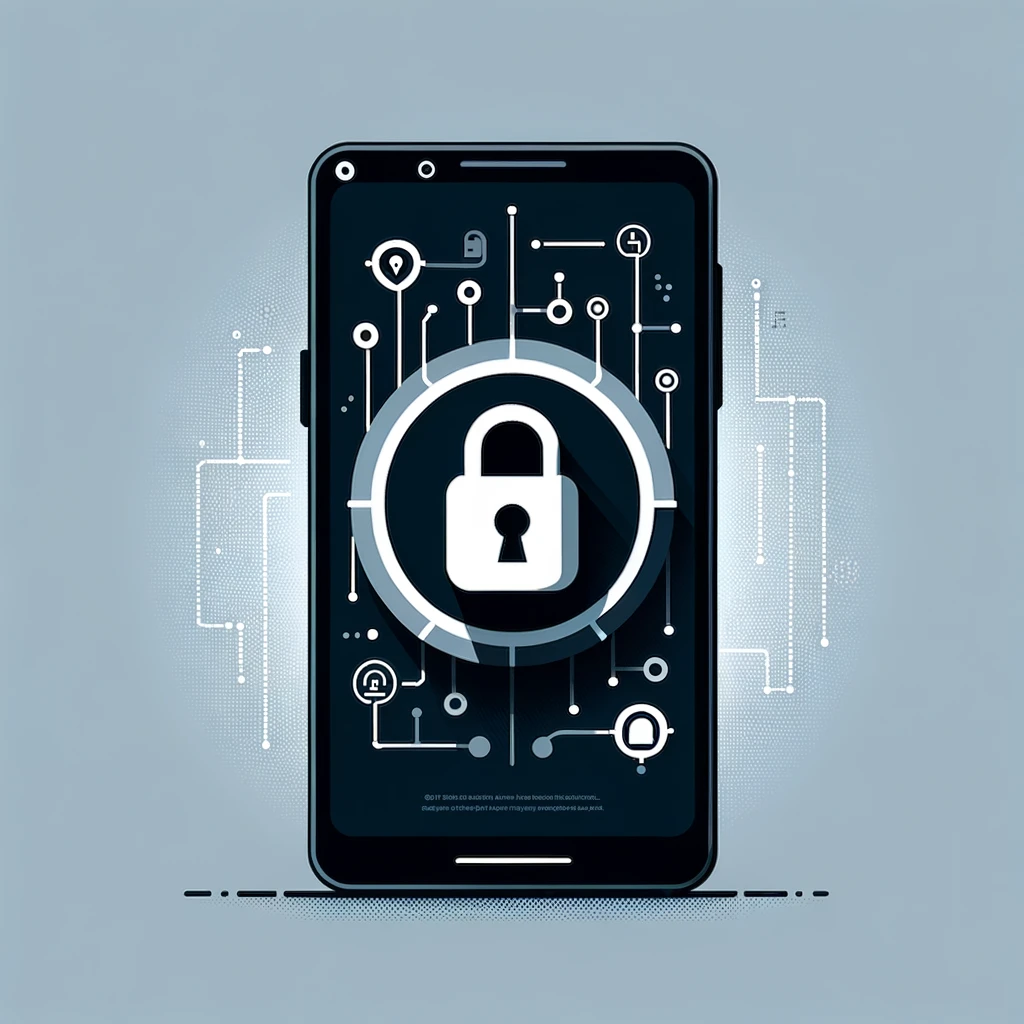
In the rapidly evolving world of mobile app development, security remains a paramount concern. With the rise of frameworks like Expo, which streamline the development process for React Native applications, it’s crucial to integrate robust security practices from the get-go. Expo, known for its efficiency and ease of use, does provide a solid foundation, but it’s the developer’s responsibility to ensure that the application is not just functional but also secure. In this blog, we’ll dive into some key strategies for fortifying your mobile application’s security when working with Expo.
Understanding the Expo Ecosystem
Before delving into security specifics, it’s important to have a grasp of what Expo brings to the table. Expo is a framework and platform for universal React applications. It’s a set of tools and services built around React Native and native platforms that help you develop, build, deploy, and quickly iterate on iOS, Android, and web apps from the same JavaScript/TypeScript codebase.
Secure Storage: Handling Sensitive Data
One of the first considerations in mobile app security is how you handle sensitive data. In the context of Expo, you have access to expo-secure-store
, which provides a way to encrypt and securely store key-value pairs locally on the device. This is ideal for storing tokens, passwords, and other sensitive information that shouldn’t be kept in plain text.
Example: Using Expo Secure Store
import * as SecureStore from "expo-secure-store";
async function save(key, value) {
await SecureStore.setItemAsync(key, value);
}
async function getValueFor(key) {
let result = await SecureStore.getItemAsync(key);
if (result) {
alert("🔐 Here's your value 🔐 \n" + result);
} else {
alert("No values stored under that key.");
}
}
This code snippet demonstrates how to securely store and retrieve data. Remember, while expo-secure-store
encrypts the data, managing how the keys are handled in your app is still crucial.
Network Security: Safe API Communication
In mobile apps, data is frequently exchanged between the app and a server. This data transfer needs to be secured to prevent interception and tampering. HTTPS should be the baseline for all your API communication. Additionally, consider implementing certificate pinning to mitigate the risk of man-in-the-middle attacks. While Expo doesn’t support certificate pinning out of the box, you can eject to ExpoKit or use a third-party library.
Libraries and Tools
- Axios: A promise-based HTTP client for making HTTP requests, check out the Axios documentation for secure usage.
- React Native SSL Pinning: Can be integrated into ejected Expo projects for certificate pinning. Learn more about it here.
Authentication and Authorization: Secure User Access
Implementing robust authentication and authorization mechanisms is absolutely necessary. For Expo apps, you might consider OAuth 2.0 for handling authentication. Expo conveniently provides expo-auth-session
to help streamline this process.
Example: Implementing OAuth with Expo
Expo simplifies OAuth implementation. Here’s a basic setup for authenticating with Google:
import * as AuthSession from "expo-auth-session";
const useGoogleAuthentication = () => {
const discovery = AuthSession.useAutoDiscovery("https://accounts.google.com");
const [request, response, promptAsync] = AuthSession.useAuthRequest(
{
clientId: "GOOGLE_CLIENT_ID",
scopes: ["openid", "profile"],
redirectUri: "https://your-redirect-uri",
},
discovery
);
useEffect(() => {
if (response?.type === "success") {
const { authentication } = response;
// Handle successful authentication. For example, store the token securely
// and use it for subsequent API requests.
}
}, [response]);
return promptAsync;
};
This example demonstrates how to initiate an OAuth session using Expo’s AuthSession
. Always ensure that your redirect URIs are correctly configured and your OAuth client ID is securely stored. You can use tools like expo-secure-store
as shown earlier to store sensitive information.
Regular Updates and Dependency Management
Keeping your Expo SDK and all dependencies up to date is vital for security. Regularly update to the latest versions, as they often include security patches and improvements. Use tools like npm audit
or yarn audit
to identify and fix vulnerabilities in your dependencies.
Final Thoughts: A Proactive Approach to Security
Security in mobile app development is not a one-off task but a continuous process. When working with frameworks like Expo, it’s essential to stay informed about the latest security best practices and updates. Regularly review your code, dependencies, and Expo SDK updates to ensure that your application remains secure against emerging threats.
For more in-depth information and updates on Expo, visit the Expo documentation. Stay proactive and vigilant, and your mobile application will not only be efficient and user-friendly but also secure.