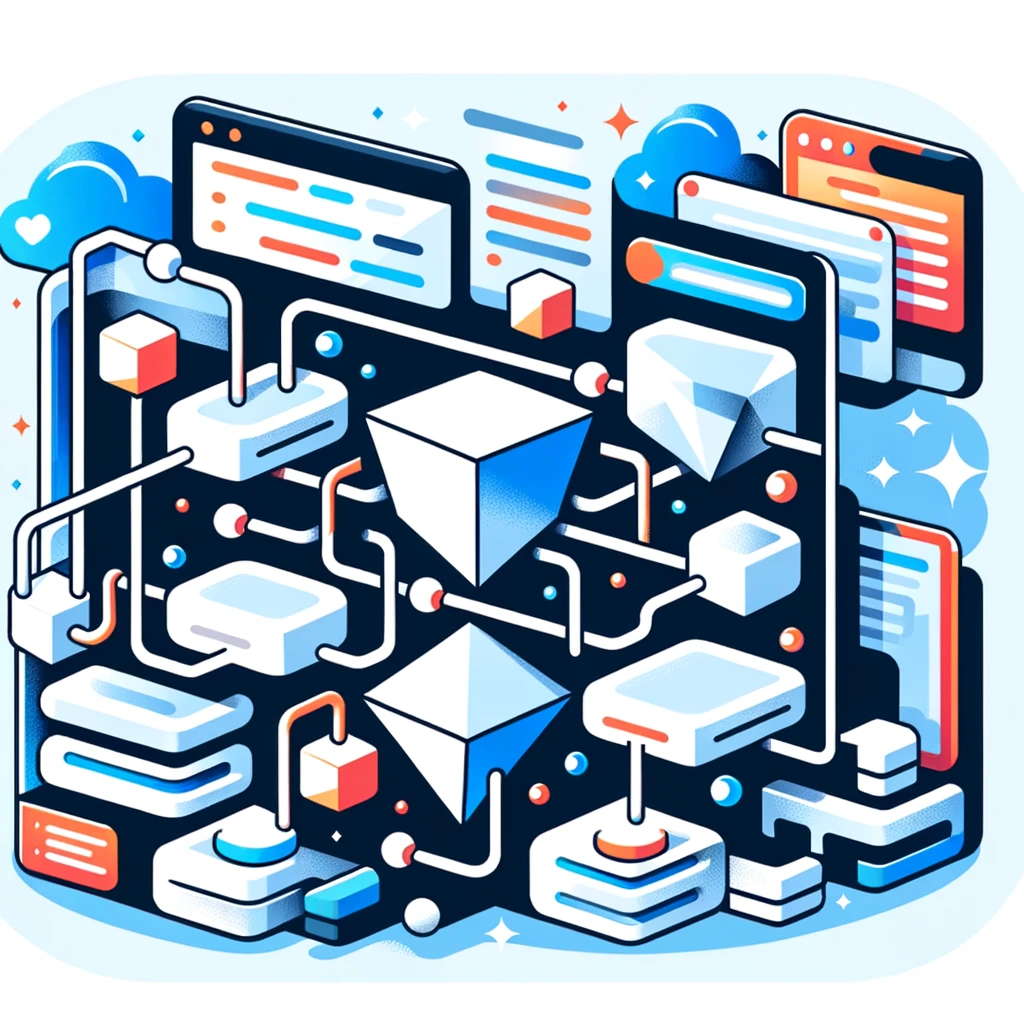
In software development, the principle of designing applications that are both loosely coupled and highly testable is paramount. Dependency Injection (DI) emerges as a silver bullet in achieving these design goals, offering a pathway towards more maintainable, scalable, and robust applications. This blog post delves into the intricacies of utilizing DI in ASP.NET.
The Essence of Dependency Injection in ASP.NET
Dependency Injection is a design pattern pivotal for achieving the Inversion of Control (IoC) design pattern between classes and their instances of dependent objects. By delegating the task of creating these dependencies to an external entity (typically an IoC container), ASP.NET applications can enjoy a higher level of modularity, making them easier to manage, test, and scale.
In ASP.NET Core, DI is a first-class citizen, with built-in support that simplifies the integration of this pattern into your projects. The framework’s IoC container allows developers to register their application’s dependencies at startup, which the framework then injects wherever needed, thus decoupling the creation of an object from its usage.
Achieving Loose Coupling with DI
Loose coupling is a design goal aimed at reducing the interdependencies among components of a system, making each component more isolated and thus easier to refactor, modify, replace, and test without affecting others. Dependency Injection shines in achieving this by providing a way to dynamically inject dependencies at runtime rather than statically at compile time.
Consider an ASP.NET Core application that implements a service layer for data access. Without DI, you might instantiate a repository directly within your service class:
public class ProductService
{
private ProductRepository _repository = new ProductRepository();
}
This design tightly couples the ProductService
to a specific ProductRepository
, making it difficult to switch out the repository implementation or to mock this dependency for testing.
Using DI, you can invert this dependency, making ProductService
agnostic of the ProductRepository
implementation:
public class ProductService
{
private readonly IRepository<Product> _repository;
public ProductService(IRepository<Product> repository)
{
_repository = repository;
}
}
Here, ProductService
depends on an abstraction (IRepository<Product>
) rather than a concrete implementation, allowing the IoC container to inject any class that implements IRepository<Product>
at runtime. This approach decouples the service from the repository, paving the way for more flexible and maintainable code.
Enhancing Testability through DI
Testability is a measure of how easily software can be tested. It’s crucial for maintaining high-quality code, ensuring that changes do not introduce regressions. Dependency Injection enhances testability by allowing developers to easily swap out real dependencies with mocks or stubs during testing.
Using the previous ProductService
example, let’s see how DI facilitates testing by allowing the injection of a mock repository:
public class ProductServiceTests
{
[Fact]
public void TestGetProductById()
{
// Arrange
var mockRepository = new Mock<IRepository<Product>>();
mockRepository.Setup(repo => repo.FindById(1)).Returns(new Product { Id = 1, Name = "Test Product" });
var productService = new ProductService(mockRepository.Object);
// Act
var product = productService.GetProductById(1);
// Assert
Assert.Equal("Test Product", product.Name);
}
}
In this test, we use Moq (a popular mocking library for .NET) to create a mock implementation of IRepository<Product>
. This mock is then injected into ProductService
, allowing us to define expected behaviors and assertions without needing a real database. This technique drastically simplifies testing, particularly for unit tests, where testing isolated pieces of functionality is crucial.
Implementing DI in ASP.NET Core: A Step-by-Step Guide
Implementing DI in ASP.NET Core is straightforward, thanks to its built-in IoC container. Here’s a concise guide to get you started:
Define Interfaces for Your Services: As demonstrated with
IRepository<T>
, start by abstracting your services and repositories with interfaces. This abstraction is key to decoupling components.Register Dependencies in
Startup.cs
: Use theConfigureServices
method inStartup.cs
to register your services and their implementations with the IoC container.
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped<IRepository<Product>, ProductRepository>();
// Other service registrations
}
- Inject Dependencies Where Needed: Finally, add parameters to your classes' constructors for the services or repositories they need. The ASP.NET Core runtime handles the rest, injecting these dependencies when creating instances of your classes.
public class ProductsController : ControllerBase
{
private readonly IRepository<Product> _repository;
public ProductsController(IRepository<Product> repository)
{
_repository = repository;
}
// Action methods that use _repository
}
This streamlined approach not only makes your application more modular and testable but also aligns with modern software development practices, emphasizing maintainability and flexibility.
Conclusion: The Transformative Power of DI in ASP.NET
Incorporating Dependency Injection in ASP.NET applications is a transformative practice that significantly elevates code quality. By fostering loose coupling, DI facilitates a more modular application structure, making systems easier to understand, extend, or modify. Moreover, the enhancement of testability ensures that applications remain robust, with a lower risk of bugs, as changes can be rigorously tested in isolation.
Embracing DI not only aligns with best practices in software development but also positions ASP.NET applications for future growth and scalability. Whether you’re building web apps, APIs, or microservices, the principles and practices outlined here will serve as a cornerstone for developing high-quality, maintainable software solutions in the .NET ecosystem.
By integrating Dependency Injection into your ASP.NET projects, you embark on a path toward more resilient, adaptable, and testable applications, underscoring the importance of solid design principles in modern software development.