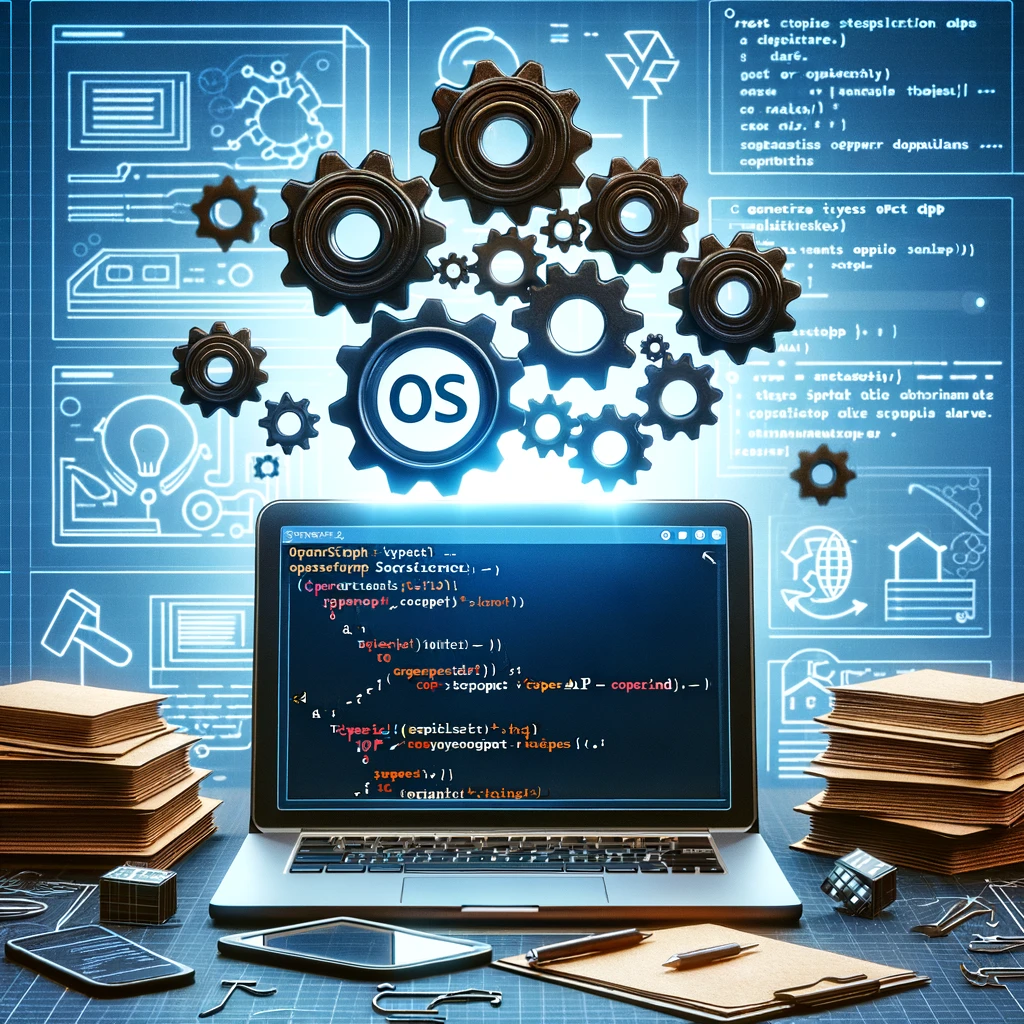
In the realm of modern web development, consuming REST APIs efficiently and securely is paramount. TypeScript, with its static typing system, plays a crucial role in ensuring type safety and enhancing developer productivity. Coupled with OpenAPI (formerly known as Swagger), developers can automate the generation of TypeScript types, ensuring that the contract between frontend and backend services is accurately represented and enforced. This synergy not only accelerates development cycles but also significantly reduces runtime errors and debugging time.
Leveraging OpenAPI for Type Safety
OpenAPI Specification (OAS) provides a powerful standard for describing RESTful APIs. By defining endpoints, parameters, and response objects in a machine-readable format, OpenAPI enables tools to generate client code, documentation, and even test cases automatically. For TypeScript developers, the most enticing capability is the generation of interface definitions directly from an API specification, ensuring that the data structures used in the application match those expected by the API.
Why Type Generation Matters
Before diving into the how, let’s understand the why. Manually writing TypeScript interfaces for complex API responses is tedious and error-prone. As APIs evolve, keeping these interfaces in sync becomes a challenge. Automated type generation from OpenAPI specifications mitigates these issues by:
- Ensuring Consistency: Automatically generated types reflect the latest API specification.
- Saving Time: Reduces manual coding, especially for large and complex APIs.
- Improving Developer Experience: Provides IntelliSense support in IDEs, making API discovery easier and reducing errors.
- Enhancing Code Quality: Early detection of potential mismatches between the frontend and backend.
Getting Started with OpenAPI and TypeScript
Assuming you have an OpenAPI specification for your REST API, the next step is to generate TypeScript types. Tools like openapi-generator
or swagger-codegen
can automate this process. For this guide, we’ll focus on openapi-generator
, which supports a wide range of languages and frameworks, including TypeScript.
Step 1: Installing OpenAPI Generator
First, install the OpenAPI Generator CLI. You can do this via npm:
npm install @openapitools/openapi-generator-cli -g
Step 2: Generating TypeScript Interfaces
With the CLI installed, you can now generate TypeScript types. Navigate to the directory where your OpenAPI specification file (api-spec.yaml
or api-spec.json
) is located and run:
openapi-generator-cli generate -i api-spec.yaml -g typescript-axios -o ./api-client
This command tells the generator to create a TypeScript client using the Axios HTTP client library. The -i
flag specifies the input OpenAPI specification file, -g
sets the generator to use, and -o
defines the output directory for the generated code.
Customizing the Output
The OpenAPI Generator provides numerous options to tailor the output to your needs. For instance, you can specify custom model prefixes, choose different HTTP libraries (like fetch
), and even control the generation of enums and interfaces. Explore the CLI’s help section or the official documentation for a comprehensive list of options:
openapi-generator-cli help generate
Integrating Generated Types into Your Project
Once the types are generated, integrate them into your TypeScript project by importing the generated modules where you make API calls. For example:
import { UsersApi, User } from './api-client';
const usersApi = new UsersApi();
async function fetchUser(userId: string): Promise<User> {
const response = await usersApi.getUserById(userId);
return response.data;
}
This approach provides strong typing for API calls and responses, significantly reducing the risk of runtime errors and making the code easier to understand and maintain.
Keeping Types Up-to-Date
APIs evolve, and so should your TypeScript types. Automate the regeneration of types as part of your continuous integration (CI) pipeline to ensure they stay in sync with the API specification. This can be done by including the OpenAPI Generator command in your build scripts or CI configuration.
Advanced Techniques and Tools
Using OpenAPI Tools for React and Vue
For developers working with React or Vue, there are tools specifically designed to enhance the experience of using OpenAPI with these frameworks. For example, openapi-generator-plus
and plugins for popular build tools can streamline the generation process and integrate more seamlessly into your existing workflow.
Handling API Evolution Gracefully
As APIs change, maintaining backward compatibility and managing versioning become critical. Semantic versioning of your API and OpenAPI specification can help frontend and backend teams coordinate updates more effectively. Additionally, leveraging tools that can diff OpenAPI specifications can alert you to breaking changes before they impact your application.
Best Practices for API Design
A well-designed API and OpenAPI specification are the foundations of a successful integration. Follow best practices for RESTful design and OpenAPI documentation to ensure that your API is easy to consume and the generated TypeScript types are as useful and accurate as possible. This includes using meaningful endpoint names, accurately describing request and response schemas, and meticulously annotating your API with relevant metadata.
Conclusion
Automating the generation of TypeScript types from OpenAPI specifications streamlines the development process, enhances code quality, and ensures a seamless integration between frontend and backend systems. By leveraging the tools and techniques discussed, developers can enjoy a more productive and error-free coding experience. As APIs continue to evolve, embracing automation and adhering to best practices in API design and documentation will remain critical components of modern web development.
Additional Resources
- OpenAPI Specification: The official OpenAPI Specification documentation is the definitive source for understanding how to effectively utilize OpenAPI in designing and documenting your APIs.
- OpenAPI Generator: The OpenAPI Generator project offers extensive documentation on how to generate client libraries, server stubs, API documentation, and other useful components from an OpenAPI Specification.
- TypeScript Documentation: The TypeScript official documentation provides insights into TypeScript’s features, including its type system and how to use TypeScript for developing large-scale JavaScript applications.
- Axios: A promise-based HTTP client for the browser and Node.js. The Axios documentation offers examples and guidance on making HTTP requests from JavaScript and TypeScript code.
- API Design Best Practices: Articles and guides on API design best practices can help in creating APIs that are easy to use and maintain, and which align well with the OpenAPI specifications for automated client generation.
- API Design Guide by Google: Google Cloud API Design Guide
- Microsoft REST API Guidelines: Microsoft REST API Guidelines