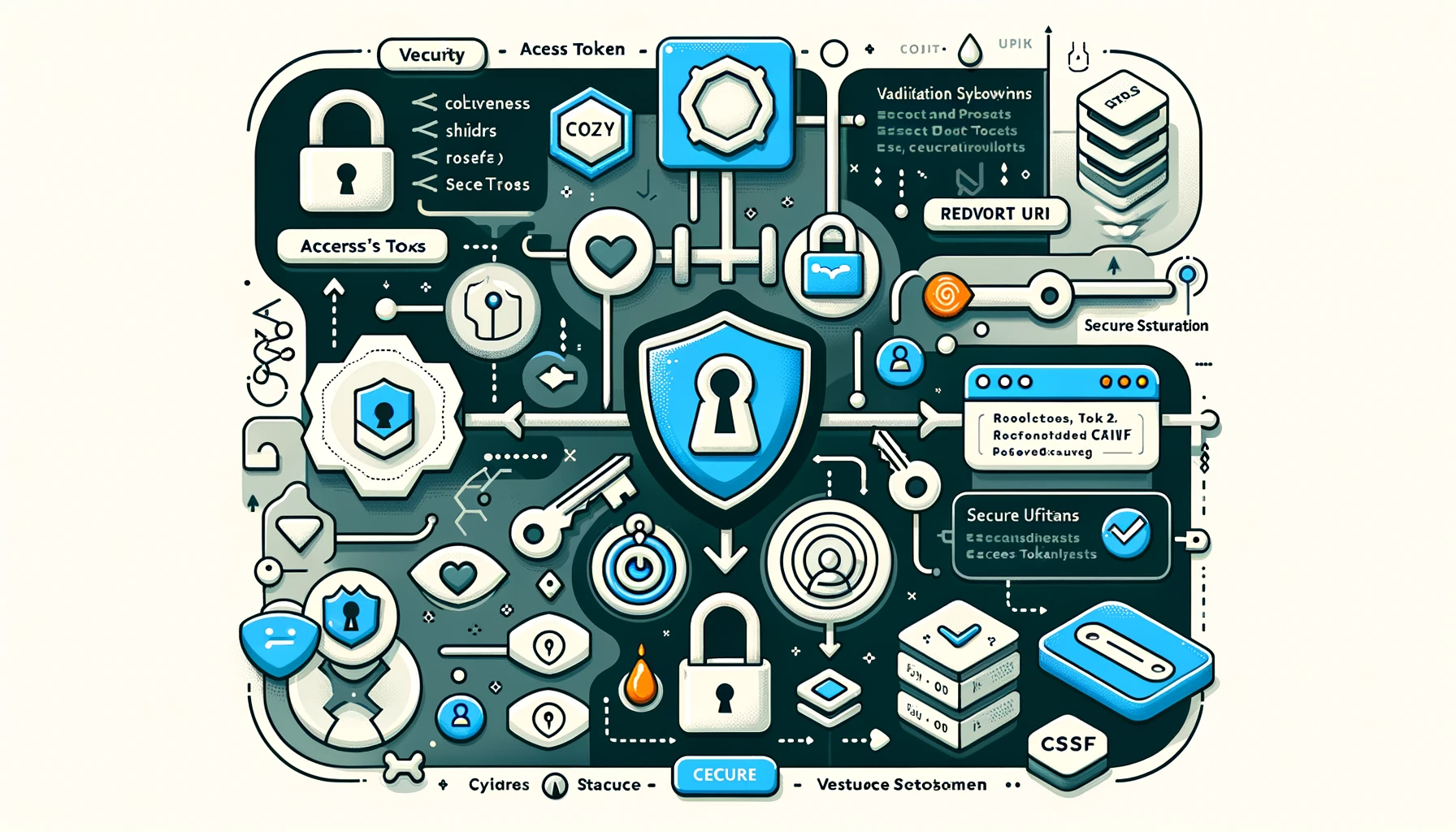
OAuth 2.0 has become the de facto standard for managing and accessing user permissions over the internet, allowing for seamless integration of third-party services without sharing password details. Despite its widespread adoption, the complexity of OAuth 2.0 implementations can lead to security vulnerabilities if not handled with care. In this post, we’ll explore some of the most common security issues associated with OAuth 2.0, alongside practical advice and code snippets to help you secure your implementations effectively.
Insecure Storage of Access Tokens
Access tokens are the lifeblood of OAuth 2.0, granting temporary access to user resources without exposing their credentials. However, improper storage of these tokens can lead to unauthorized access if attackers exploit vulnerabilities in your application.
Mitigation: For web applications, databases should always be on an internal network with tight access controls. In order to store OAuth access and refresh tokens, these fields should also be encrypted rather being stored in plain text. If these tokens are to be passed back to the user via a cookie, consider using HTTP-only and Secure cookies to store tokens, preventing them from being accessible via JavaScript and reducing the risk of cross-site scripting (XSS) attacks. Here’s an example of setting a secure cookie in an HTTP response:
from http.server import BaseHTTPRequestHandler
class SecureTokenHandler(BaseHTTPRequestHandler):
def set_secure_cookie(self, access_token):
self.send_response(200)
self.send_header('Set-Cookie', f'access_token={access_token}; HttpOnly; Secure; SameSite=Strict')
self.end_headers()
Redirect URI Manipulation
The redirect URI is a critical component in the OAuth 2.0 flow, determining where the authorization server sends the user after granting or denying access. Attackers can manipulate this URI to redirect users to malicious sites, potentially leading to phishing attacks or the theft of the authorization code.
Mitigation: Always validate redirect URIs against a whitelist of approved URLs on the server side. Avoid using wildcards or dynamic redirects that can be easily manipulated. Here’s a snippet demonstrating a simple redirect URI validation:
approved_redirect_uris = ["https://example.com/callback", "https://secure.example.com/oauth2/callback"]
def validate_redirect_uri(requested_uri):
if requested_uri in approved_redirect_uris:
return True
return False
Cross-Site Request Forgery (CSRF) in OAuth Flows
CSRF attacks can exploit the OAuth 2.0 authorization flow, tricking a logged-in user into executing actions without their knowledge or consent. This can be particularly harmful if the user has privileged access.
Mitigation: Use the “state” parameter effectively by generating a unique token for each authorization request and validating it upon the user’s return to the callback URL. This ensures that the request originated from your application. Here’s how you might implement this in Python:
import secrets
def generate_state_token():
return secrets.token_urlsafe(16)
def validate_state_token(returned_state, expected_state):
return secrets.compare_digest(returned_state, expected_state)
Insecure Direct Object References (IDOR) via Access Tokens
Insecure Direct Object References occur when an application allows direct access to objects based on user-supplied input. In the context of OAuth 2.0, attackers might use access tokens to gain unauthorized access to other users' resources.
Mitigation: Implement strict access control checks and token validation to ensure that the access token corresponds to the rightful resource owner. Here’s an example using a fictional API:
def get_user_data(access_token, user_id):
token_owner = validate_access_token(access_token)
if token_owner.id != user_id:
raise UnauthorizedAccessException
return retrieve_user_data(user_id)
Insufficient Scope Validation
OAuth 2.0 scopes limit what resources an access token can access. Insufficient scope validation can allow attackers to access unauthorized resources by exploiting overly permissive tokens.
Mitigation: Strictly enforce scope checks at your resource server. Ensure that each endpoint validates the token’s scope before fulfilling requests. Here’s a basic scope validation function:
def validate_token_scope(access_token, required_scope):
token_scopes = get_token_scopes(access_token)
return required_scope in token_scopes
Use of Implicit Grant Flow
The implicit grant flow was designed for clients that cannot securely store client secrets, such as single-page applications. However, it exposes tokens directly to the client, increasing the risk of token interception.
Mitigation: Prefer the authorization code flow with Proof Key for Code Exchange (PKCE) over the implicit flow, especially for single-page applications and mobile apps. PKCE adds an additional layer of security by dynamically generating a secret that is verified by the authorization server. Here’s how you might start an authorization request with PKCE in JavaScript:
const code_verifier = generateRandomString();
const code_challenge = base64UrlEncode(sha256(code_verifier));
const authorizationUrl = `https://auth.example.com/oauth/authorize?response_type=code&client_id=your_client_id&redirect_uri=${encodeURIComponent(redirectUri)}&code_challenge=${code_challenge}&code_challenge_method=S256`;
// Redirect user to authorizationUrl
Conclusion
Implementing OAuth 2.0 securely requires a deep understanding of its potential vulnerabilities and the adoption of best practices to mitigate these risks. By addressing common issues such as insecure token storage, redirect URI manipulation, CSRF attacks, IDOR, insufficient scope validation, and the risks associated with the implicit grant flow, developers can strengthen the security of their OAuth 2.0 implementations. Remember, security is an ongoing process, not a one-time setup. Regularly review and update your OAuth 2.0 implementations to protect against evolving threats.