About the author
@catalinmpit is a software engineer, AWS community builder and technical writer based out of London. He’s currently an engineer at TypingDNA, working on applying keystroke dynamics as a means of biometrics authentication.
Check out more of his work on catalins.tech
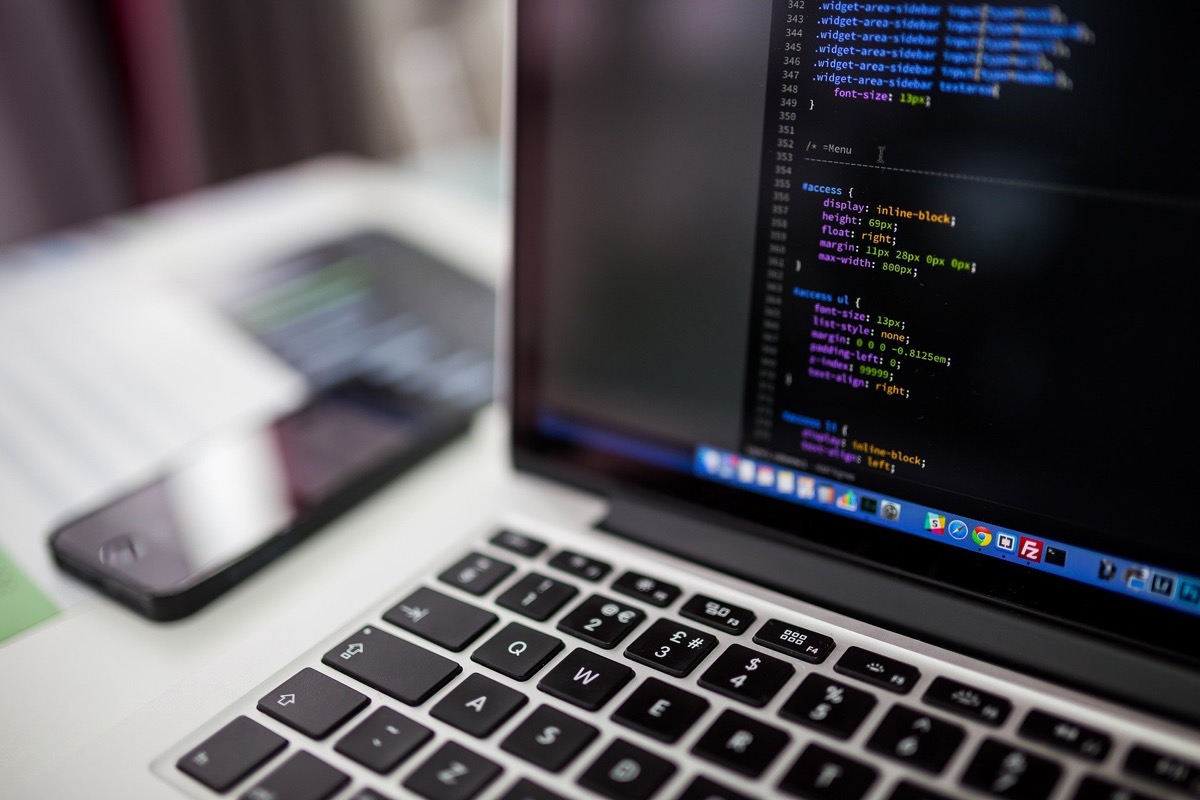
You’re probably familiar with console.log
, the most popular “debugging tool”. Although it is the most commonly used,
there are other popular Console methods, such as:
assert
warn
error
table
All these methods are useful and can make it easier to debug your applications. It’s important to note that they’re not the only console logging methods, but the ones I found to be the most useful.
Console Warn
Let’s talk first about the warn()
method. If we want to display a warning message in the console, we can use ‘warn
.’ When this method is used, the message is less likely to be missed because it is formatted differently.
Figure 1 illustrates a basic example of using console.warn()
. You can see that the message has a different color
and that it also has an exclamation mark at the beginning. So the warn()
method is handy when you want to
display a warning message in the console.
Console Table
This method allows us to display arrays or objects as a table. The method takes two arguments:
- The first one is the array/object you want to show, and it’s mandatory.
- The second argument specifies the name of the columns and is optional.
For example:
function Car(make, model) {
this.make = make;
this.model = model;
}
const audi = new Car("Audi", "A8");
const bmw = new Car("BMW", "X5");
const mercedes = new Car("Mercedes-Benz", "G-Class");
console.table([audi, bmw, mercedes]);
console.table([audi, bmw, mercedes], ['make']);
The first console.table
- console.table([audi, bmw, mercedes]);
- only specifies the array to be displayed as a
table. That means the columns are make
and model
by default. The columns are automatically taken from the “Car”
object. Thus, by running the code, the following will be printed to the console:
However, in the second console.table
- console.table([audi, bmw, mercedes], ['make'])
- we specify which columns to
display. In this case, limited to the make
column. Figure 3, below, illustrates the table with only the “make”
column.
Thus, if we do not pass the second argument (i.e. specify columns), console.table
uses the fields in the
object/array.
Console Assert
Console.assert()
sends an error to the console if the assertion is false, and does not return anything if the
assertion is true. Figure 4 shows an example where the assertion is false.
It is important to note that the execution of the code is not stopped when the assertion is false
. That means that
you cannot rely on it to test your code. However, the console assert
method can be handy as an alternative to
console.log
if you want to quickly debug your application or quickly/temporarily try certain cases.
In essence, console.assert
can be useful in many cases. Keep in mind, though, that it should be used in lieu of
proper application testing!
Console Error
The error
method is another method that is useful for debugging. What differentiates console warn
from console
log
is, as the name suggests, this console method allows us to output an error message.
How is it helpful, though? The main reason for this is that it outputs a red error message. Which will clearly
differentiate it from other debugging messages printed in the console. Figure 5 shows an example of console.error
in
action.
When you debug your application, because of its red background, you are less likely to miss the message. Use it appropriately, as with all other console methods! This shouldn’t be used as a substitute for proper error logging or testing of your application. However, it might help when debugging during development.
Conclusion
There are a lot more console methods than just console.log
which aren’t covered here, which you can find on Mozilla MDN.
These are the ones I use most and have found to be particularly helpful.
To recap:
console.warn()
allows you to output a warning to the console.console.table()
makes it easier to view the data in a tabular format.console.assert()
provides you with the ability to test specific scenarios.console.error()
allows the output of an error in the console.
Have you found a way to use console methods that you’ve found particularly useful? Let us know!
Catalin regularly posts helpful development tips and guides on Twitter. Be sure to follow him at @catalinmpit