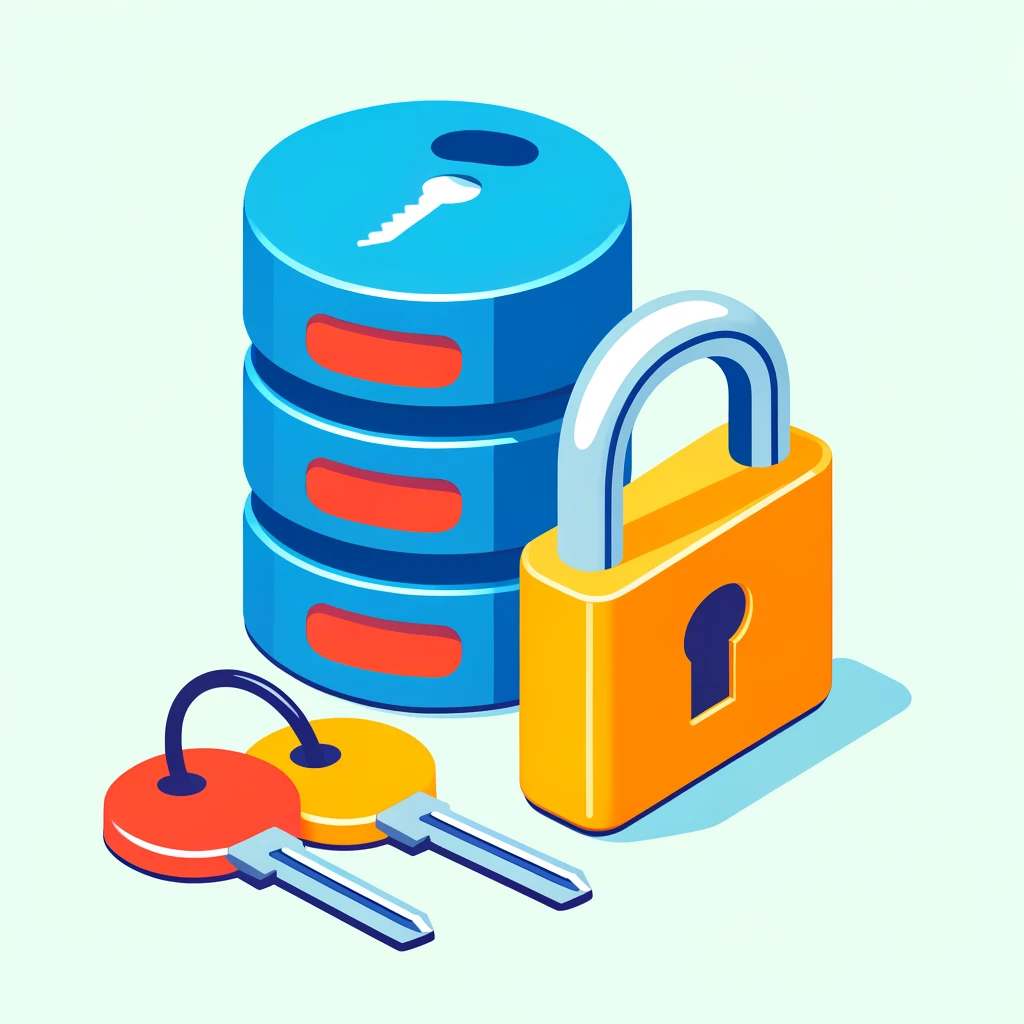
In the realm of modern software development, where data integrity and consistency are paramount, leveraging the full spectrum of features offered by database services is crucial. Among these, Amazon DynamoDB stands out for its fully managed NoSQL database service, providing predictable performance with seamless scalability. A new feature added last year within DynamoDB’s arsenal is the ReturnValuesOnConditionCheckFailure
option. This feature plays a pivotal role in enhancing data handling operations, particularly in ensuring that applications behave predictably and data integrity is maintained during conditional write operations.
Understanding Conditional Writes
Before delving into the specifics of ReturnValuesOnConditionCheckFailure
, it’s essential to grasp the concept of conditional writes in DynamoDB. Conditional writes allow you to specify conditions that must be met for the write operation (PutItem, UpdateItem, DeleteItem) to succeed. This capability is invaluable for maintaining data integrity, as it ensures that operations only occur when the application’s logic requirements are met.
For instance, you might use a conditional write to update an item only if it exists or has a specific attribute value. This prevents unwanted data overwrites and ensures that updates are made based on the current state of the data, aligning with application logic.
The Role of ReturnValuesOnConditionCheckFailure
The ReturnValuesOnConditionCheckFailure
parameter comes into play when a conditional write fails to meet the specified condition. Normally, if a condition is not met, DynamoDB simply returns a ConditionalCheckFailedException
, and the matched row is not provided. However, by using ReturnValuesOnConditionCheckFailure
, developers can instruct DynamoDB to return the item’s attributes as they were before the failed write attempt. This feature supports two settings:
ALL_OLD
: Returns all attributes of the item as they were before the attempted write.NONE
: No data is returned. This is the default behavior.
Practical Use Cases
The utility of ReturnValuesOnConditionCheckFailure
becomes evident in complex applications where understanding why a conditional write failed is crucial for maintaining data integrity and providing contextual feedback to the user or for logging purposes. Here are a few scenarios where it proves invaluable:
- Optimistic Locking:
ReturnValuesOnConditionCheckFailure
is particularly useful in implementing optimistic locking patterns, where a write operation includes a condition based on a version number or timestamp. If the write fails, the returned data can be used to inform the user of the specific version conflict, facilitating a more informed resolution process. - Debugging and Logging: By returning the item’s previous state on a condition check failure, developers can log detailed information about the failure context, making it easier to debug issues related to conditional writes.
- User Feedback: In user-facing applications, understanding the state of an item that led to a conditional write failure can help in providing more informative feedback to the user. For instance, if an update fails because an item was already updated by another process, the application can alert the user to the latest state and possibly suggest alternative actions.
Code Example: Implementing ReturnValuesOnConditionCheckFailure
import boto3
from botocore.exceptions import ClientError
# Initialize a DynamoDB client
dynamodb = boto3.client('dynamodb')
try:
response = dynamodb.update_item(
TableName='YourTableName',
Key={
'PrimaryKey': {'S': 'YourPrimaryKeyValue'}
},
UpdateExpression='SET #attr = :val',
ConditionExpression='attribute_exists(YourConditionAttribute)',
ExpressionAttributeNames={
'#attr': 'YourAttributeToUpdate'
},
ExpressionAttributeValues={
':val': {'S': 'NewValue'}
},
ReturnValuesOnConditionCheckFailure='ALL_OLD'
)
except ClientError as e:
if e.response['Error']['Code'] == 'ConditionalCheckFailedException':
# Handle the condition check failure
print("Condition check failed. Item before failure:", e.response['Attributes'])
else:
# Handle other exceptions
raise
This example attempts to update an item in a table, provided a specific condition is met. If the condition fails, it captures the item’s state before the failure, offering insight into why the operation did not proceed as expected.
Conclusion
The ReturnValuesOnConditionCheckFailure
option in DynamoDB is a testament to the depth and flexibility offered by AWS’s NoSQL service. By allowing developers to retrieve the state of an item before a conditional write operation fails, it opens up possibilities for more robust error handling, debugging, and user feedback mechanisms. As applications grow in complexity and the demands on data integrity increase, features like ReturnValuesOnConditionCheckFailure
ensure that developers have the tools they need to build resilient and user-friendly applications.