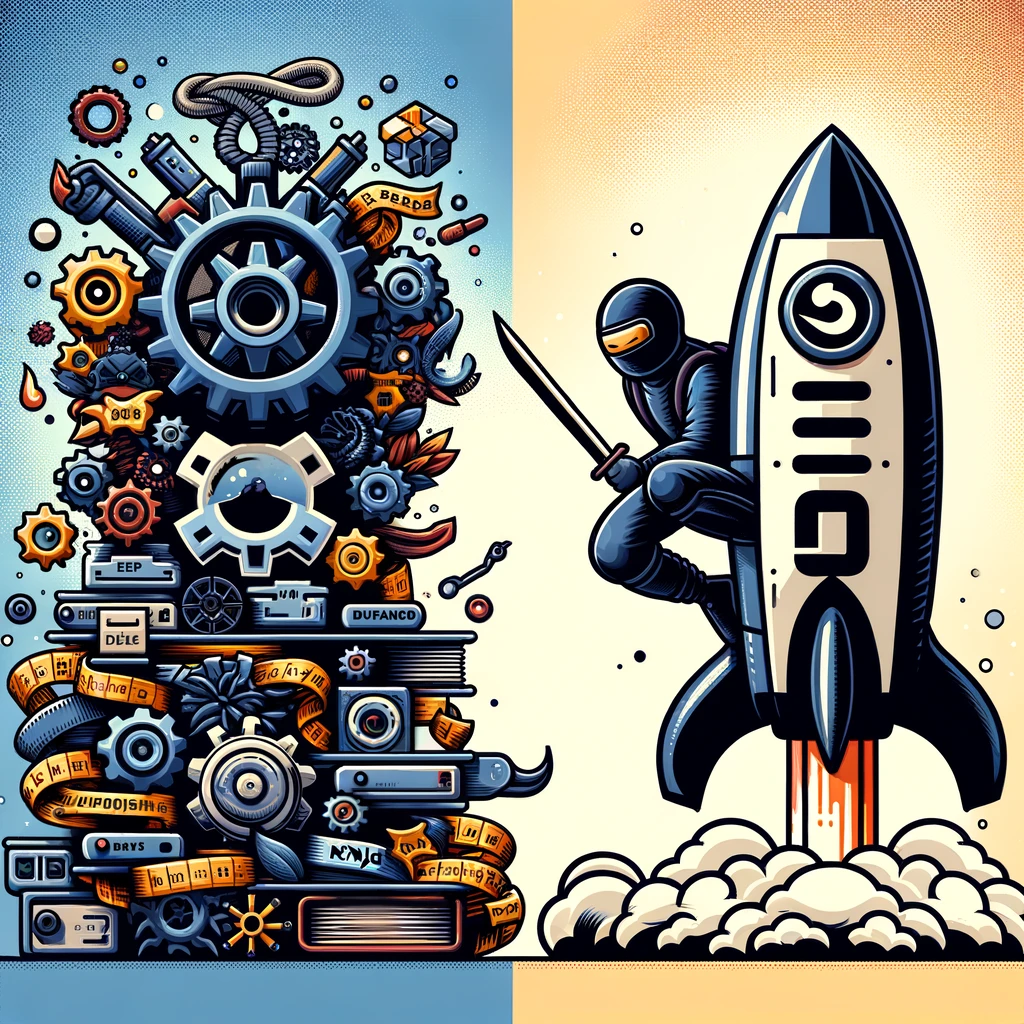
When developing web applications with Django, selecting the right toolkit for building APIs is crucial for both performance and productivity. Django Rest Framework (DRF) has been the go-to choice for many years, known for its comprehensive features and flexibility. However, Django-Ninja is a newer contender that promises faster API development with less boilerplate code. Let’s dive into the high-level differences between these two frameworks to help you make an informed decision for your next project.
Flexibility and Ease of Use
Django Rest Framework is celebrated for its flexibility and the depth of features it offers. It provides a powerful set of tools for web APIs, including serialization that supports ORM and non-ORM data sources, authentication policies, and comprehensive browsing and testing capabilities. DRF is designed to accommodate almost any API construction need, from simple CRUD operations to complex data processing. This flexibility, however, comes with a steeper learning curve and often requires more boilerplate code to get started.
# serializers.py
from rest_framework import serializers
from myapp.models import MyModel
class MyModelSerializer(serializers.ModelSerializer):
class Meta:
model = MyModel
fields = ['id', 'name', 'description']
# views.py
from rest_framework import viewsets
from .models import MyModel
from .serializers import MyModelSerializer
class MyModelViewSet(viewsets.ModelViewSet):
queryset = MyModel.objects.all()
serializer_class = MyModelSerializer
# urls.py
urlpatterns = [
path("api/my-model", MyModelViewSet.as_view()),
... # Repeated for each view set
]
Django-Ninja, on the other hand, focuses on speed and simplicity, leveraging Python type hints to reduce boilerplate and accelerate development. It’s heavily inspired by FastAPI, a framework known for its performance and ease of use in the async web world. Django-Ninja aims to bring similar benefits to Django, making API development faster and type-safe. For developers looking for quick iterations and those who prefer the modern feel of Python 3.6+ features, Django-Ninja offers an appealing approach.
# schemas.py
from pydantic import BaseModel
class MyModelSchema(BaseModel):
id: int
name: str
description: str
# urls.py
from django.urls import path
from ninja import NinjaAPI
from myapp.models import MyModel
from .schemas import MyModelSchema
api = NinjaAPI()
@api.get("/mymodels", response=list[MyModelSchema])
def list_models(request):
qs = MyModel.objects.all()
return qs
urlpatterns = [
path("api/", api.urls),
]
Performance
While Django Rest Framework provides a robust solution for API development, its comprehensive nature can sometimes lead to slower performance compared to more streamlined frameworks. It’s designed for versatility, not for speed, which is usually not a concern for most traditional web applications but can be a bottleneck for high-load environments.
Django-Ninja claims to offer better performance thanks to its lightweight nature and the use of pydantic for data parsing and validation. By emphasizing efficiency and reducing overhead, Django-Ninja can serve requests faster, making it a potentially better choice for performance-critical applications.
Learning Curve
The learning curve for Django Rest Framework can be steep for beginners. Its extensive documentation covers a wide range of functionalities, from serializers and viewsets to permissions and authentication classes. Mastering DRF takes time and patience, but it rewards developers with the ability to handle complex web API tasks.
Django-Ninja is designed to be more intuitive, especially for those familiar with modern Python features like type hints and async functions. Its simplicity and resemblance to FastAPI mean that developers can get up to speed quickly, making it an excellent choice for teams looking to minimize ramp-up time.
Community and Ecosystem
Django Rest Framework has a large and active community, which translates into a wealth of tutorials, third-party packages, and support resources. This ecosystem is invaluable for solving common problems and extending the framework’s capabilities.
While Django-Ninja is newer, its community is growing rapidly, driven by enthusiasm for its performance and ease of use. However, it doesn’t yet match DRF’s extensive ecosystem of plugins and extensions, which can be a consideration for projects requiring specific functionalities not covered by the core framework.
Conclusion
Choosing between Django Rest Framework and Django-Ninja depends largely on your project’s specific needs, your team’s familiarity with modern Python features, and performance requirements. DRF offers unparalleled flexibility and a rich set of features at the cost of a steeper learning curve and potentially slower performance. Django-Ninja, by contrast, prioritizes speed—both in terms of API response times and development velocity—with a more streamlined, type-safe approach.
For projects that require comprehensive API functionality and have a team experienced in Django, DRF may be the preferred choice. On the other hand, for new projects looking for rapid development and performance, or when experimenting with modern Python features, Django-Ninja could offer a compelling alternative.
Before making a decision, consider experimenting with both frameworks for a small prototype project. This hands-on experience can provide deeper insights into which framework best suits your project’s needs and team’s preferences.
Feature | Django Rest Framework (DRF) | Django-Ninja |
---|---|---|
Flexibility and Ease of Use | Offers comprehensive features but comes with more boilerplate code | Focuses on speed and simplicity, reduces boilerplate with Python type hints |
Performance | Comprehensive but may have slower performance due to extensive nature | Claims better performance due to lightweight design and efficient data parsing |
Learning Curve | Steeper learning curve due to extensive features and configurations | Designed to be more intuitive, especially for those familiar with modern Python features |
Community and Ecosystem | Large and active community with extensive resources and third-party packages | Growing community, may not have as extensive an ecosystem as DRF |
Links and references
- Official DRF Documentation: https://www.django-rest-framework.org/
- DRF Quickstart: https://www.django-rest-framework.org/tutorial/quickstart/
- Official Django-Ninja Documentation: https://django-ninja.dev/
- Getting Started with Django-Ninja: https://django-ninja.dev/tutorial/
- For a deeper dive into Python type hints which are extensively used in Django-Ninja: https://docs.python.org/3/library/typing.html