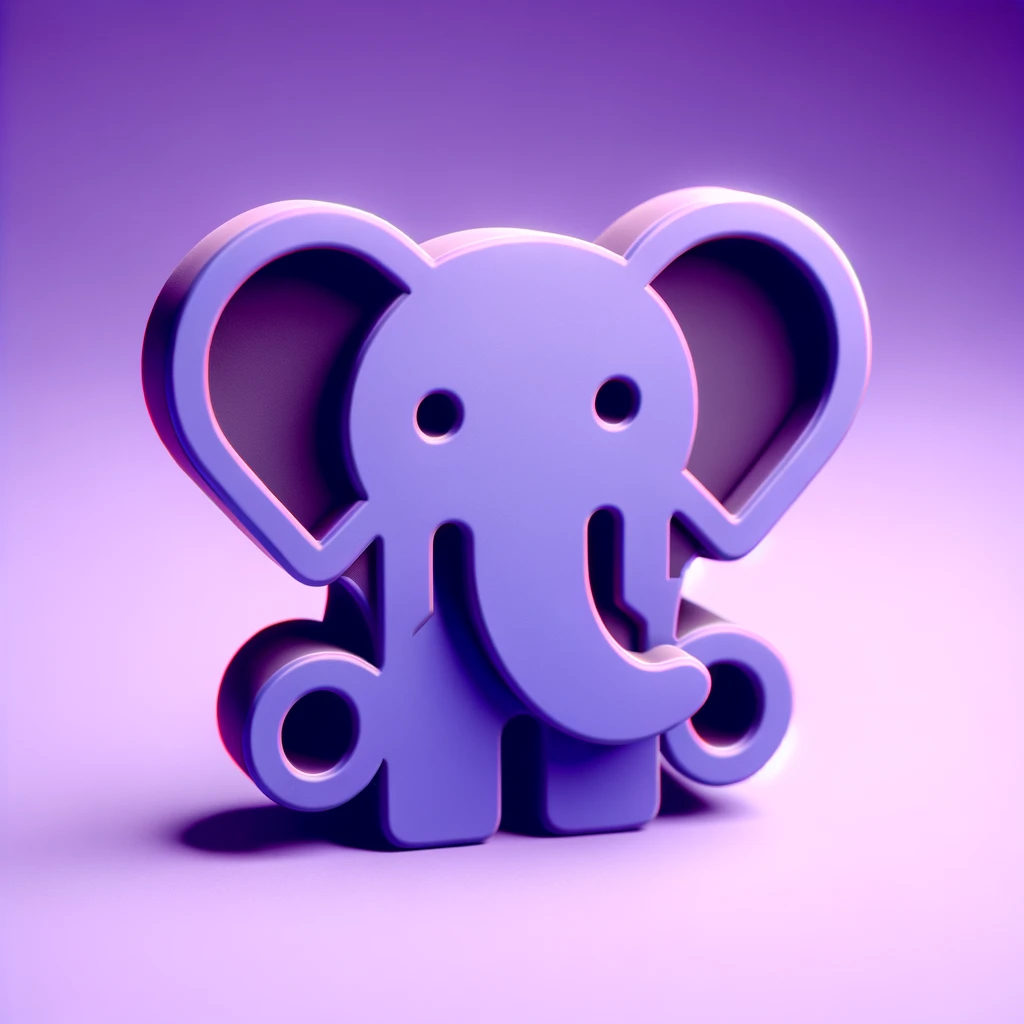
Understanding the nuances of the programming language you are using is crucial. Today, we’re diving deep into a common pitfall in PHP related to arrays and how they differ significantly from arrays in languages like Python and JavaScript. This insight is particularly important for developers who are transitioning between these languages or working in a multi-language environment.
PHP Arrays: More Than Meets the Eye
PHP, a popular general-purpose server-side interpreted languge, treats arrays as a primitive type. In PHP, when you reassign an array or other primative types, it uses copy-on-write semantics for the new variable. This means that if you write to one of the arrays after the assignment, it will create a copy an not mutate the previous array. This behavior can lead to unexpected outcomes if you are used to semantics in other popular interpreted server-side languages like Python and JavaScript that use references by default for arrays.
Example: The Copy-on-Write Mechanism
$originalArray = [1, 2, 3];
$copiedArray = $originalArray;
$copiedArray[0] = 10;
echo "Original: " . implode(', ', $originalArray) . "\n";
echo "Copied: " . implode(', ', $copiedArray) . "\n";
Original: 1, 2, 3
Copied: 10, 2, 3
In this snippet, altering $copiedArray
does not affect $originalArray
. This is because PHP employs a copy-on-write mechanism. When you assign $originalArray
to $copiedArray
, PHP doesn’t immediately copy the entire array. It only creates a new copy when you modify one of the arrays. This is efficient but can be confusing if you’re expecting reference-like behavior.
Closure scoping
This copy-on-write behavior also applies to closures. Consider the following example:
$originalArray = [1, 2, 3];
$closure = function () use ($originalArray) {
$originalArray[0] = 10;
echo implode(', ', $originalArray) . "\n";
};
$closure();
echo implode(', ', $originalArray) . "\n";
10, 2, 3
1, 2, 3
Here, the closure modifies $originalArray
, but the change doesn’t persist outside the closure. This is because the closure also captures a new variable with copy-on-write semantics of $originalArray
when it’s defined. This variable is then modified within the closure creating a copy, but the original array remains unchanged.
Passing by reference with the &
operator can be used to modify the original array:
$originalArray = [1, 2, 3];
$closure = function () use (&$originalArray) {
$originalArray[0] = 10;
};
$closure();
echo implode(', ', $originalArray) . "\n";
10, 2, 3
Contrast with Python and JavaScript
To appreciate the uniqueness of PHP’s approach to arrays, let’s compare it with Python and JavaScript.
Python: Lists as Objects
In Python, lists (the equivalent of arrays in PHP) are treated as objects. When you assign one list to another, both variables point to the same object.
original_list = [1, 2, 3]
copied_list = original_list
copied_list[0] = 10
print(f"Original: {original_list}")
print(f"Copied: {copied_list}")
Original: [10, 2, 3]
Copied: [10, 2, 3]
Here, changing copied_list
also alters original_list
. This reference-based approach is different from PHP’s copy-on-write strategy. It is worth noting that this behavior in Python does introduce its own set of common mistakes, such as mutable default arguments.
JavaScript: A Similar Story
JavaScript behaves similarly to Python in this context. Arrays in JavaScript are objects, and assigning an array to another variable doesn’t create a copy. Instead, it creates a reference to the original array.
let originalArray = [1, 2, 3];
let copiedArray = originalArray;
copiedArray[0] = 10;
console.log(`Original: ${originalArray}`);
console.log(`Copied: ${copiedArray}`);
Original: 10, 2, 3
Copied: 10, 2, 3
Again, modifying copiedArray
impacts originalArray
, showcasing the reference-based behavior typical in JavaScript.
Implications in Code Review
When reviewing PHP code, especially if your background is in Python or JavaScript, keep an eye out for array assignments. Ensure that developers understand the copy-on-write mechanics of PHP to prevent unintended side effects. This understanding is crucial for effective memory management and performance optimization.
Best Practices for PHP Developers
- Explicit Copying: When you need a true copy of an array in PHP, use functions like
array_slice()
with no arguments orarray_merge()
with a single array. - Reference Assignment: If you actually need reference behavior, use the
&
operator.$copiedArray = &$originalArray;
will link both variables to the same array. This can also be used in other contexts such as function arguments and closures. - Memory Management: Be aware of PHP’s memory usage with large arrays, especially when potentially duplicating them. If you reassign a large array and mutate it, you’ll end up with two copies in memory. This can lead to memory exhaustion and performance issues.
Conclusion
Understanding the subtle differences in array handling across PHP, Python, and JavaScript is essential for writing efficient and bug-free code. This knowledge is particularly vital during code reviews, where spotting these nuances can prevent performance issues and logical errors. As you transition between these languages, keep these differences in mind to leverage their respective strengths effectively.
PHP’s array handling, with its copy-on-write mechanism, offers a somewhat unique approach that, when understood and used correctly, can lead to clean and efficient code. Remember, the devil is in the details, and in the world of programming, these details make all the difference.