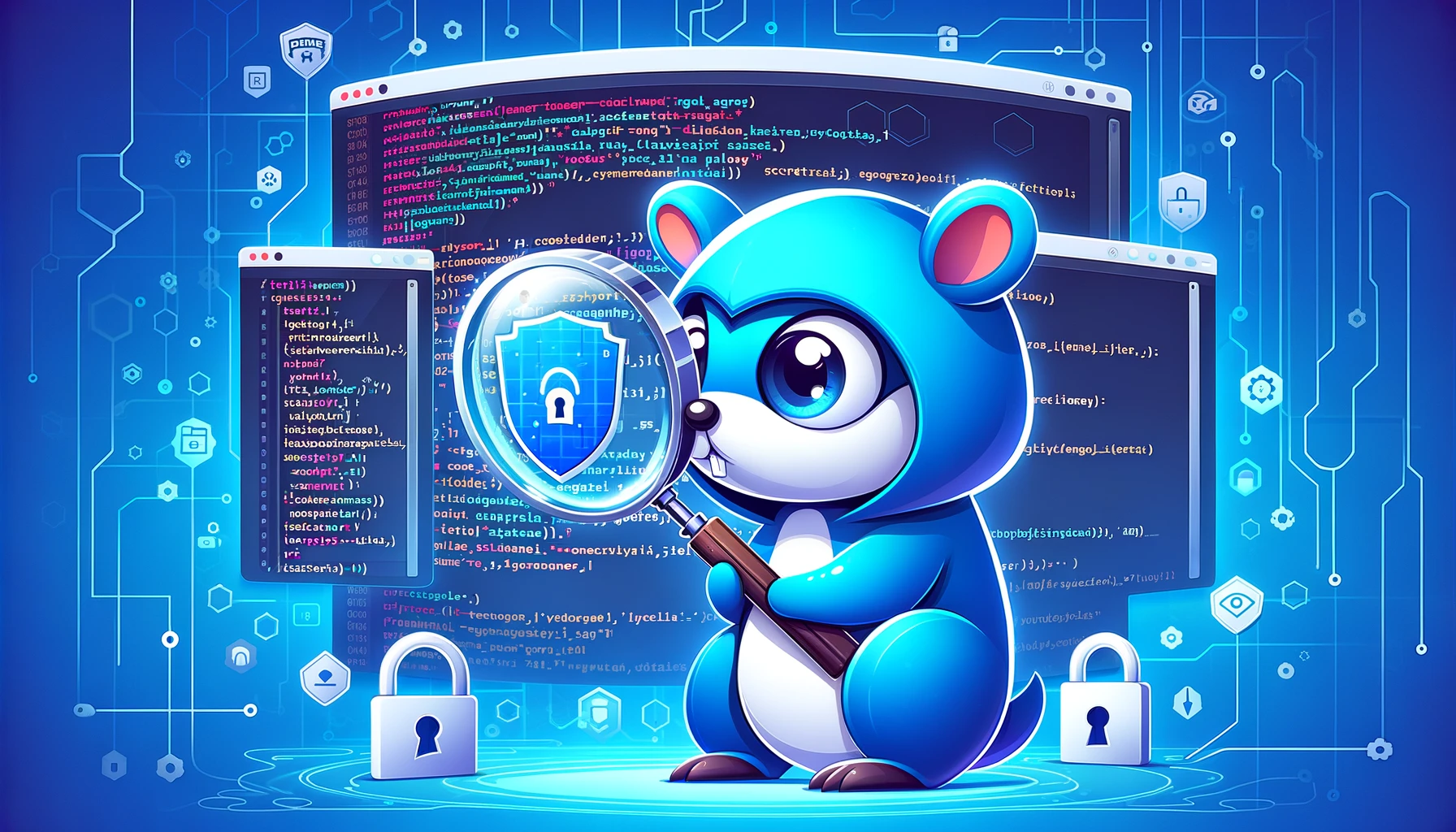
Golang, known for its simplicity and efficiency, often catches developers off guard with its unique approach to error handling. While error handling is a fundamental aspect of robust software development, in Golang, it takes on a heightened significance due to the language’s design of returning multiple values, including errors. This characteristic, while powerful, can lead to subtle yet significant security vulnerabilities, especially among developers transitioning from languages with different error handling paradigms.
Understanding the Issue
In Golang, functions can return multiple values, typically including an error as the last value. This design encourages explicit error checking. However, developers, particularly those new to Golang, might overlook or intentionally ignore these errors. Such practices can lead to unhandled exceptions and, more critically, to security loopholes that attackers can exploit.
A Common Oversight
Consider a scenario where a function returns a crucial error that goes unchecked:
func fetchUserDetails(id string) (*User, error) {
// Database fetch operation...
}
user, _ := fetchUserDetails(userID)
In this snippet, the error returned by fetchUserDetails
is ignored. While this might seem harmless, it could lead to scenarios where user
is nil
or contains incorrect data, potentially leading to unauthorized access or data corruption.
A Security Perspective
To understand how this can become a security issue, let’s delve into a more nuanced example:
Example: Ignoring Authentication Errors
Imagine a function that authenticates users:
func authenticateUser(username, password string) (bool, error) {
// Authentication logic...
}
// In the calling code
authenticated, _ := authenticateUser(inputUsername, inputPassword)
if authenticated {
// Grant access
}
Here, if authenticateUser
encounters an error (e.g., database connectivity issues) and can’t verify the user, it might return false
along with an error. Ignoring this error could lead to the assumption that the user is not authenticated when, in reality, the authentication process didn’t complete. An attacker could exploit this by triggering errors, bypassing authentication mechanisms.
Best Practices for Secure Error Handling
Always Check Errors: Treat every error as a potential failure point. Logically handle errors to prevent unintended behavior.
Use Custom Error Types: Define custom error types for better error categorization and handling strategies. See Dave Cheney’s article for more details.
Fail Securely: Design your application to fail securely in case of errors. For instance, deny access or halt operations if critical errors occur.
Log and Monitor Errors: Implement comprehensive logging for errors and monitor logs for unusual patterns or frequent error occurrences.
Code Reviews and Static Analysis: Utilize code reviews and static analysis tools to detect and rectify unhandled errors.
Conclusion
In conclusion, improper error handling in Golang, while often perceived as a general coding oversight, can open doors to security vulnerabilities. This issue is particularly acute for developers not accustomed to Golang’s error handling model. By recognizing the security implications of unhandled errors and adopting robust error handling practices, developers can significantly bolster the security and reliability of their Golang applications. Remember, in the world of software security, the devil often lies in the details.